mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-06-07 11:52:07 +08:00
leetcode update
This commit is contained in:
parent
e27c9fd5c4
commit
ecac9ba497
@ -4929,3 +4929,183 @@ func findMaxK(nums []int) int {
|
|||||||
return result
|
return result
|
||||||
}
|
}
|
||||||
```
|
```
|
||||||
|
|
||||||
|
## day 66 2024-05-03
|
||||||
|
|
||||||
|
### 165. Compare Version Numbers
|
||||||
|
|
||||||
|
Given two version numbers, version1 and version2, compare them.
|
||||||
|
|
||||||
|
Version numbers consist of one or more revisions joined by a dot '.'. Each revision consists of digits and may contain leading zeros. Every revision contains at least one character. Revisions are 0-indexed from left to right, with the leftmost revision being revision 0, the next revision being revision 1, and so on. For example 2.5.33 and 0.1 are valid version numbers.
|
||||||
|
|
||||||
|
To compare version numbers, compare their revisions in left-to-right order. Revisions are compared using their integer value ignoring any leading zeros. This means that revisions 1 and 001 are considered equal. If a version number does not specify a revision at an index, then treat the revision as 0. For example, version 1.0 is less than version 1.1 because their revision 0s are the same, but their revision 1s are 0 and 1 respectively, and 0 < 1.
|
||||||
|
|
||||||
|
Return the following:
|
||||||
|
|
||||||
|
If version1 < version2, return -1.
|
||||||
|
If version1 > version2, return 1.
|
||||||
|
Otherwise, return 0.
|
||||||
|
|
||||||
|
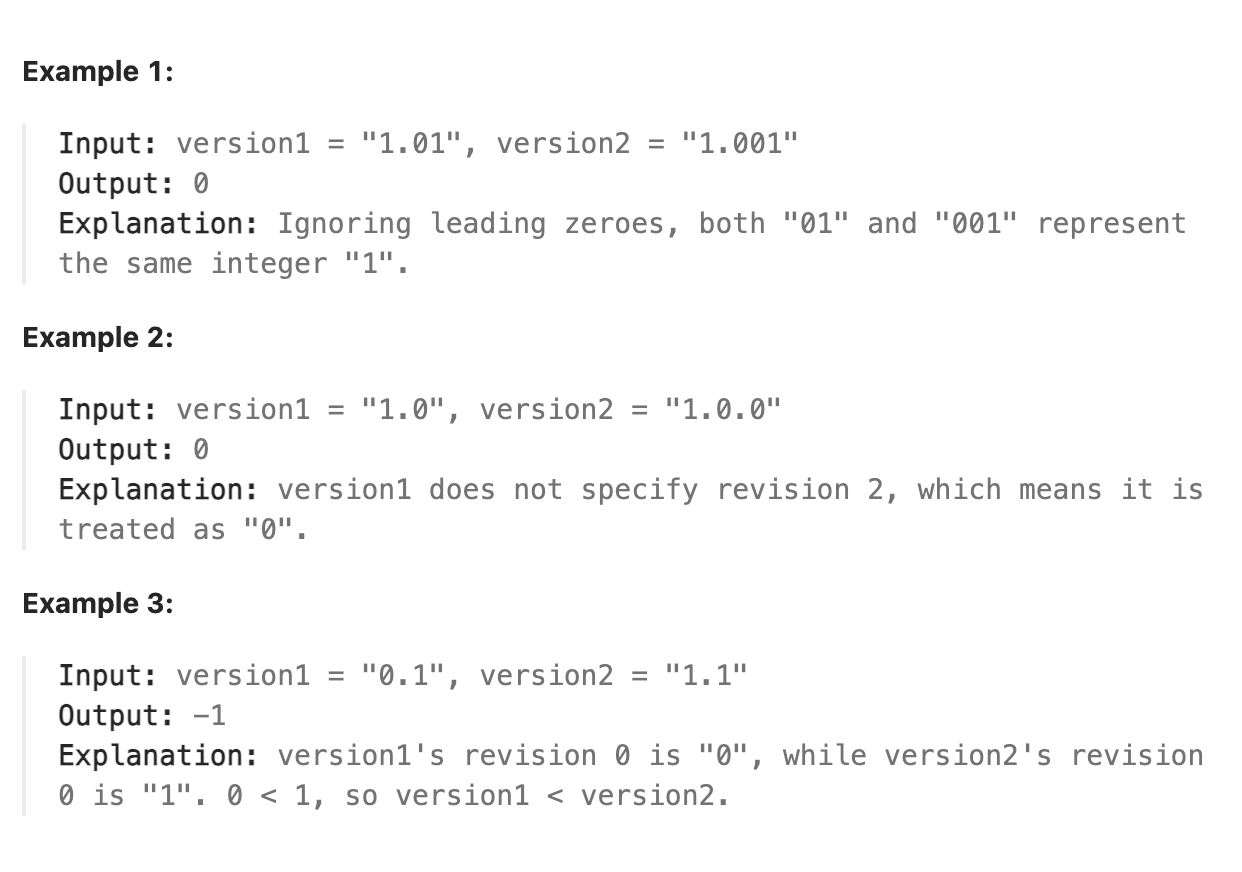
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题将字符串根据.号分割成字符数组, 再将两个字符数组转换成整数并比较大小.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
func compareVersion(version1 string, version2 string) int {
|
||||||
|
ver1slice := strings.Split(version1, ".")
