mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-04-19 21:42:07 +08:00
leetcode update
This commit is contained in:
parent
9c3ddf2e40
commit
e2a41d0dcc
@ -22981,3 +22981,44 @@ public:
|
|||||||
}
|
}
|
||||||
};
|
};
|
||||||
```
|
```
|
||||||
|
## day340 2025-02-13
|
||||||
|
### 3066. Minimum Operations to Exceed Threshold Value II
|
||||||
|
You are given a 0-indexed integer array nums, and an integer k.
|
||||||
|
|
||||||
|
In one operation, you will:
|
||||||
|
|
||||||
|
Take the two smallest integers x and y in nums.
|
||||||
|
Remove x and y from nums.
|
||||||
|
Add min(x, y) * 2 + max(x, y) anywhere in the array.
|
||||||
|
Note that you can only apply the described operation if nums contains at least two elements.
|
||||||
|
|
||||||
|
Return the minimum number of operations needed so that all elements of the array are greater than or equal to k.
|
||||||
|
|
||||||
|
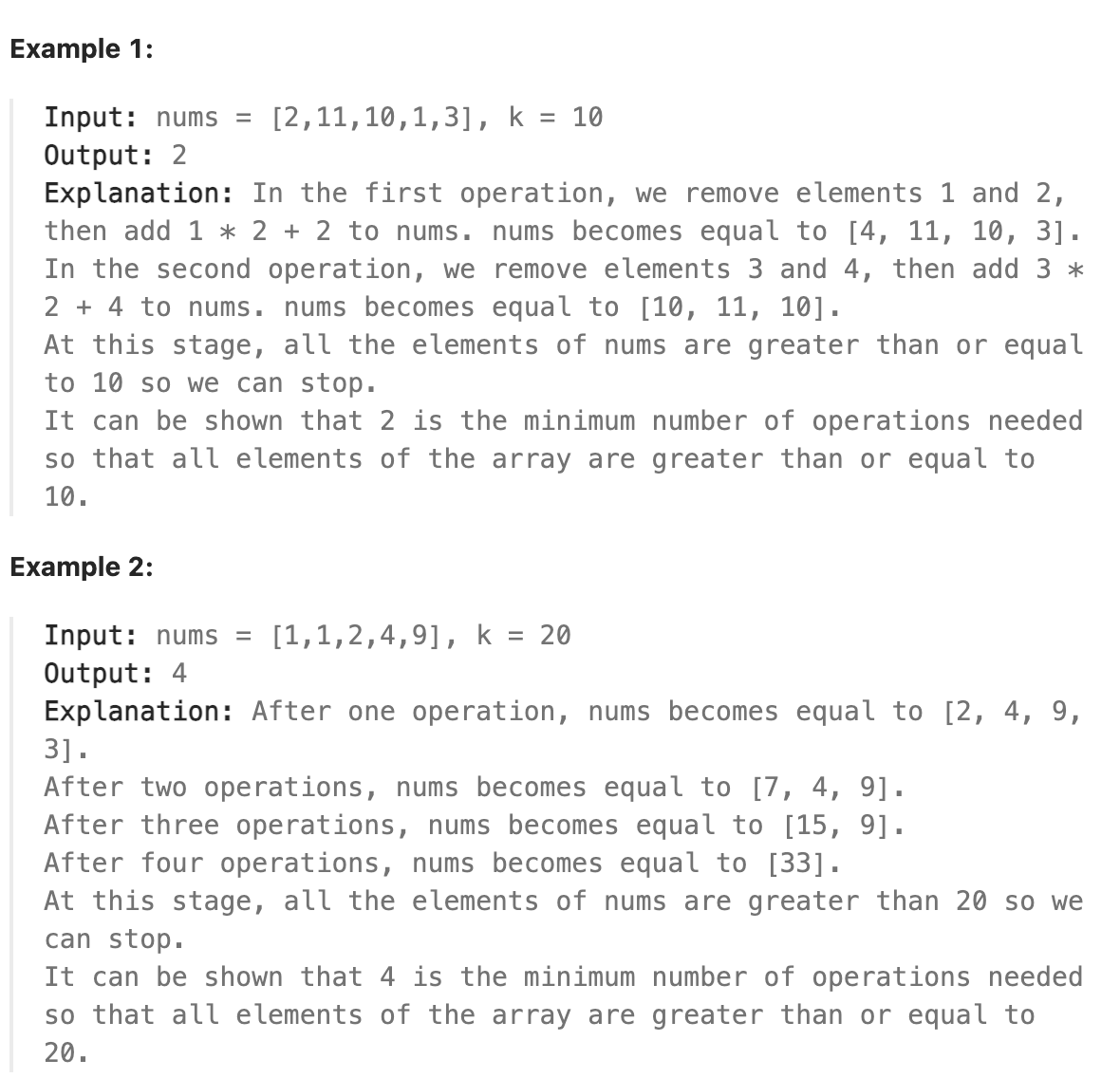
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
本题注意到题目中会多次用到nums中的最小值,操作中的第三步min(x,y)*2+max(x,y)如果先拿到nums中的最小值,删掉这个最小值,再取出nums中的最小值则先取得的一定是更小的那个,后取得的是更大的那个。则此处可使用最小堆,弹出堆顶元素即为nums中的最小值,执行题目所述操作得到新的数字后再将新的数字插入堆中,如此反复直到堆顶元素大于等于k,返回记录的操作次数。
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
```cpp
|
||||||
|
class Solution {
|
||||||
|
public:
|
||||||
|
int minOperations(vector<int>& nums, int k) {
|
||||||
|
priority_queue<long long int,vector<long long int>,greater<long long int>> min_heap(nums.begin(), nums.end());
|
||||||
|
int opnum = 0;
|
||||||
|
long long int small = 0;
|
||||||
|
long long int big = 0;
|
||||||
|
while(min_heap.top() < k){
|
||||||
|
small = min_heap.top();
|
||||||
|
min_heap.pop();
|
||||||
|
big = min_heap.top();
|
||||||
|
min_heap.pop();
|
||||||
|
min_heap.push(small*2+big);
|
||||||
|
opnum++;
|
||||||
|
}
|
||||||
|
return opnum;
|
||||||
|
}
|
||||||
|
};
|
||||||
|
```
|
||||||
|
### 总结
|
||||||
|
注意到想构建优先级队列需要定义好三个属性,一是优先级队列中保存的数据类型,二是用来保存这些数据的容器类型,三则是优先级队列中的比较函数,定义好这三个抽象出来的属性就可以定义好一个优先级队列。
|
||||||
|
Loading…
Reference in New Issue
Block a user