mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-07-03 05:02:07 +08:00
leetcode update
This commit is contained in:
parent
b862dcbe08
commit
cec8338f6e
@ -3454,3 +3454,77 @@ func lastnode(root *TreeNode, value int) int{
|
|||||||
### 总结
|
### 总结
|
||||||
|
|
||||||
运行时间2ms本以为算法效率不够高, 结果看了看运行时间0ms的代码, 代码逻辑和我一模一样, 可能被优化的点在于递归函数中可以先判断是否为叶子节点, 再去创建sum变量. 其实这种将属性传递给子节点的方法在编译原理中的语义分析阶段很常用, 也就是属性文法, 可以看作一种继承属性, 更加详细的内容可以查阅编译原理相关的书籍.
|
运行时间2ms本以为算法效率不够高, 结果看了看运行时间0ms的代码, 代码逻辑和我一模一样, 可能被优化的点在于递归函数中可以先判断是否为叶子节点, 再去创建sum变量. 其实这种将属性传递给子节点的方法在编译原理中的语义分析阶段很常用, 也就是属性文法, 可以看作一种继承属性, 更加详细的内容可以查阅编译原理相关的书籍.
|
||||||
|
|
||||||
|
## day49 2024-04-16
|
||||||
|
|
||||||
|
### 623. Add One Row to Tree
|
||||||
|
|
||||||
|
Given the root of a binary tree and two integers val and depth, add a row of nodes with value val at the given depth depth.
|
||||||
|
|
||||||
|
Note that the root node is at depth 1.
|
||||||
|
|
||||||
|
The adding rule is:
|
||||||
|
|
||||||
|
Given the integer depth, for each not null tree node cur at the depth depth - 1, create two tree nodes with value val as cur's left subtree root and right subtree root.
|
||||||
|
|
||||||
|
cur's original left subtree should be the left subtree of the new left subtree root.
|
||||||
|
|
||||||
|
cur's original right subtree should be the right subtree of the new right subtree root.
|
||||||
|
|
||||||
|
If depth == 1 that means there is no depth depth - 1 at all, then create a tree node with value val as the new root of the whole original tree, and the original tree is the new root's left subtree.
