mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-07-12 09:43:46 +08:00
leetcode update
This commit is contained in:
@ -17092,25 +17092,30 @@ public:
|
|||||||
}
|
}
|
||||||
};
|
};
|
||||||
```
|
```
|
||||||
|
|
||||||
## day243 2024-11-06
|
## day243 2024-11-06
|
||||||
### 3011. Find if Array Can Be Sorted
|
|
||||||
|
### 3011. Find if Array Can Be Sorted
|
||||||
|
|
||||||
You are given a 0-indexed array of positive integers nums.
|
You are given a 0-indexed array of positive integers nums.
|
||||||
|
|
||||||
In one operation, you can swap any two adjacent elements if they have the same number of
|
In one operation, you can swap any two adjacent elements if they have the same number of
|
||||||
set bits
|
set bits
|
||||||
. You are allowed to do this operation any number of times (including zero).
|
. You are allowed to do this operation any number of times (including zero).
|
||||||
|
|
||||||
Return true if you can sort the array, else return false.
|
Return true if you can sort the array, else return false.
|
||||||
|
|
||||||
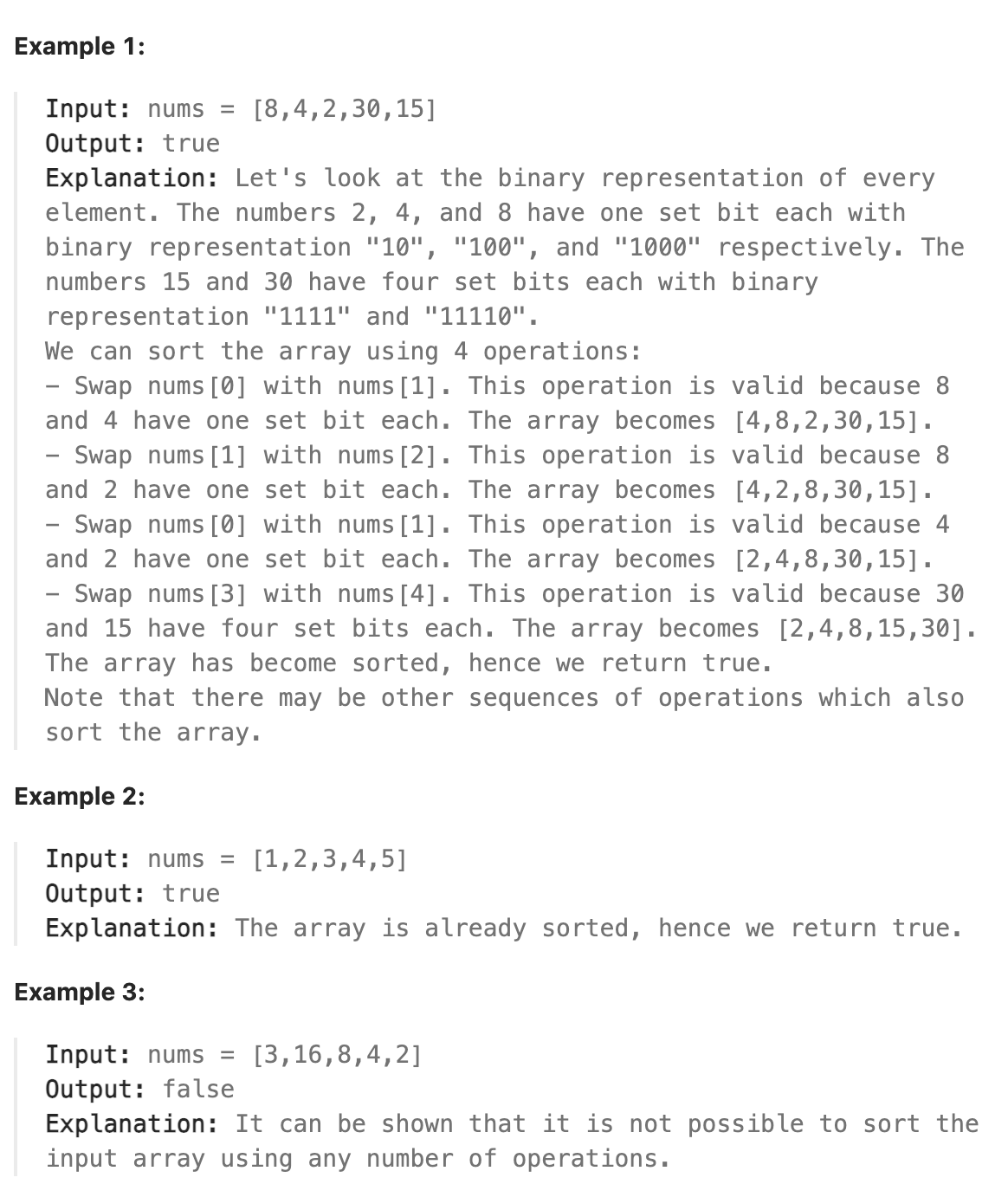
|
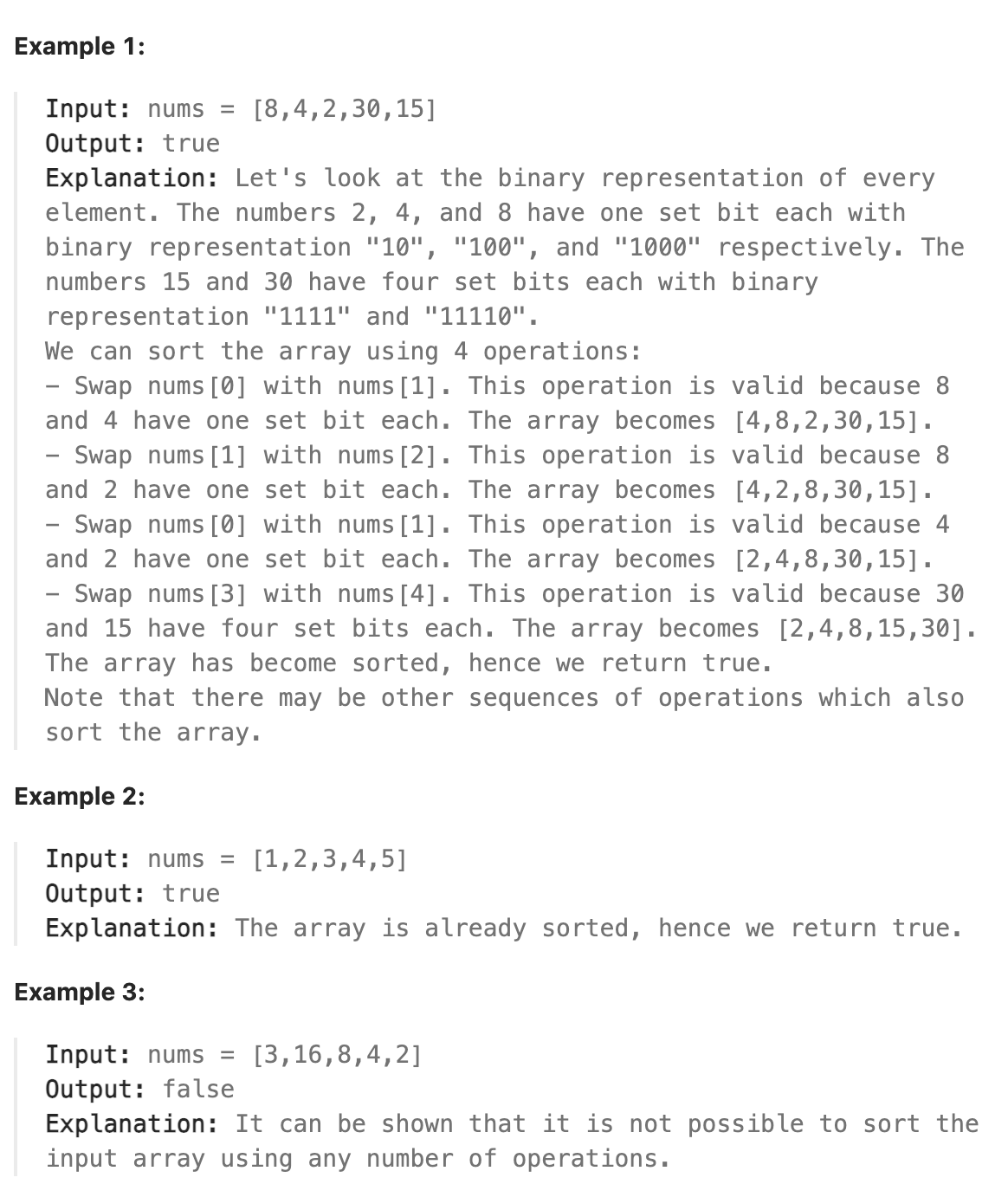
|
||||||
|
|
||||||
### 题解
|
### 题解
|
||||||
|
|
||||||
本题要求从小到大排列数组,对于包含个数相同的1的连续数字,这些数字之间总可以通过不断的相邻交换最终从小到大排列。因此我们不必关心这些数字具体的排序方式,只需记录连续数字中值最大的那个,当遇到新的一组包含不同个数1的数字时只需该组数字中最小值大于前面组数字的最大值数组整体即可完成排序,若小于最大值则由于该数字包含的1的个数和前面的数字不同,该数字不可能与前面的数字交换位置,因此该数字不可能交换到数组的前面,此时就无法成功排序。
|
本题要求从小到大排列数组,对于包含个数相同的1的连续数字,这些数字之间总可以通过不断的相邻交换最终从小到大排列。因此我们不必关心这些数字具体的排序方式,只需记录连续数字中值最大的那个,当遇到新的一组包含不同个数1的数字时只需该组数字中最小值大于前面组数字的最大值数组整体即可完成排序,若小于最大值则由于该数字包含的1的个数和前面的数字不同,该数字不可能与前面的数字交换位置,因此该数字不可能交换到数组的前面,此时就无法成功排序。
|
||||||
|
|
||||||
统计某个数字n中包含的二进制1的个数,可以使用n&(n-1),n&(n-1)每次可以消掉一个尾部所有0之前的第一个二进制1,通过不断的进行n&(n-1)的变换,记录变换的次数,当n为0时变换的次数即为该数字中包含的1的个数。