|
||||||
|
ver2slice := strings.Split(version2, ".")
|
||||||
|
|
||||||
|
len1 := len(ver1slice)
|
||||||
|
len2 := len(ver2slice)
|
||||||
|
|
||||||
|
shortlen := 0
|
||||||
|
short := []string{}
|
||||||
|
long := []string{}
|
||||||
|
flag := 0
|
||||||
|
if len1 > len2{
|
||||||
|
short = ver2slice
|
||||||
|
long = ver1slice
|
||||||
|
shortlen = len2
|
||||||
|
flag = 1
|
||||||
|
}else{
|
||||||
|
short = ver1slice
|
||||||
|
long = ver2slice
|
||||||
|
shortlen = len1
|
||||||
|
flag = -1
|
||||||
|
}
|
||||||
|
|
||||||
|
for index, str := range short{
|
||||||
|
result1,_ := strconv.Atoi(str)
|
||||||
|
result2,_ := strconv.Atoi(long[index])
|
||||||
|
if result1 < result2{
|
||||||
|
return flag
|
||||||
|
}else if result1 > result2{
|
||||||
|
return -flag
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
for _, str := range long[shortlen:]{
|
||||||
|
result, _ := strconv.Atoi(str)
|
||||||
|
if result != 0{
|
||||||
|
return flag
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return 0
|
||||||
|
|
||||||
|
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
## day67 2024-05-04
|
||||||
|
|
||||||
|
### 881. Boats to Save People
|
||||||
|
|
||||||
|
You are given an array people where people[i] is the weight of the ith person, and an infinite number of boats where each boat can carry a maximum weight of limit. Each boat carries at most two people at the same time, provided the sum of the weight of those people is at most limit.
|
||||||
|
|
||||||
|
Return the minimum number of boats to carry every given person.
|
||||||
|
|
||||||
|
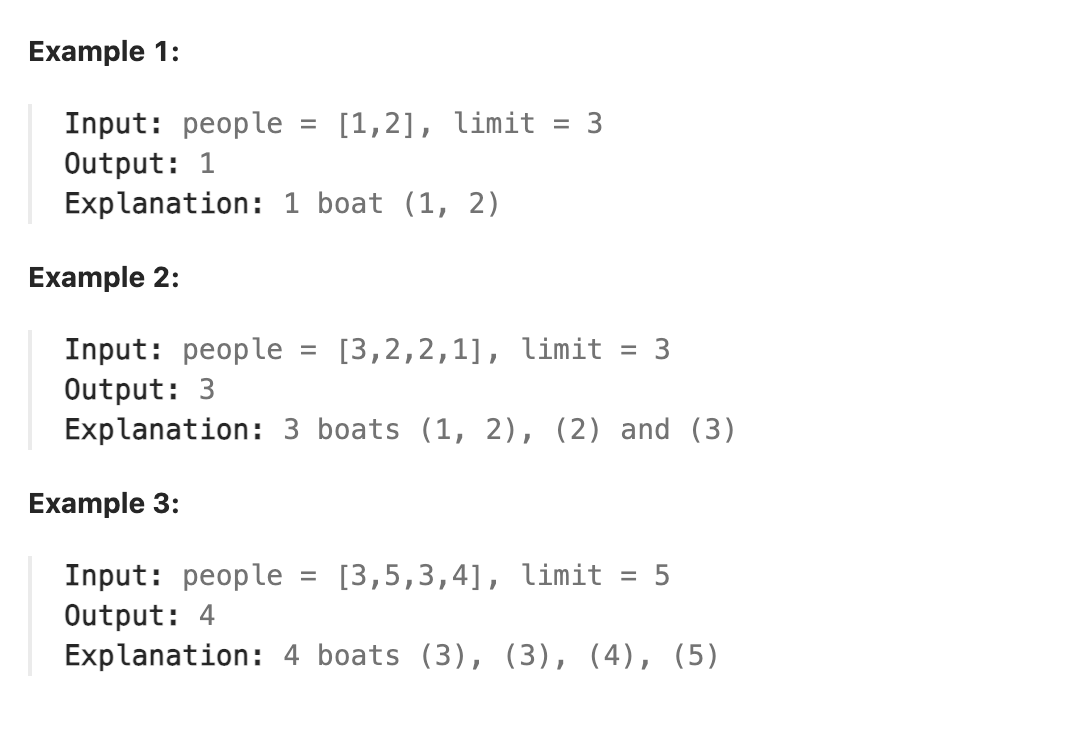
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题中每艘船只能乘坐两个人, 则两个人的重量和应小于船的限制limit, 因此重量较小的人可以和重量较大的人乘同一艘船, 但重量大于limit/2的人只能独自乘船, 不能与他人共乘. 则先统计各个重量的人数, 放在数组中, 数组下标表示对应的重量. 值为对应的人数. 从头遍历数组, 对于重量小于limit/2的人, 可以和与其重量和为limit及以下的人共同乘船, 从能与其共同乘船的最大重量开始向前遍历, 直到遍历过的重量的人数和与当前重量人数相同为止. 这些人每两两一组可以共乘同一艘船. 如果还有剩余, 说明后面已经全部遍历完, 考虑当前重量小于等于limit/2, 故两个人可以共同乘船, 将结果加上剩余人数的1/2, 奇数再加上一即可. 对于遍历到重量大于limit/2的情况, 因为不能和他人共同乘船, 直接将结果加上当前重量人数即可.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
func numRescueBoats(people []int, limit int) int {
|
||||||
|
peoples := make([]int, limit+1)
|
||||||
|
for _, value := range people{
|
||||||
|
peoples[value] = peoples[value] + 1
