mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-06-05 23:22:06 +08:00
leetcode update
This commit is contained in:
parent
4b65aa9c40
commit
a073d1936f
@ -9533,3 +9533,380 @@ func luckyNumbers (matrix [][]int) []int {
|
||||
return result
|
||||
}
|
||||
```
|
||||
|
||||
## day145 2024-07-20
|
||||
### 1605. Find Valid Matrix Given Row and Column Sums
|
||||
You are given two arrays rowSum and colSum of non-negative integers where rowSum[i] is the sum of the elements in the ith row and colSum[j] is the sum of the elements of the jth column of a 2D matrix. In other words, you do not know the elements of the matrix, but you do know the sums of each row and column.
|
||||
|
||||
Find any matrix of non-negative integers of size rowSum.length x colSum.length that satisfies the rowSum and colSum requirements.
|
||||
|
||||
Return a 2D array representing any matrix that fulfills the requirements. It's guaranteed that at least one matrix that fulfills the requirements exists.
|
||||
|
||||
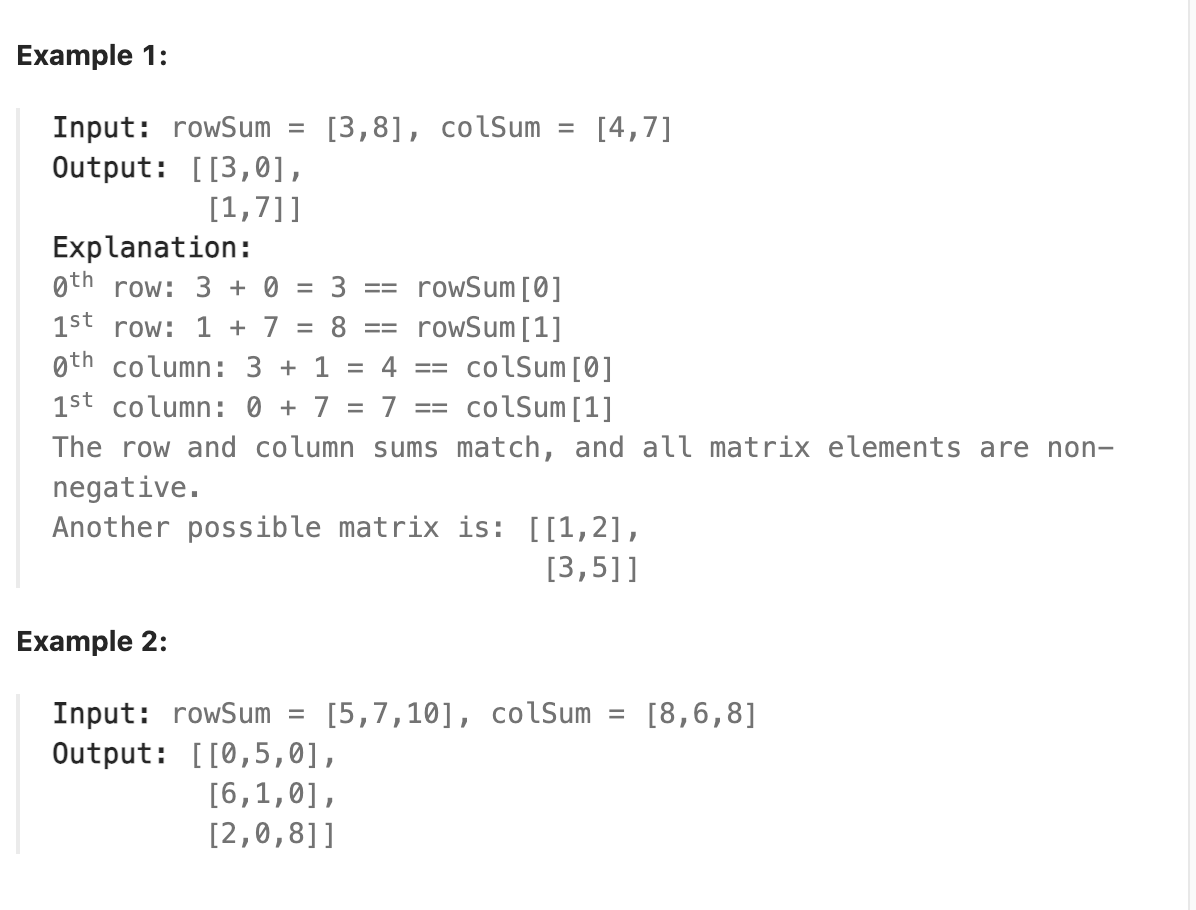
|
||||
|
||||
### 题解
|
||||
本题使用贪心算法, 在填充每一行的数字时, 每次都取当前位置行和和列和的较小值. 再分别从行和和列和中减去当前取的值. 继续填充下一个位置的数字. 这样可以保证填充的每个数字都不会使当前行和列超过和的限制(实际按这个思路只要取小于等于二者较小值即可), 直接取二者中的较小值可以保证二者中的一个变为0. 避免了后续继续填充时可能会因行或列的和的限制而无法取得合适的数的问题(0对和没有影响). 而当和为0时只需将该位置填充为0即可.
