mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-07-05 22:13:46 +08:00
leetcode update
This commit is contained in:
@ -7393,3 +7393,96 @@ func minIncrementForUnique(nums []int) int {
|
||||
return result
|
||||
}
|
||||
```
|
||||
|
||||
## day110 2024-06-15
|
||||
|
||||
### 502. IPO
|
||||
|
||||
Suppose LeetCode will start its IPO soon. In order to sell a good price of its shares to Venture Capital, LeetCode would like to work on some projects to increase its capital before the IPO. Since it has limited resources, it can only finish at most k distinct projects before the IPO. Help LeetCode design the best way to maximize its total capital after finishing at most k distinct projects.
|
||||
|
||||
You are given n projects where the ith project has a pure profit profits[i] and a minimum capital of capital[i] is needed to start it.
|
||||
|
||||
Initially, you have w capital. When you finish a project, you will obtain its pure profit and the profit will be added to your total capital.
|
||||
|
||||
Pick a list of at most k distinct projects from given projects to maximize your final capital, and return the final maximized capital.
|
||||
|
||||
The answer is guaranteed to fit in a 32-bit signed integer.
|
||||
|
||||
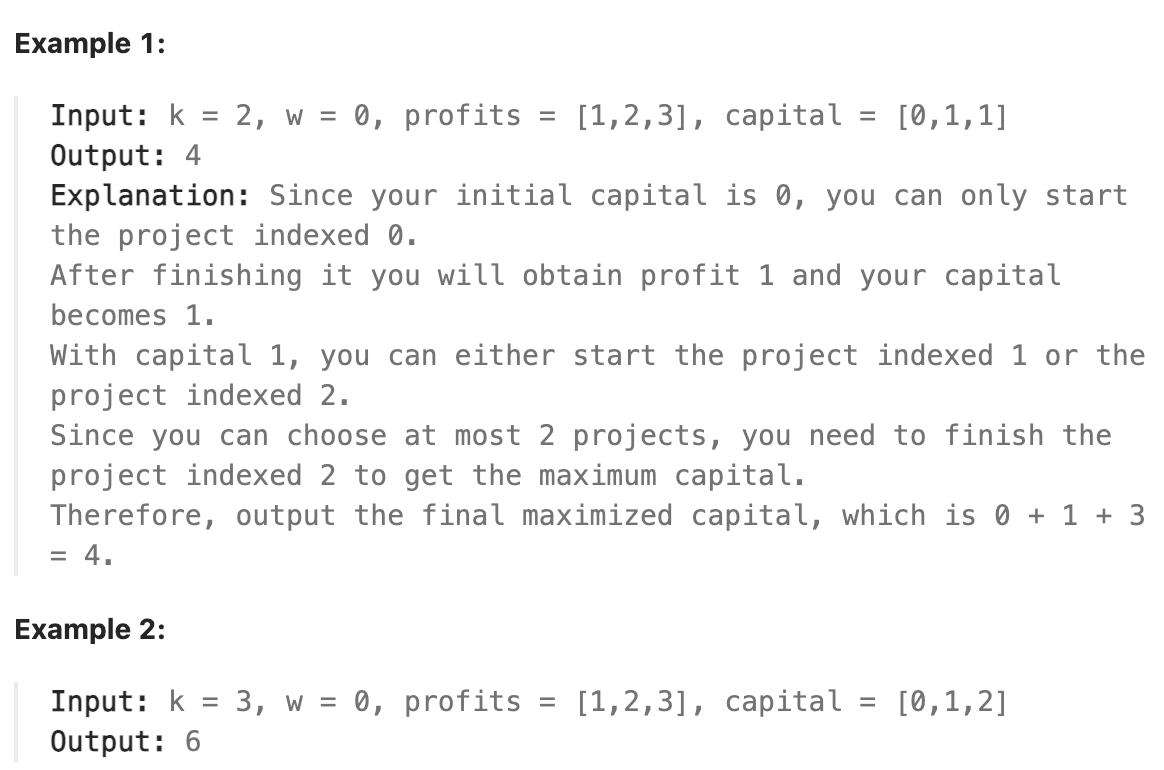
|
||||
|
||||
### 题解
|
||||
|
||||
本题是一道难题, 思路总体还是比较清晰的, 本题目标是取k个不同的project, 使得最后能得到的利润最大. 每一个project都有对应的利润和成本. 要使最后的整体利润最大, 无疑只要在当前可以选择(即成本在当前已获得的资金范围内)的所有project中选择利润最大的, 再更新可选择的project, 再次选择利润最大的. 如此反复直到选择完全部的k个project即可. 有了这个思路, 我们要解决两个问题. 1. 如何高效的选择利润最大的project, 最大堆显然是一个不错的选择. 2. 如何更新可选择的project的范围, 我们将project的成本排序, 每次获得了更多的本金后就将小于当前本金的所有project的利润加入最大堆, 为了每次只放入新增加的project, 可以用一个标记变量标记之前已经选择过的project位置. 获得更多本金后从标记位置开始继续遍历数组, 并将成本小于本金的项的利润放入最大堆中. 代码实现以上解题思路即可.
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
type ProHeap []int
|
||||
|
||||
func (h ProHeap) Len()int {
|
||||
return len(h)
|
||||
}
|
||||
|
||||
func (h ProHeap) Less(i,j int)bool {
|
||||
return h[i] > h[j]
|
||||
}
|
||||
|
||||
func (h *ProHeap) Swap(i, j int) {
|
||||
(*h)[i], (*h)[j] = (*h)[j], (*h)[i]
|
||||
}
|
||||
|
||||
func (h *ProHeap) Push(x interface{}) {
|
||||
*h = append(*h, x.(int))
|
||||
}
|
||||
|
||||
func (h *ProHeap) Pop() interface{}{
|
||||
res := (*h)[len(*h)-1]
|
||||
*h = (*h)[:len(*h)-1]
|
||||
return res
|
||||
}
|
||||
|
||||
type Project struct {
|
||||
capital int
|
||||
profit int
|
||||
}
|
||||
|
||||
func findMaximizedCapital(k int, w int, profits []int, capital []int) int {
|
||||
n := len(profits)
|
||||
projects := make([]Project, n)
|
||||
|
||||
for i := 0; i < n; i++ {
|
||||
projects[i] = Project{capital: capital[i], profit: profits[i]}
|
||||
}
|
||||
|
||||
sort.Slice(projects, func(i, j int) bool {
|
||||
return projects[i].capital < projects[j].capital
|
||||
})
|
||||
|
||||
|
||||
|
||||
proheap := make(ProHeap, 0)
|
||||
heap.Init(&proheap)
|
||||
|
||||
mark := 0
|
||||
for k>0{
|
||||
for _,value := range projects[mark:n]{
|
||||
if value.capital > w{
|
||||
break
|
||||
}else{
|
||||
heap.Push(&proheap, projects[mark].profit)
|
||||
mark++
|
||||
}
|
||||
}
|
||||
if proheap.Len() == 0{
|
||||
return w
|
||||
}
|
||||
w += heap.Pop(&proheap).(int)
|
||||
k--
|
||||
}
|
||||
return w
|
||||
}
|
||||
```
|
||||
|
||||
### 总结
|
||||
|
||||
go中最大堆可以使用容器类heap来实现, 只要实现了heap接口的所有方法, 就可以直接使用这个堆了.
|
||||
|
Reference in New Issue
Block a user