|
||||||
|
|
||||||
|
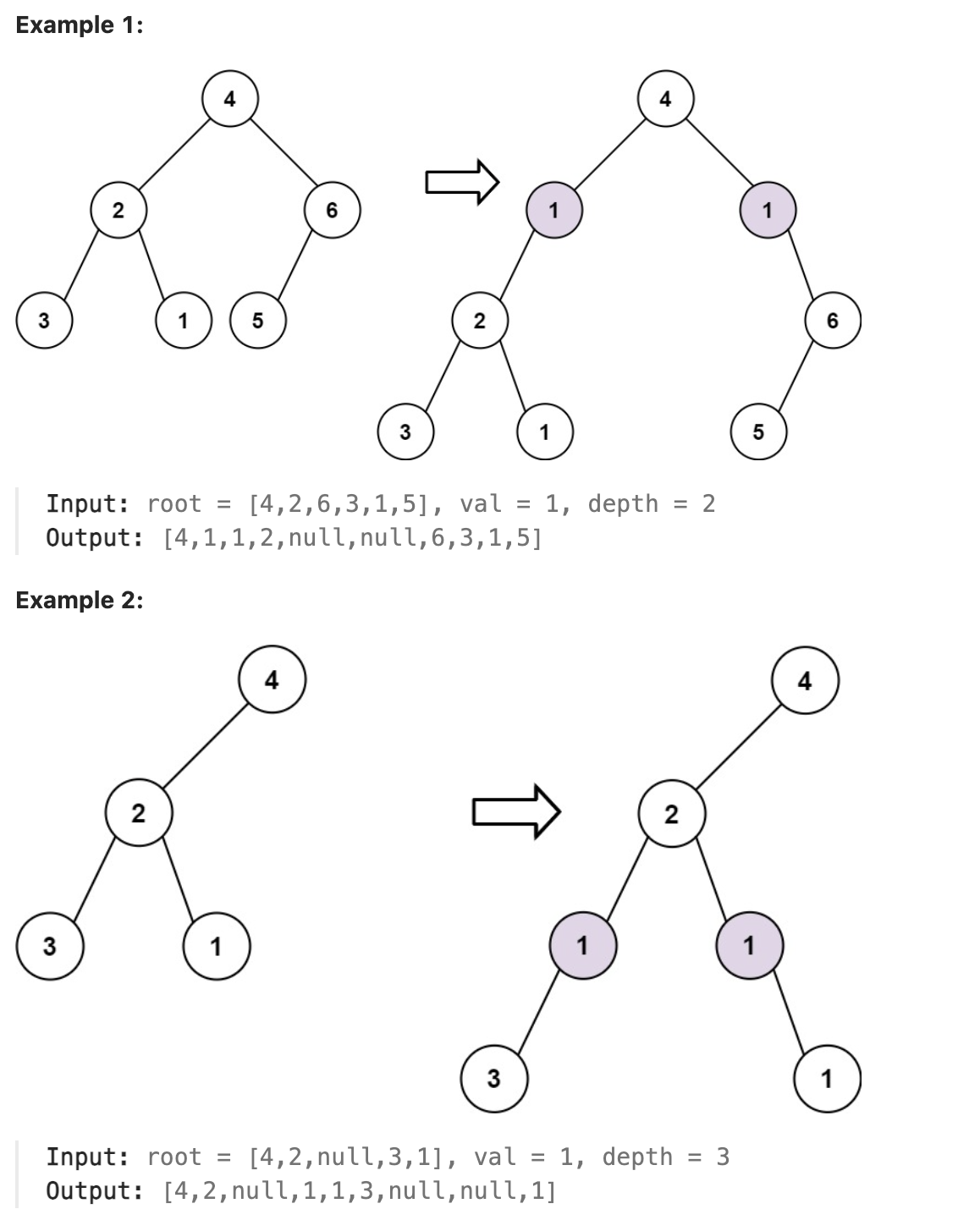
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题使用层序遍历, 遍历到对应深度减一的节点时按规则插入节点, 注意处理只有一个节点的特殊情况即可.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
/**
|
||||||
|
* Definition for a binary tree node.
|
||||||
|
* type TreeNode struct {
|
||||||
|
* Val int
|
||||||
|
* Left *TreeNode
|
||||||
|
* Right *TreeNode
|
||||||
|
* }
|
||||||
|
*/
|
||||||
|
func addOneRow(root *TreeNode, val int, depth int) *TreeNode {
|
||||||
|
newnode := &TreeNode{val, nil, nil}
|
||||||
|
if depth == 1{
|
||||||
|
newnode.Left = root
|
||||||
|
return newnode
|
||||||
|
}
|
||||||
|
queue := []*TreeNode{root}
|
||||||
|
depths := 1
|
||||||
|
for len(queue) != 0{
|
||||||
|
if depths == depth-1{
|
||||||
|
for _,value := range queue{
|
||||||
|
dunode := &TreeNode{val,nil,nil}
|
||||||
|
dunode.Left = value.Left
|
||||||
|
value.Left = dunode
|
||||||
|
dunoderight := &TreeNode{val,nil,nil}
|
||||||
|
dunoderight.Right = value.Right
|
||||||
|
value.Right = dunoderight
|
||||||
|
}
|
||||||
|
return root
|
||||||
|
}
|
||||||
|
for _,value := range queue{
|
||||||
|
if value.Left != nil{
|
||||||
|
queue = append(queue, value.Left)
|
||||||
|
}
|
||||||
|
if value.Right != nil{
|
||||||
|
queue = append(queue, value.Right)
|
||||||
|
}
|
||||||
|
queue = queue[1:]
|
||||||
|
}
|
||||||
|
depths++
|
||||||
|
}
|
||||||
|
return root
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
### 总结
|
||||||
|
|
||||||
|
本题也可使用dfs结合变量记录当前深度来解决.
|
||||||
|
Loading…
Reference in New Issue
Block a user