|
||||
|
||||
### 代码
|
||||
```go
|
||||
func restoreMatrix(rowSum []int, colSum []int) [][]int {
|
||||
result := [][]int{}
|
||||
collen := len(colSum)
|
||||
rowlen := len(rowSum)
|
||||
for i:=0;i<rowlen;i++{
|
||||
temprow := []int{}
|
||||
for j:=0;j<collen;j++{
|
||||
current := min(rowSum[i],colSum[j])
|
||||
temprow = append(temprow, current)
|
||||
rowSum[i] -= current
|
||||
colSum[j] -= current
|
||||
}
|
||||
result = append(result, temprow)
|
||||
}
|
||||
return result
|
||||
}
|
||||
```
|
||||
|
||||
## day146 2024-07-21
|
||||
### 2392. Build a Matrix With Conditions
|
||||
|
||||
You are given a positive integer k. You are also given:
|
||||
|
||||
a 2D integer array rowConditions of size n where rowConditions[i] = [abovei, belowi], and
|
||||
a 2D integer array colConditions of size m where colConditions[i] = [lefti, righti].
|
||||
The two arrays contain integers from 1 to k.
|
||||
|
||||
You have to build a k x k matrix that contains each of the numbers from 1 to k exactly once. The remaining cells should have the value 0.
|
||||
|
||||
The matrix should also satisfy the following conditions:
|
||||
|
||||
The number abovei should appear in a row that is strictly above the row at which the number belowi appears for all i from 0 to n - 1.
|
||||
The number lefti should appear in a column that is strictly left of the column at which the number righti appears for all i from 0 to m - 1.
|
||||
Return any matrix that satisfies the conditions. If no answer exists, return an empty matrix.
|
||||
|
||||
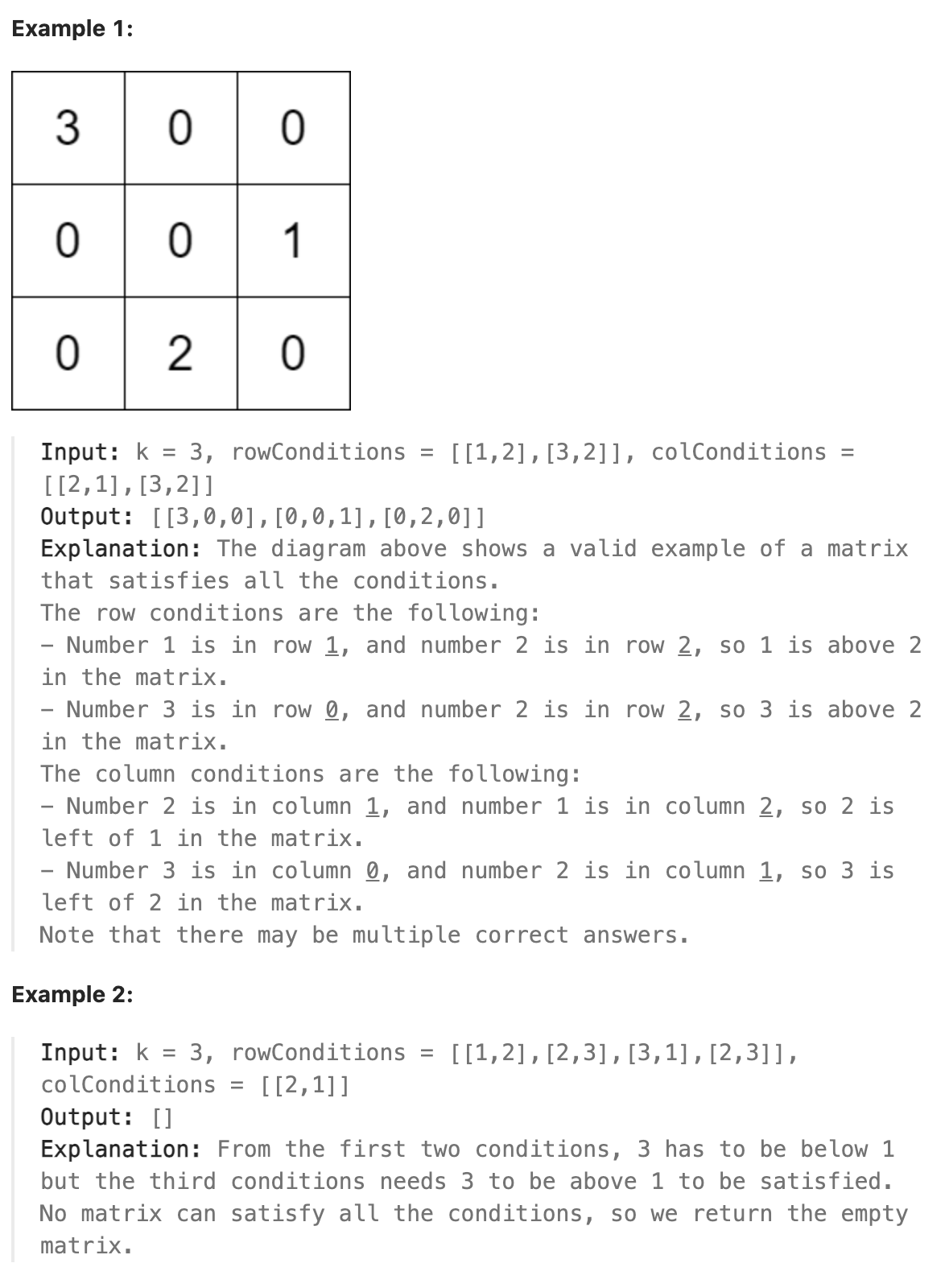
|
||||
|
||||
### 题解
|
||||
本题的条件实际上隐含了一种先后顺序, above在below的上面可以理解为above在below之前, 拓扑排序就是解决这种广义上的"顺序关系", 从a->b存在一种后继关系. 可以将above和below的关系视为有向图中的一条边, 从above指向below. 但本题中可能会出现环, 出现环即产生了冲突, 无法进行拓扑排序. 此时无解, 因此先判断由两个条件数组构建的有向图是否包含环, 包含环则无解, 不包含环继续进行拓扑排序, 得到拓扑排序后的数组, 按照数组中数对应的下标将其放入对应的行或者列. 注意对行进行拓扑排序得到的结果和对列进行拓扑排序得到的结果二者并不冲突. 所以分别排序并按顺序填入数组就能得到正确答案.
