mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-06-07 11:52:07 +08:00
leetcode update
This commit is contained in:
parent
bcdcdfb395
commit
e967f7682d
@ -1616,3 +1616,65 @@ func leastInterval(tasks []byte, n int) int {
|
||||
return len(tasks) + idleSlots
|
||||
}
|
||||
```
|
||||
|
||||
## day23 2024-03-20
|
||||
|
||||
### 1669. Merge In Between Linked Lists
|
||||
|
||||
You are given two linked lists: list1 and list2 of sizes n and m respectively.
|
||||
|
||||
Remove list1's nodes from the ath node to the bth node, and put list2 in their place.
|
||||
|
||||
The blue edges and nodes in the following figure indicate the result:
|
||||
|
||||
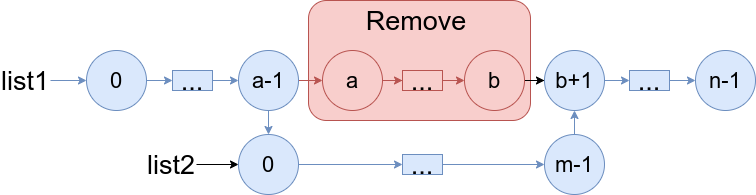
|
||||
|
||||
Build the result list and return its head.
|
||||
|
||||
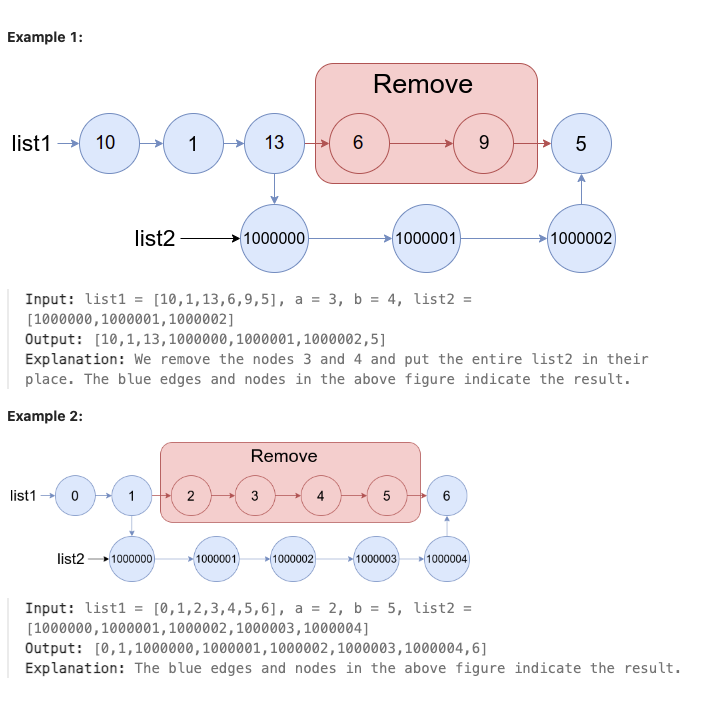
|
||||
|
||||
### 题解
|
||||
|
||||
本题为将链表中某段替换为指定的链表, 只需要遍历链表, 保存需要替换部分首尾两个节点的指针即可, 需要注意边界情况的处理, 即替换开头某段或者结尾某段链表时要处理空指针.
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
/**
|
||||
* Definition for singly-linked list.
|
||||
* type ListNode struct {
|
||||
* Val int
|
||||
* Next *ListNode
|
||||
* }
|
||||
*/
|
||||
func mergeInBetween(list1 *ListNode, a int, b int, list2 *ListNode) *ListNode {
|
||||
count := 0
|
||||
var before *ListNode
|
||||
var after *ListNode
|
||||
current := list1
|
||||
head := list1
|
||||
for count <= b {
|
||||
if count == a-1 {
|
||||
before = current
|
||||
}
|
||||
count++
|
||||
current = current.Next
|
||||
}
|
||||
after = current
|
||||
if before == nil {
|
||||
head = list2
|
||||
} else {
|
||||
before.Next = list2
|
||||
}
|
||||
current = list2
|
||||
for current.Next != nil {
|
||||
current = current.Next
|
||||
}
|
||||
current.Next = after
|
||||
return head
|
||||
}
|
||||
```
|
||||
|
||||
### 总结
|
||||
|
||||
本题保存了首尾两个指针的地址, 是典型的用空间换时间的思路, 不过实际应用过程中可能还要根据语言注意被动态分配出去的空间回收的问题.
|
||||
|
Loading…
Reference in New Issue
Block a user