mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-06-20 20:22:07 +08:00
leetcode update
This commit is contained in:
parent
9690039188
commit
d8084d2a16
@ -6605,7 +6605,9 @@ func numSteps(s string) int {
|
||||
本题直接从最后一位开始向前遍历, 遇到0直接将结果加1, 遇到1设定一个标记进位的标记变量为1, 同时将结果加2, 如此重复即可. 注意在每次判断当前位是0还是1之前要将原本的当前位值加上进位的标记变量再判断.
|
||||
|
||||
## day94 2024-05-30
|
||||
|
||||
### 1442. Count Triplets That Can Form Two Arrays of Equal XOR
|
||||
|
||||
Given an array of integers arr.
|
||||
|
||||
We want to select three indices i, j and k where (0 <= i < j <= k < arr.length).
|
||||
@ -6625,6 +6627,7 @@ Return the number of triplets (i, j and k) Where a == b.
|
||||
题目中要求i-j和j-k之间的数组元素全部异或后的值相等, 而i<j<=k. 两个相同的值异或后的值为0, 因此可以得出结论, i-k之间的数异或后的值为0(i-j,j-k范围内的数异或后的值相同). i-k区间内的数异或后的值为0, 就意味着从头开始到i和从头开始到k这两个区间的数异或的值相等(不一定为0). 遍历数组, 计算到当前下标处的异或值. 并将异或值作为key, 下标作为value保存到map中, 计算得到异或值后查询map, 找到与当前下标异或值相等的全部下标, 并将这些区间内的所有可行分割(长度为n的区间有n-1种分割方法, 直观理解放木板到这个区间的数字之间, 一共能放几块就是几种分割方法)数目添加到结果中.
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
func countTriplets(arr []int) int {
|
||||
xormap := map[int][]int{}
|
||||
@ -6647,3 +6650,110 @@ func countTriplets(arr []int) int {
|
||||
return result
|
||||
}
|
||||
```
|
||||
|
||||
## day95 2024-05-31
|
||||
|
||||
### 260. Single Number III
|
||||
|
||||
Given an integer array nums, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. You can return the answer in any order.
|
||||
|
||||
You must write an algorithm that runs in linear runtime complexity and uses only constant extra space.
|
||||
|
||||
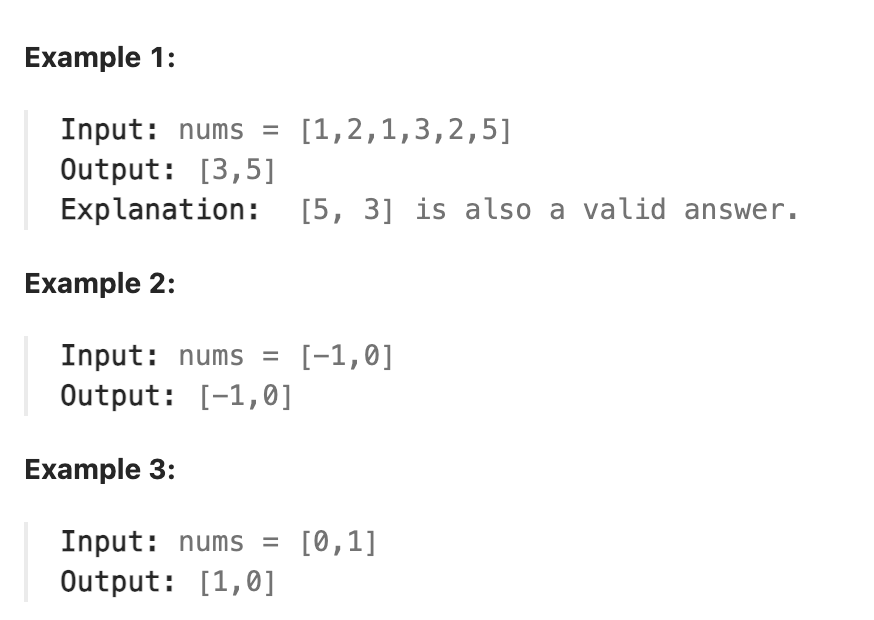
|
||||
|
||||
### 题解
|
||||
|
||||
本题之前有一个前置问题, 即找到所有数中仅有一个出现了一次的数. 这个问题通过位运算的技巧, 两个相同的数异或之后得到的结果为0. 而唯一一个只出现了一次的数最终会被剩下. 那么对应有两个数都只出现了一次的情况怎么处理, 首先类似的,通过将所有的数异或最终实际上相当于只剩下两个数异或. 此时分辨不出来两个数分别是什么, 但仍保存了一些信息, 因为数字不同的位异或后的结果为1. 则可以通过对应的位为1了解到在对应位两个数不同. 则可以通过按照得到的两个数不同的位将所有数分割为两组, 考虑到在组内其他数个数均为两个, 则在组内使用对前置问题的解决方法即可分别得到两个数.
