mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-06-07 20:02:08 +08:00
leetcode update
This commit is contained in:
parent
410ff80ba7
commit
fab75c4b92
@ -5801,3 +5801,127 @@ func validpath(grid [][]int,rowlen int,mid int)bool{
|
|||||||
return false
|
return false
|
||||||
}
|
}
|
||||||
```
|
```
|
||||||
|
|
||||||
|
## day 79 2024-05-16
|
||||||
|
|
||||||
|
### 2331. Evaluate Boolean Binary Tree
|
||||||
|
|
||||||
|
You are given the root of a full binary tree with the following properties:
|
||||||
|
|
||||||
|
Leaf nodes have either the value 0 or 1, where 0 represents False and 1 represents True.
|
||||||
|
Non-leaf nodes have either the value 2 or 3, where 2 represents the boolean OR and 3 represents the boolean AND.
|
||||||
|
The evaluation of a node is as follows:
|
||||||
|
|
||||||
|
If the node is a leaf node, the evaluation is the value of the node, i.e. True or False.
|
||||||
|
Otherwise, evaluate the node's two children and apply the boolean operation of its value with the children's evaluations.
|
||||||
|
Return the boolean result of evaluating the root node.
|
||||||
|
|
||||||
|
A full binary tree is a binary tree where each node has either 0 or 2 children.
|
||||||
|
|
||||||
|
A leaf node is a node that has zero children.
|
||||||
|
|
||||||
|
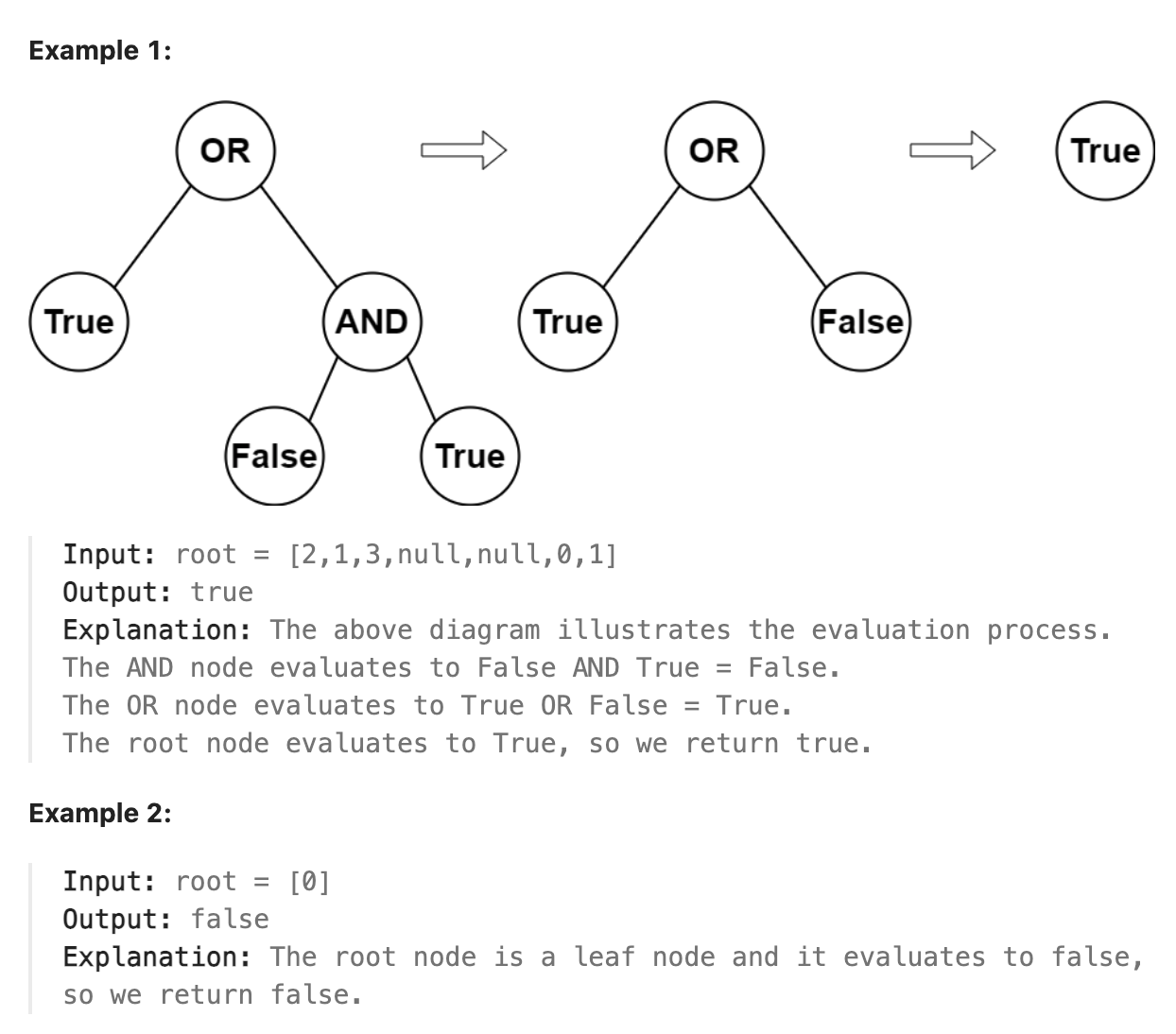
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
后续遍历计算各个节点的布尔值最终得到根节点的布尔值即可, 考查二叉树的后序遍历, 可以使用递归实现.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
/**
|
||||||
|
* Definition for a binary tree node.
