mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-04-20 14:02:07 +08:00
leetcode update
This commit is contained in:
parent
28eb47a664
commit
f0d7c6a4e0
@ -650,3 +650,46 @@ func hasCycle(head *ListNode) bool {
|
|||||||
return false
|
return false
|
||||||
}
|
}
|
||||||
```
|
```
|
||||||
|
|
||||||
|
## day10 2024-03-07
|
||||||
|
|
||||||
|
### 876. Middle of the Linked List
|
||||||
|
|
||||||
|
Given the head of a singly linked list, return the middle node of the linked list.
|
||||||
|
|
||||||
|
If there are two middle nodes, return the second middle node.
|
||||||
|
|
||||||
|
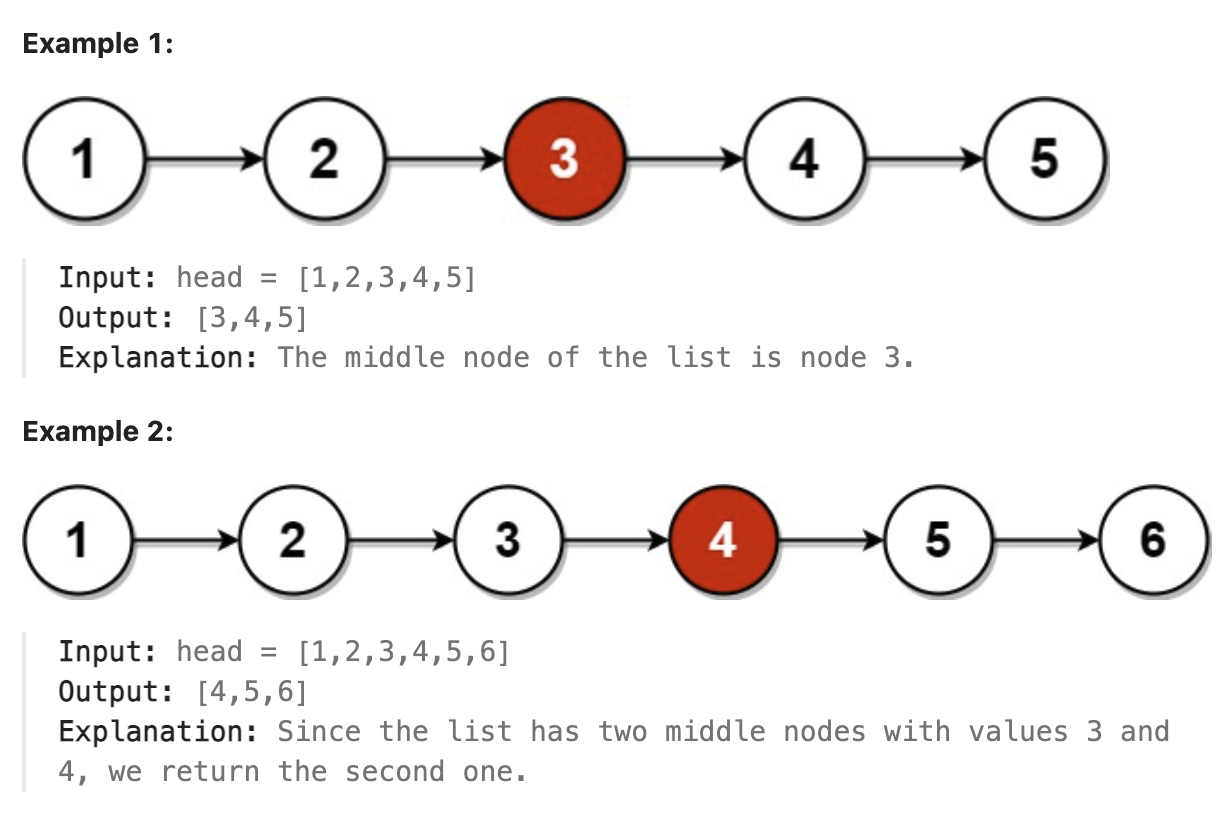
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题寻找链表中间位置的元素, 是一个经典快慢指针的题目. 只需要让快指针前进的速度为2, 慢指针为1, 则快指针到达链表末尾时慢指针正好指向中间位置. 要注意链表元素个数为奇数和偶数时的处理方法的不同.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
/**
|
||||||
|
* Definition for singly-linked list.
|
||||||
|
* type ListNode struct {
|
||||||
|
* Val int
|
||||||
|
* Next *ListNode
|
||||||
|
* }
|
||||||
|
*/
|
||||||
|
func middleNode(head *ListNode) *ListNode {
|
||||||
|
fast := head
|
||||||
|
slow := head
|
||||||
|
for fast.Next != nil && fast.Next.Next != nil{
|
||||||
|
fast = fast.Next.Next
|
||||||
|
slow = slow.Next
|
||||||
|
}
|
||||||
|
// consider list number is even
|
||||||
|
if fast.Next != nil{
|
||||||
|
slow = slow.Next
|
||||||
|
}
|
||||||
|
return slow
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
### 总结
|
||||||
|
|
||||||
|
本题是一道经典的快慢指针的简单题目, 进一步深化了快慢指针的应用.
|
||||||
|
Loading…
Reference in New Issue
Block a user