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
func singleNumber(nums []int) []int {
|
||||
if len(nums) == 2{
|
||||
return nums
|
||||
}
|
||||
result := 0
|
||||
for _, value := range nums{
|
||||
result = result ^ value
|
||||
}
|
||||
bit := 0
|
||||
for i:=0;i<32;i++{
|
||||
if ((result >> i) & 1) == 1{
|
||||
bit = i
|
||||
}
|
||||
}
|
||||
result1 := 0
|
||||
result2 := 0
|
||||
for _, value := range nums{
|
||||
if ((value >> bit) & 1) == 1{
|
||||
result1 = result1 ^ value
|
||||
}else{
|
||||
result2 = result2 ^ value
|
||||
}
|
||||
}
|
||||
return []int{result1, result2}
|
||||
}
|
||||
```
|
||||
|
||||
## day96 2024-06-01
|
||||
|
||||
### 3110. Score of a String
|
||||
|
||||
You are given a string s. The score of a string is defined as the sum of the absolute difference between the ASCII values of adjacent characters.
|
||||
|
||||
Return the score of s.
|
||||
|
||||
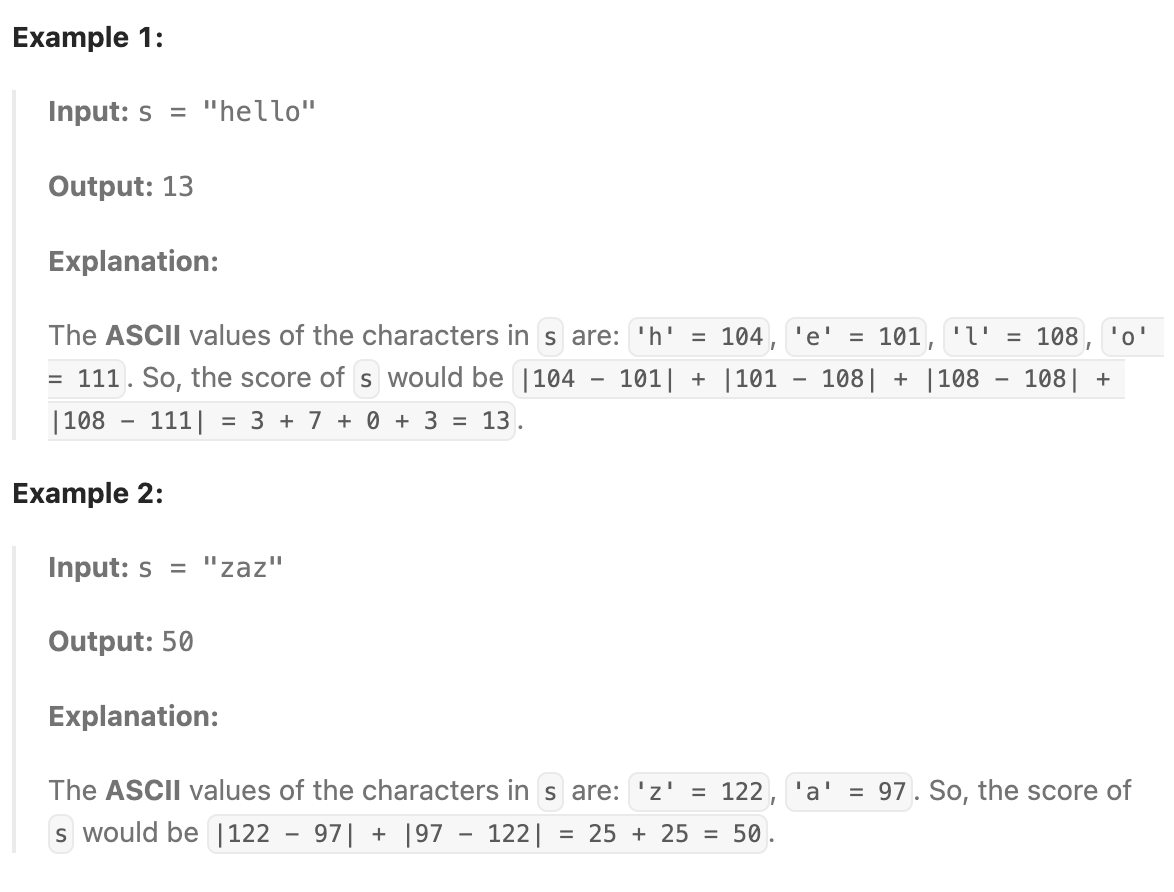
|
||||
|
||||
### 题解
|
||||
|
||||
比较基础, 按照题目要求计算相邻字符的差的绝对值之和即可
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
func scoreOfString(s string) int {
|
||||
result := 0
|
||||
for i,_ := range s[1:]{
|
||||
if s[i] < s[i+1]{
|
||||
result += int(s[i+1] - s[i])
|
||||
}else{
|
||||
result += int(s[i] - s[i+1])
|
||||
}
|
||||
}
|
||||
return result
|
||||
}
|
||||
```
|
||||
|
||||
## day97 2024-06-02
|
||||
|
||||
### 344. Reverse String
|
||||
|
||||
Write a function that reverses a string. The input string is given as an array of characters s.
|
||||
|
||||
You must do this by modifying the input array in-place with O(1) extra memory.
|
||||
|
||||
Example 1:
|
||||
|
||||
Input: s = ["h","e","l","l","o"]
|
||||
Output: ["o","l","l","e","h"]
|
||||
|
||||
Example 2:
|
||||
|
||||
Input: s = ["H","a","n","n","a","h"]
|
||||
Output: ["h","a","n","n","a","H"]
|
||||
|
||||
### 题解
|
||||
|
||||
将字符串逆转也是一道简单题, 要求只能使用常数额外空间且直接在原始字符串上逆转, 因为原始字符串以数组形式保存, 可以分别从首尾开始向中间遍历数组并交换首尾的字符即可完成逆转操作.
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
func reverseString(s []byte) {
|
||||
length := len(s) - 1
|
||||
for i:=0;0+i <= length-i;i++{
|
||||
s[i], s[length-i] = s[length-i], s[i]
|
||||
}
|
||||
}
|
||||
```
|
||||
|
Loading…
Reference in New Issue
Block a user