|
统计某个数字n中包含的二进制1的个数,可以使用n&(n-1),n&(n-1)每次可以消掉一个尾部所有0之前的第一个二进制1,通过不断的进行n&(n-1)的变换,记录变换的次数,当n为0时变换的次数即为该数字中包含的1的个数。
|
||||||
|
|
||||||
### 代码
|
### 代码
|
||||||
```cpp
|
|
||||||
|
```cpp
|
||||||
class Solution {
|
class Solution {
|
||||||
public:
|
public:
|
||||||
bool canSortArray(vector<int>& nums) {
|
bool canSortArray(vector<int>& nums) {
|
||||||
@ -17151,3 +17156,92 @@ public:
|
|||||||
}
|
}
|
||||||
};
|
};
|
||||||
```
|
```
|
||||||
|
|
||||||
|
## day244 2024-11-07
|
||||||
|
|
||||||
|
### 2275. Largest Combination With Bitwise AND Greater Than Zero
|
||||||
|
|
||||||
|
The bitwise AND of an array nums is the bitwise AND of all integers in nums.
|
||||||
|
|
||||||
|
For example, for nums = [1, 5, 3], the bitwise AND is equal to 1 & 5 & 3 = 1.
|
||||||
|
Also, for nums = [7], the bitwise AND is 7.