|
||||
|
||||
### 代码
|
||||
```go
|
||||
func buildMatrix(k int, rowConditions [][]int, colConditions [][]int) [][]int {
|
||||
// 定义检查环和拓扑排序的辅助函数
|
||||
hasCycle := func(graph [][]int, k int) bool {
|
||||
inDegree := make([]int, k+1)
|
||||
for _, edge := range graph {
|
||||
for _,value := range edge{
|
||||
inDegree[value]++
|
||||
}
|
||||
}
|
||||
|
||||
queue := []int{}
|
||||
for i := 1; i <= k; i++ {
|
||||
if inDegree[i] == 0 {
|
||||
queue = append(queue, i)
|
||||
}
|
||||
}
|
||||
|
||||
visited := 0
|
||||
for len(queue) > 0 {
|
||||
node := queue[0]
|
||||
queue = queue[1:]
|
||||
visited++
|
||||
for _, neighbor := range graph[node] {
|
||||
inDegree[neighbor]--
|
||||
if inDegree[neighbor] == 0 {
|
||||
queue = append(queue, neighbor)
|
||||
}
|
||||
}
|
||||
}
|
||||
return visited != k
|
||||
}
|
||||
|
||||
topologicalSort := func(graph [][]int, k int) []int {
|
||||
inDegree := make([]int, k+1)
|
||||
for _, edge := range graph {
|
||||
for _,value := range edge{
|
||||
inDegree[value]++
|
||||
}
|
||||
}
|
||||
|
||||
queue := []int{}
|
||||
for i := 1; i <= k; i++ {
|
||||
if inDegree[i] == 0 {
|
||||
queue = append(queue, i)
|
||||
}
|
||||
}
|
||||
|
||||
order := []int{}
|
||||
for len(queue) > 0 {
|
||||
node := queue[0]
|
||||
queue = queue[1:]
|
||||
order = append(order, node)
|
||||
for _, neighbor := range graph[node] {
|
||||
inDegree[neighbor]--
|
||||
if inDegree[neighbor] == 0 {
|
||||
queue = append(queue, neighbor)
|
||||
}
|
||||
}
|
||||
}
|
||||
if len(order) != k {
|
||||
return nil
|
||||
}
|
||||
return order
|
||||
}
|
||||
|
||||
// 构建行和列的有向图
|
||||
rowGraph := make([][]int, k+1)
|
||||
colGraph := make([][]int, k+1)
|
||||
for i := 0; i <= k; i++ {
|
||||
rowGraph[i] = []int{}
|
||||
colGraph[i] = []int{}
|
||||
}
|
||||
for _, cond := range rowConditions {
|
||||
rowGraph[cond[0]] = append(rowGraph[cond[0]], cond[1])
|
||||
}
|
||||
for _, cond := range colConditions {
|
||||
colGraph[cond[0]] = append(colGraph[cond[0]], cond[1])
|
||||
}
|
||||
|
||||
// 检查是否存在环
|
||||
if hasCycle(rowGraph, k) || hasCycle(colGraph, k) {
|
||||
return [][]int{}
|
||||
}
|
||||
|
||||
// 获取拓扑排序
|
||||
rowOrder := topologicalSort(rowGraph, k)
|
||||
colOrder := topologicalSort(colGraph, k)
|
||||
if rowOrder == nil || colOrder == nil {
|
||||
return [][]int{}
|
||||
}
|
||||
|
||||
// 构建结果矩阵
|
||||
matrix := make([][]int, k)
|
||||
for i := range matrix {
|
||||
matrix[i] = make([]int, k)
|
||||
}
|
||||
|
||||
rowPos := make(map[int]int)
|
||||
colPos := make(map[int]int)
|
||||
for i, num := range rowOrder {
|
||||
rowPos[num] = i
|
||||
}
|
||||
for i, num := range colOrder {
|
||||
colPos[num] = i
|
||||
}
|
||||
|
||||
for num := 1; num <= k; num++ {
|
||||
matrix[rowPos[num]][colPos[num]] = num
|
||||
}
|
||||
|
||||
return matrix
|
||||
}
|
||||
```
|
||||
|
||||
## day147 2024-07-22
|
||||
### 2418. Sort the People
|
||||
You are given an array of strings names, and an array heights that consists of distinct positive integers. Both arrays are of length n.
|
||||
|
||||
For each index i, names[i] and heights[i] denote the name and height of the ith person.
|
||||
|
||||
Return names sorted in descending order by the people's heights.