|
||||||
|
}
|
||||||
|
|
||||||
|
peoples[0] = peoples[limit]
|
||||||
|
result := 0
|
||||||
|
tail := 0
|
||||||
|
index := 0
|
||||||
|
value := 0
|
||||||
|
half := limit/2
|
||||||
|
for index <= limit{
|
||||||
|
value = peoples[index]
|
||||||
|
tail = limit-index
|
||||||
|
for tail > index{
|
||||||
|
value -= peoples[tail]
|
||||||
|
if value > 0{
|
||||||
|
result += peoples[tail]
|
||||||
|
peoples[tail] = 0
|
||||||
|
tail--
|
||||||
|
}else{
|
||||||
|
result += peoples[tail] + value
|
||||||
|
peoples[tail] = -value
|
||||||
|
value = 0
|
||||||
|
break
|
||||||
|
}
|
||||||
|
}
|
||||||
|
if index <= half{
|
||||||
|
result += value/2 + (value%2)
|
||||||
|
}else{
|
||||||
|
result += value
|
||||||
|
}
|
||||||
|
index++
|
||||||
|
}
|
||||||
|
|
||||||
|
return result
|
||||||
|
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
### 总结
|
||||||
|
|
||||||
|
本题关键在于船的人数有限制, 只能两个人, 如果船的人数无限而重量有限的话, 要尽可能将船装满, 从后向前遍历时要根据当前遍历到的重量减去对应的比较轻的重量的人数直到将船装满为止.
|
||||||
|
|
||||||
|
## day68 2024-05-05
|
||||||
|
|
||||||
|
### 237. Delete Node in a Linked List
|
||||||
|
|
||||||
|
There is a singly-linked list head and we want to delete a node node in it.
|
||||||
|
|
||||||
|
You are given the node to be deleted node. You will not be given access to the first node of head.
|
||||||
|
|
||||||
|
All the values of the linked list are unique, and it is guaranteed that the given node node is not the last node in the linked list.
|
||||||
|
|
||||||
|
Delete the given node. Note that by deleting the node, we do not mean removing it from memory. We mean:
|
||||||
|
|
||||||
|
The value of the given node should not exist in the linked list.
|
||||||
|
The number of nodes in the linked list should decrease by one.
|
||||||
|
All the values before node should be in the same order.
|
||||||
|
All the values after node should be in the same order.
|
||||||
|
Custom testing:
|
||||||
|
|
||||||
|
For the input, you should provide the entire linked list head and the node to be given node. node should not be the last node of the list and should be an actual node in the list.
|
||||||
|
We will build the linked list and pass the node to your function.
|
||||||
|
The output will be the entire list after calling your function.