|
||||||
|
You are given an array of positive integers candidates. Evaluate the bitwise AND of every combination of numbers of candidates. Each number in candidates may only be used once in each combination.
|
||||||
|
|
||||||
|
Return the size of the largest combination of candidates with a bitwise AND greater than 0.
|
||||||
|
|
||||||
|
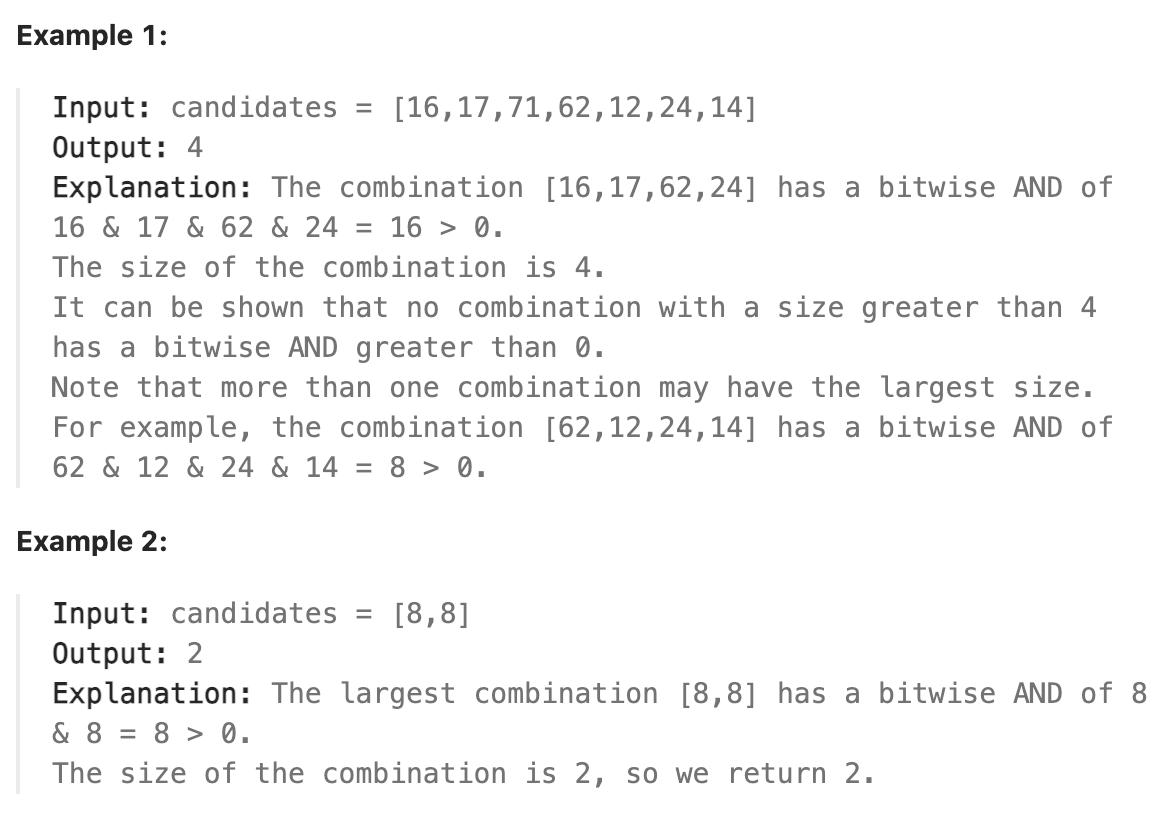
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题要得到最长的按位与和大于0的数字组合的长度。这种涉及位运算的题目就要从二进制的角度来看,按位与的特点是参与运算的数字中只要有一个在第n位上为0,最终得到的结果在该位上就为0。因此要保证最终得到的结果不为0,至少要保证所有参与运算的数字在某一个相同的二进制位上均为1,则可构造一个数组表示某个二进制位上为1的数字个数。遍历candidates,对每个遍历的数字,将其所有为1的二进制位对应的数组中的数字加1,最终即可得到全部二进制位对应的为1的数字个数,取其中的最大值即得结果。
|
||||||
|
|
||||||
|
注意本题给定条件candidates中数字不大于10^7,用24位二进制位即可表示,可以构建一个长度位25的数组来表示25个不同二进制位上为1的数字个数。
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```cpp
|
||||||
|
class Solution {
|
||||||
|
public:
|
||||||
|
int largestCombination(vector<int>& candidates) {
|
||||||
|
vector<int> count(25,0);
|
||||||
|
for (int can : candidates){
|
||||||
|
int i = 1;
|
||||||
|
while(can > 0){
|
||||||