|
||||||
|
* type TreeNode struct {
|
||||||
|
* Val int
|
||||||
|
* Left *TreeNode
|
||||||
|
* Right *TreeNode
|
||||||
|
* }
|
||||||
|
*/
|
||||||
|
func evaluateTree(root *TreeNode) bool {
|
||||||
|
result := caculatebool(root)
|
||||||
|
return result
|
||||||
|
}
|
||||||
|
|
||||||
|
func caculatebool(father *TreeNode)bool{
|
||||||
|
if father.Left == nil{
|
||||||
|
return father.Val == 1
|
||||||
|
}else {
|
||||||
|
if father.Val == 2{
|
||||||
|
return caculatebool(father.Left) || caculatebool(father.Right)
|
||||||
|
}else if father.Val == 3{
|
||||||
|
return caculatebool(father.Left) && caculatebool(father.Right)
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return false
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
## day80 2024-05-17
|
||||||
|
|
||||||
|
### 1325. Delete Leaves With a Given Value
|
||||||
|
|
||||||
|
Given a binary tree root and an integer target, delete all the leaf nodes with value target.
|
||||||
|
|
||||||
|
Note that once you delete a leaf node with value target, if its parent node becomes a leaf node and has the value target, it should also be deleted (you need to continue doing that until you cannot).
|
||||||
|
|
||||||
|
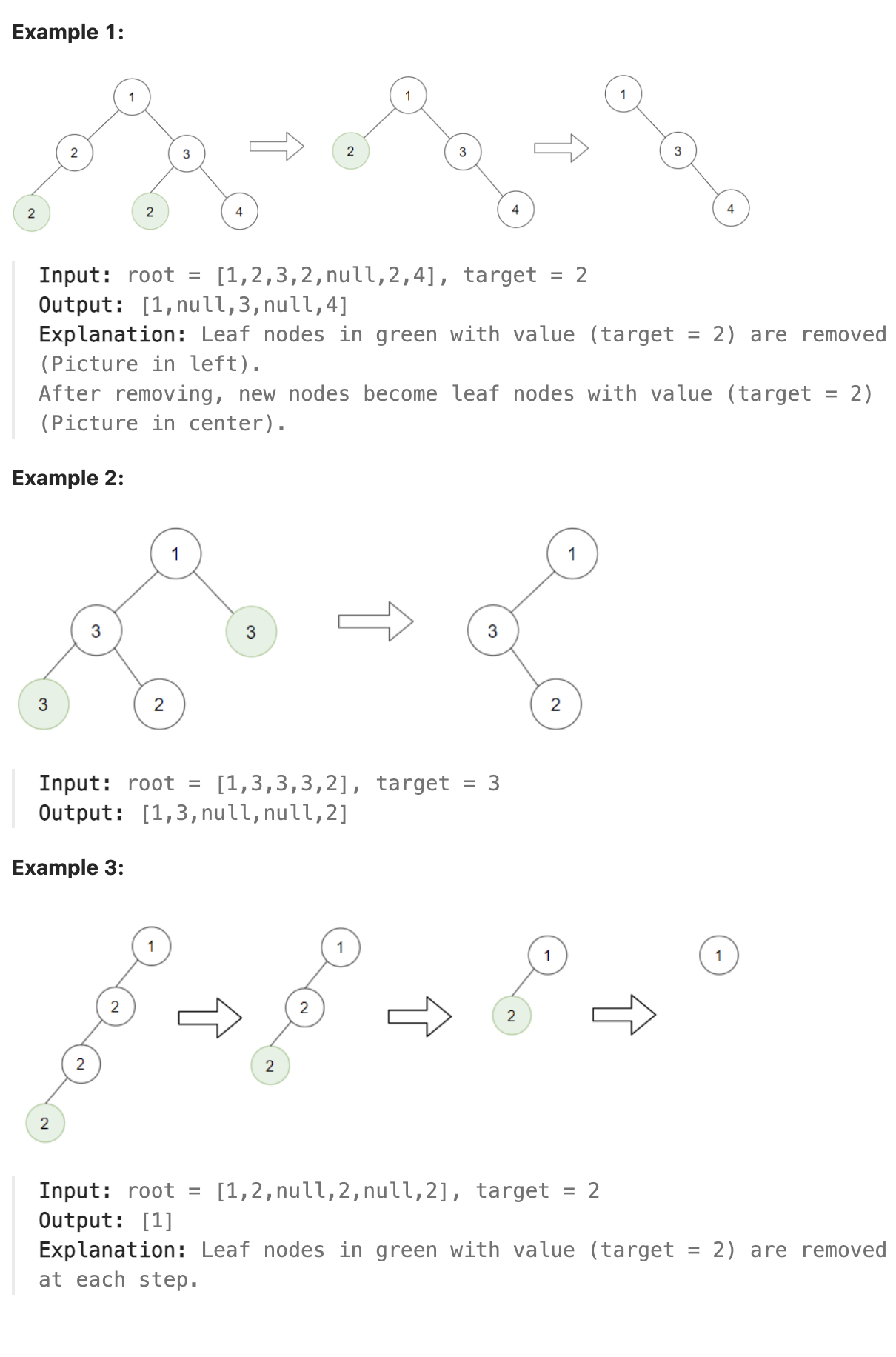
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
后序遍历即可, 使用递归遍历过程中返回当前节点是否被删除, 需要被删除返回true, 否则返回false. 父节点对子节点进行函数调用, 根据子节点的情况决定是否判断父节点是否要被删除. 这里相当于将子节点及子节点以下的信息向上传递.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