|
||||
|
||||
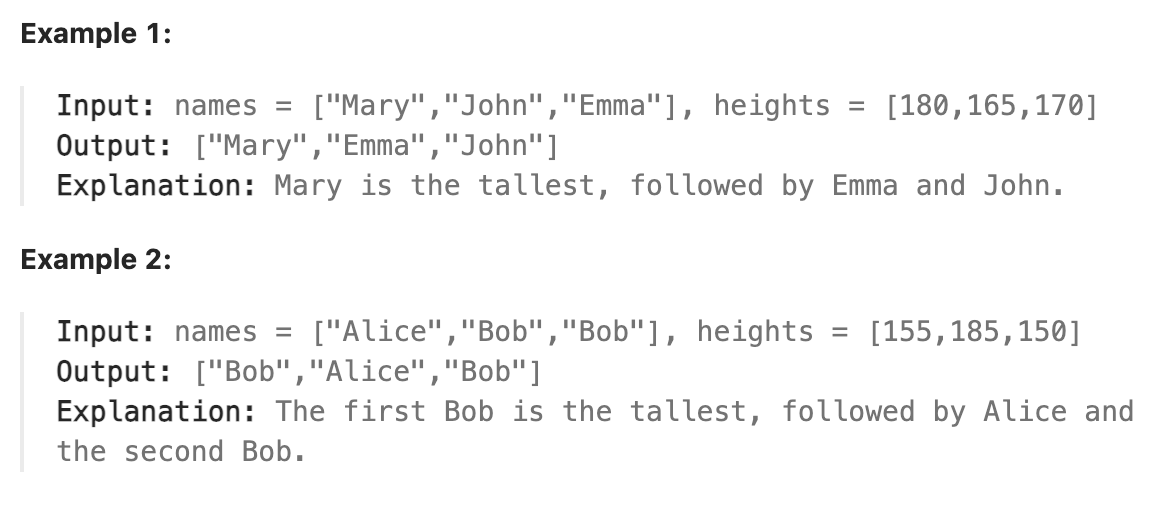
|
||||
|
||||
### 题解
|
||||
本题先将人名和身高绑定成一个结构体, 再根据身高排序, 思路是比较简单, 因此手动实现快排用于身高排序.
|
||||
|
||||
### 代码
|
||||
```go
|
||||
func sortPeople(names []string, heights []int) []string {
|
||||
type people struct{
|
||||
name string
|
||||
height int
|
||||
}
|
||||
peoples := []people{}
|
||||
for i,name := range names{
|
||||
peoples = append(peoples, people{name, heights[i]})
|
||||
}
|
||||
var quicksort func(sortpeople []people)
|
||||
quicksort = func(sortpeople []people){
|
||||
if len(sortpeople) <= 1{
|
||||
return
|
||||
}
|
||||
flag := sortpeople[0]
|
||||
i := 1
|
||||
j := len(sortpeople)-1
|
||||
for i<j{
|
||||
if sortpeople[i].height < flag.height{
|
||||
for sortpeople[j].height < flag.height && j>i{
|
||||
j--
|
||||
}
|
||||
sortpeople[i],sortpeople[j] = sortpeople[j],sortpeople[i]
|
||||
}
|
||||
i++
|
||||
}
|
||||
if sortpeople[j].height > sortpeople[0].height{
|
||||
sortpeople[0],sortpeople[j] = sortpeople[j],sortpeople[0]
|
||||
}
|
||||
quicksort(sortpeople[0:j])
|
||||
quicksort(sortpeople[j:])
|
||||
return
|
||||
}
|
||||
quicksort(peoples)
|
||||
result := []string{}
|
||||
for _,value := range peoples{
|
||||
result = append(result, value.name)
|
||||
}
|
||||
return result
|
||||
}
|
||||
```
|
||||
|
||||
## day148 2024-07-23
|
||||
### 1636. Sort Array by Increasing Frequency
|
||||
Given an array of integers nums, sort the array in increasing order based on the frequency of the values. If multiple values have the same frequency, sort them in decreasing order.
|
||||
|
||||
Return the sorted array.