|
if((can & 1) == 1){
|
||||||
|
count[i]++;
|
||||||
|
}
|
||||||
|
can = can >> 1;
|
||||||
|
i++;
|
||||||
|
}
|
||||||
|
}
|
||||||
|
int max = 0;
|
||||||
|
for (int co : count){
|
||||||
|
if (co > max){
|
||||||
|
max = co;
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return max;
|
||||||
|
}
|
||||||
|
};
|
||||||
|
```
|
||||||
|
|
||||||
|
## day245 2024-11-08
|
||||||
|
|
||||||
|
### 1829. Maximum XOR for Each Query
|
||||||
|
|
||||||
|
You are given a sorted array nums of n non-negative integers and an integer maximumBit. You want to perform the following query n times:
|
||||||
|
|
||||||
|
Find a non-negative integer k < 2maximumBit such that nums[0] XOR nums[1] XOR ... XOR nums[nums.length-1] XOR k is maximized. k is the answer to the ith query.
|
||||||
|
Remove the last element from the current array nums.
|
||||||
|
Return an array answer, where answer[i] is the answer to the ith query.
|
||||||
|
|
||||||
|
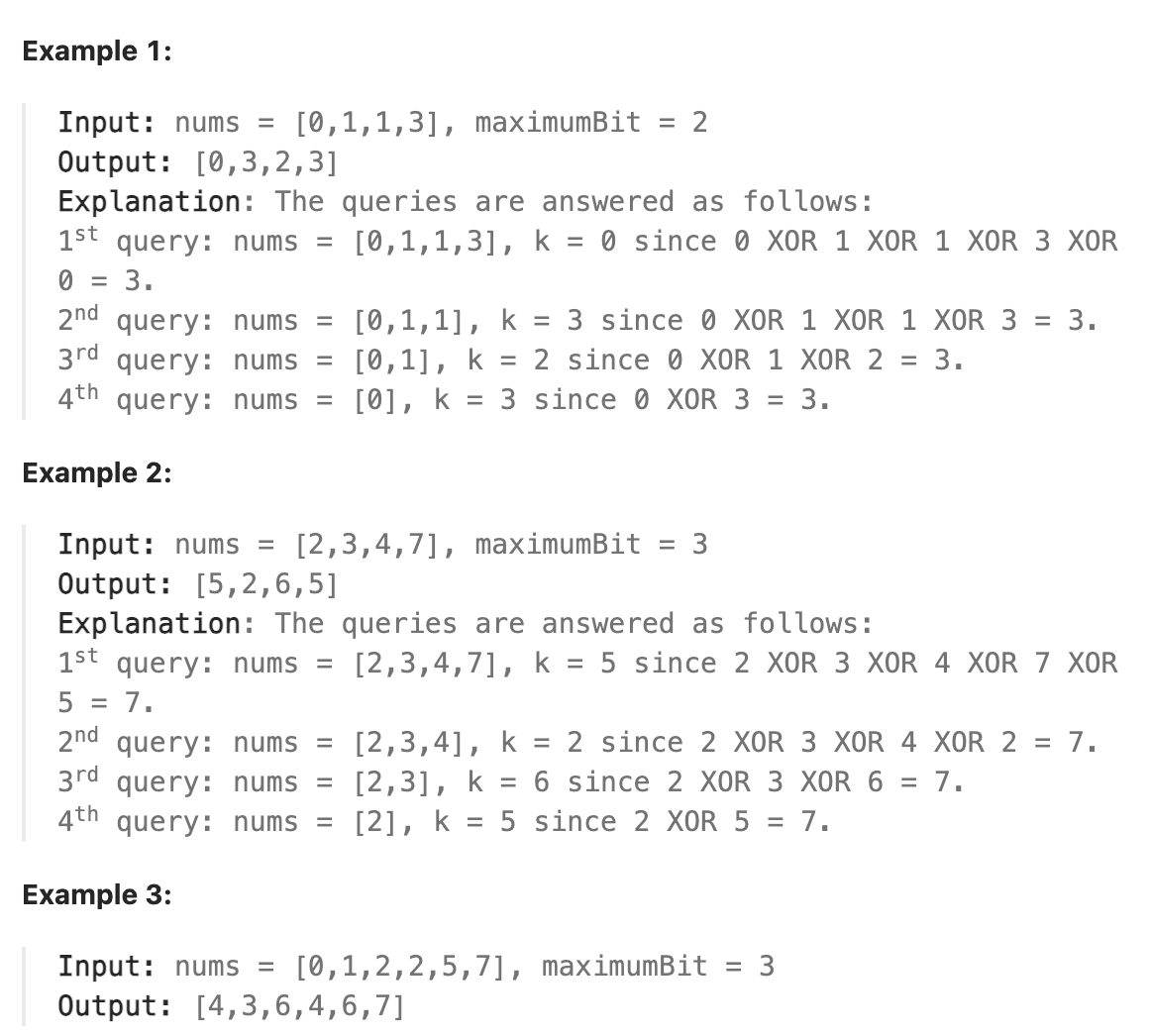