|
||||||
|
|
||||||
|
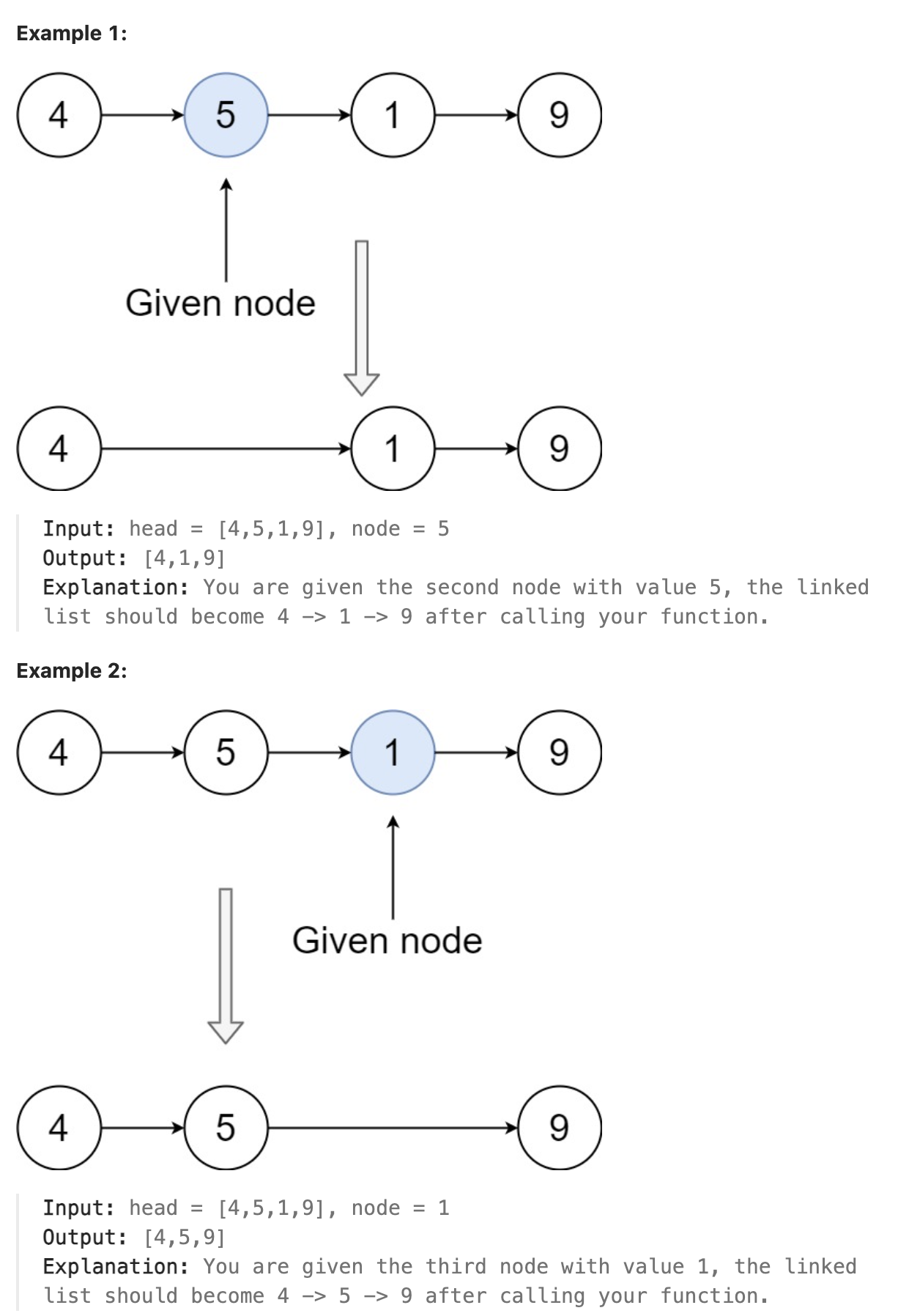
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题是一道基本的链表操作问题, 给定要删除的节点指针, 则不知道节点的前置节点指针, 只能通过遍历链表并将将后一个节点的值赋给前一个节点的方式来删除掉(覆盖)当前节点的值. 注意对倒数第二个节点, 当将最后一个节点的值赋给它后将其Next指针置为nil从而删掉原来的最后一个节点.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
/**
|
||||||
|
* Definition for singly-linked list.
|
||||||
|
* type ListNode struct {
|
||||||
|
* Val int
|
||||||
|
* Next *ListNode
|
||||||
|
* }
|
||||||
|
*/
|
||||||
|
func deleteNode(node *ListNode) {
|
||||||
|
for node.Next.Next != nil{
|
||||||
|
node.Val = node.Next.Val
|
||||||
|
node = node.Next
|
||||||
|
}
|
||||||
|
node.Val = node.Next.Val
|
||||||
|
node.Next = nil
|
||||||
|
}
|
||||||
|
```
|
||||||
|
Loading…
Reference in New Issue
Block a user