|
||||
|
||||
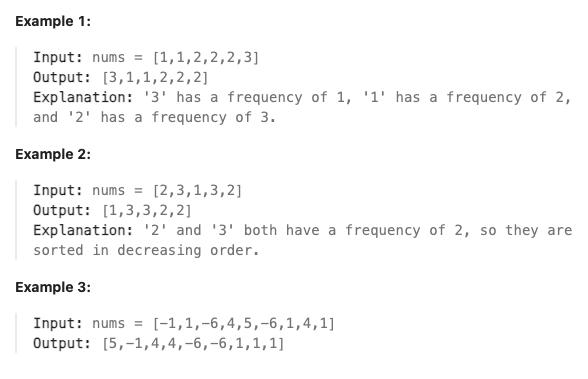
|
||||
|
||||
### 题解
|
||||
本题可使用计数排序, 先使用map统计各个数字出现的次数, 再直接对原始数组进行排序, 首先根据出现的次数排序, 如果两个数字出现次数相同则根据数字大小排序, 将数字更大的放在前面, 这里只需根据上面的条件写好go内置的sort函数即可完成排序. 最后返回结果.
|
||||
|
||||
### 代码
|
||||
```go
|
||||
func frequencySort(nums []int) []int {
|
||||
mapp:= make(map[int]int)
|
||||
n:= len(nums)
|
||||
if n==1{
|
||||
return nums
|
||||
}
|
||||
for i:=0;i<n;i++{
|
||||
mapp[nums[i]]++
|
||||
}
|
||||
sort.Slice(nums, func(i, j int)bool{
|
||||
if mapp[nums[i]]==mapp[nums[j]]{
|
||||
return nums[i]>nums[j]
|
||||
}
|
||||
return mapp[nums[i]]<mapp[nums[j]]
|
||||
})
|
||||
return nums
|
||||
}
|
||||
```
|
||||
|
||||
## day149 2024-07-24
|
||||
### 2191. Sort the Jumbled Numbers
|
||||
You are given a 0-indexed integer array mapping which represents the mapping rule of a shuffled decimal system. mapping[i] = j means digit i should be mapped to digit j in this system.
|
||||
|
||||
The mapped value of an integer is the new integer obtained by replacing each occurrence of digit i in the integer with mapping[i] for all 0 <= i <= 9.
|
||||
|
||||
You are also given another integer array nums. Return the array nums sorted in non-decreasing order based on the mapped values of its elements.
|
||||
|
||||
Notes:
|
||||
|
||||
Elements with the same mapped values should appear in the same relative order as in the input.
|
||||