/**
|
||||||
|
* Definition for a binary tree node.
|
||||||
|
* type TreeNode struct {
|
||||||
|
* Val int
|
||||||
|
* Left *TreeNode
|
||||||
|
* Right *TreeNode
|
||||||
|
* }
|
||||||
|
*/
|
||||||
|
func removeLeafNodes(root *TreeNode, target int) *TreeNode {
|
||||||
|
if root == nil{
|
||||||
|
return nil
|
||||||
|
}
|
||||||
|
result := deleteaNode(root, target)
|
||||||
|
if result{
|
||||||
|
return nil
|
||||||
|
}else{
|
||||||
|
return root
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
func deleteaNode(father *TreeNode, target int)bool{
|
||||||
|
deleted := true
|
||||||
|
judge := false
|
||||||
|
if father.Left != nil {
|
||||||
|
judge = deleteaNode(father.Left, target)
|
||||||
|
if judge{
|
||||||
|
father.Left = nil
|
||||||
|
}
|
||||||
|
deleted = deleted && judge
|
||||||
|
|
||||||
|
}
|
||||||
|
if father.Right != nil{
|
||||||
|
judge = deleteaNode(father.Right, target)
|
||||||
|
if judge{
|
||||||
|
father.Right = nil
|
||||||
|
}
|
||||||
|
deleted = deleted && deleteaNode(father.Right, target)
|
||||||
|
}
|
||||||
|
if deleted{
|
||||||
|
if father.Val == target{
|
||||||
|
return true
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
return false
|
||||||
|
}
|
||||||
|
|
||||||
|
```
|
||||||
|
|
||||||
|
### 总结
|
||||||
|
|
||||||
|
直接返回节点会更简洁一些, 对于值符合的叶子节点, 递归时返回nil即可.
|
||||||
|
Loading…
Reference in New Issue
Block a user