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题仍然从二进制的角度来看,对于整个数组的异或和,若要再与一个有限位数(maximumBit)的二进制数异或后得到最大值,显然要使最终结果中的maximumBit均为1。根据异或的特性,假如当前数字是一个三位数x,在与一个数字y异或后得到三位二进制数的最大值111(7),则由x^y=111可知x^111=y,可以将其称为异或的“恢复性”,即能根据结果和其中一个参与运算的值恢复出另一个参与运算的值。
|
||||||
|
|
||||||
|
则本题同理,将数组异或的结果和maximumBit位数的最大值异或,即可得到使maximumBit位数最大的数,但注意数组异或的结果位数可能比maximumBit位数多,此时在和maximumBit最大值异或后应该取maximumBit位(可以通过与maximumBit位全1二进制数做按位与得到)作为最终的结果k。前面的位数因为k取不到故默认为0(可以保留这些位上的原始的数组异或值)。
|
||||||
|
|
||||||
|
每次算出一个k后,数组要删掉末尾的数字,此时不必再重新从头计算一遍数组的异或和,只需将以前的异或和与删掉的数字做异或即得剩余数字的异或和。
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```cpp
|
||||||
|
class Solution {
|
||||||
|
public:
|
||||||
|
vector<int> getMaximumXor(vector<int>& nums, int maximumBit) {
|
||||||
|
int max = (1LL << maximumBit) - 1;
|
||||||
|
int nxor = 0;
|
||||||
|
for (int num : nums){
|
||||||
|
nxor ^= num;
|
||||||
|
}
|
||||||
|
vector<int> result;
|
||||||
|
for (int i=nums.size()-1;i>=0;i--){
|
||||||
|
result.push_back(nxor ^ max & max);
|
||||||
|
nxor ^= nums[i];
|
||||||
|
}
|
||||||
|
return result;
|
||||||
|
}
|
||||||
|
};
|
||||||
|
```
|
||||||
|
Reference in New Issue
Block a user