The elements of nums should only be sorted based on their mapped values and not be replaced by them.
|
||||
|
||||
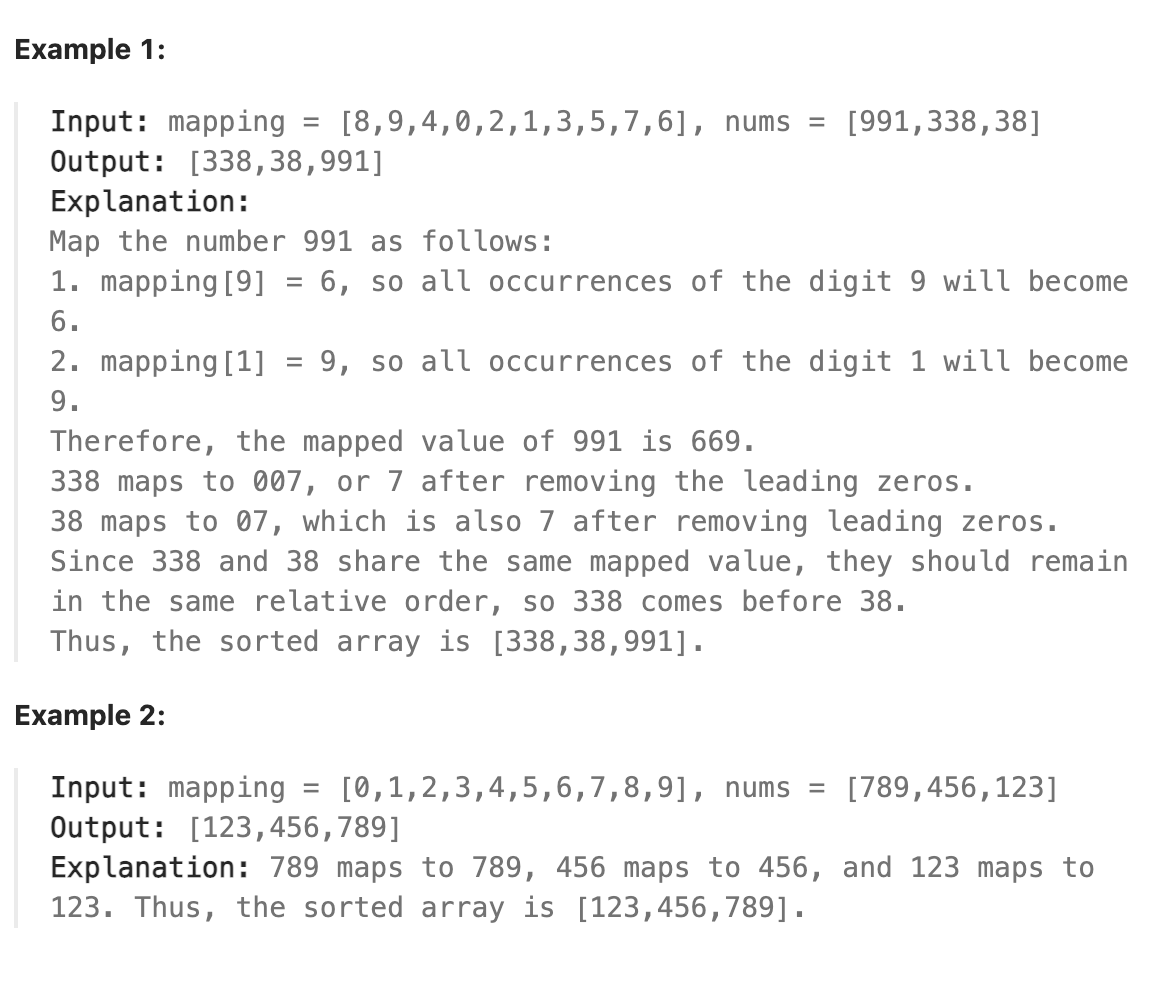
|
||||
|
||||
### 题解
|
||||
本题要解决两个问题, 一是将原始数字映射为其对应的映射数字, 二是将映射数字排序并按序排列对应的原始数字并返回. 将原始数字映射为对应数字只需每次除以10, 按位处理即可, 注意单独处理0的情况. 对映射后数字排序并反映到原来的数组上需要注意体重明确说明了对于映射数字相同的数字需保持原始数组的顺序, 因此需要稳定排序, 在go中使用sort.SliceStable即可进行稳定排序.
|
||||
|
||||
### 代码
|
||||
```go
|
||||
func sortJumbled(mapping []int, nums []int) []int {
|
||||
type mapNum struct{
|
||||
raw int
|
||||
mapped int
|
||||
}
|
||||
mapNums := []mapNum{}
|
||||
for _,value := range nums{
|
||||
mapNums = append(mapNums, mapNum{value, toMap(value, mapping)})
|
||||
}
|
||||
sort.SliceStable(mapNums, func(i,j int)bool{
|
||||
return mapNums[i].mapped < mapNums[j].mapped
|
||||
})
|
||||
result := []int{}
|
||||
for _,num := range mapNums{
|
||||
result = append(result, num.raw)
|
||||
}
|
||||
return result
|
||||
|
||||
}
|
||||
|
||||
func toMap(raw int, mapping []int)int{
|
||||
if raw == 0{
|
||||
return mapping[0]
|
||||
}else{
|
||||
result := 0
|
||||
i := 1
|
||||
remain := 0
|
||||
quote := 0
|
||||
for raw != 0{
|
||||
remain = raw % 10
|
||||
quote = raw / 10
|
||||
raw = quote
|
||||
result += mapping[remain] * i
|
||||
i *= 10
|
||||
}
|
||||
return result
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
## day150 2024-07-25
|
||||
### 912. Sort an Array
|
||||
Given an array of integers nums, sort the array in ascending order and return it.
|
||||
|
||||
You must solve the problem without using any built-in functions in O(nlog(n)) time complexity and with the smallest space complexity possible.
|
||||
|
||||
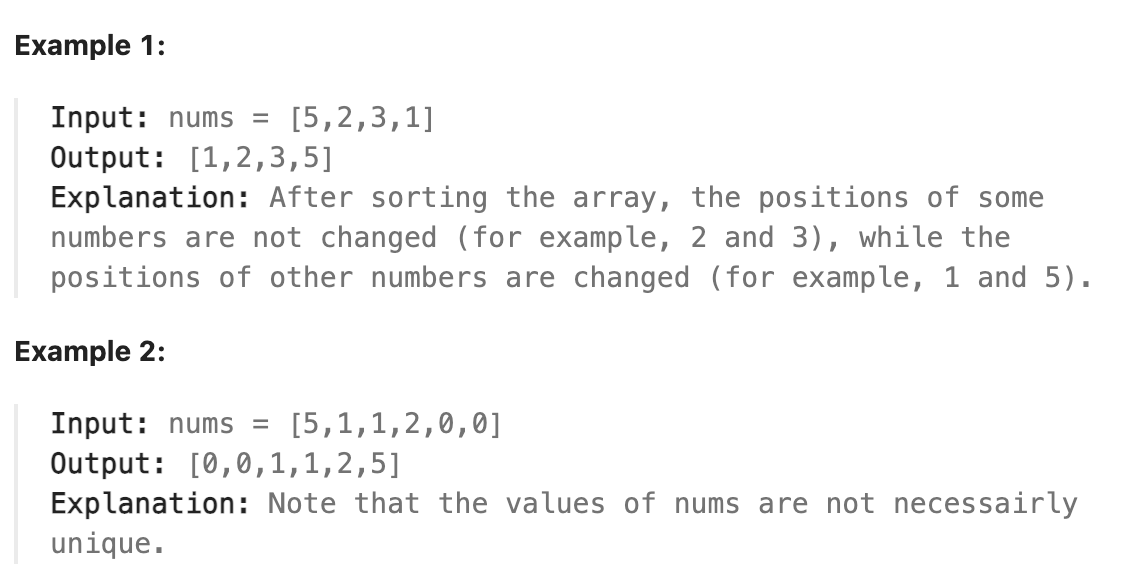
|
||||
|
||||
### 题解
|
||||
本题是一道基础题, 要求手动实现一种排序方式进行排序, 之前已经实现过归并排序, 快排, 计数排序, 因此本次实现堆排序, 实现堆排序的关键在于构建一个最小堆.
|
||||
|
||||
### 代码
|
||||
```go
|
||||
|
||||
type IntHeap []int
|
||||
|
||||
func (h IntHeap) Len() int {
|
||||
return len(h)
|
||||
}
|
||||
|
||||
func (h IntHeap) Less(i, j int) bool {
|
||||
return h[i] < h[j]
|
||||
}
|
||||
|
||||
func (h IntHeap) Swap(i, j int) {
|
||||
h[i], h[j] = h[j], h[i]
|
||||
}
|
||||
|
||||
func (h *IntHeap) Push(x interface{}) {
|
||||
*h = append(*h, x.(int))
|
||||
}
|
||||
|
||||
func (h *IntHeap) Pop() interface{} {
|
||||
old := *h
|
||||
n := len(old)
|
||||
x := old[n-1]
|
||||
*h = old[0 : n-1]
|
||||
return x
|
||||
}
|
||||
|
||||
func sortArray(nums []int) []int {
|
||||
h := &IntHeap{}
|
||||
heap.Init(h) // 初始化堆
|
||||
|
||||
// 将所有元素推入堆中
|
||||
for _, num := range nums {
|
||||
heap.Push(h, num)
|
||||
}
|
||||
|
||||
sortedArray := make([]int, 0, len(nums))
|
||||
for h.Len() > 0 {
|
||||
sortedArray = append(sortedArray, heap.Pop(h).(int))
|
||||
}
|
||||
return sortedArray
|
||||
}
|
||||
```
|
||||
|
Loading…
Reference in New Issue
Block a user