mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-04-20 05:52:07 +08:00
add content for leetcode post
This commit is contained in:
parent
d2019dad9f
commit
a393f9719a
@ -73,3 +73,55 @@ func postorder(father *TreeNode) int {
|
|||||||
}
|
}
|
||||||
}
|
}
|
||||||
```
|
```
|
||||||
|
|
||||||
|
## day2 2024-02-28
|
||||||
|
|
||||||
|
### 513. Find Bottom Left Tree Value
|
||||||
|
|
||||||
|
Given the root of a binary tree, return the leftmost value in the last row of the tree.
|
||||||
|
|
||||||
|
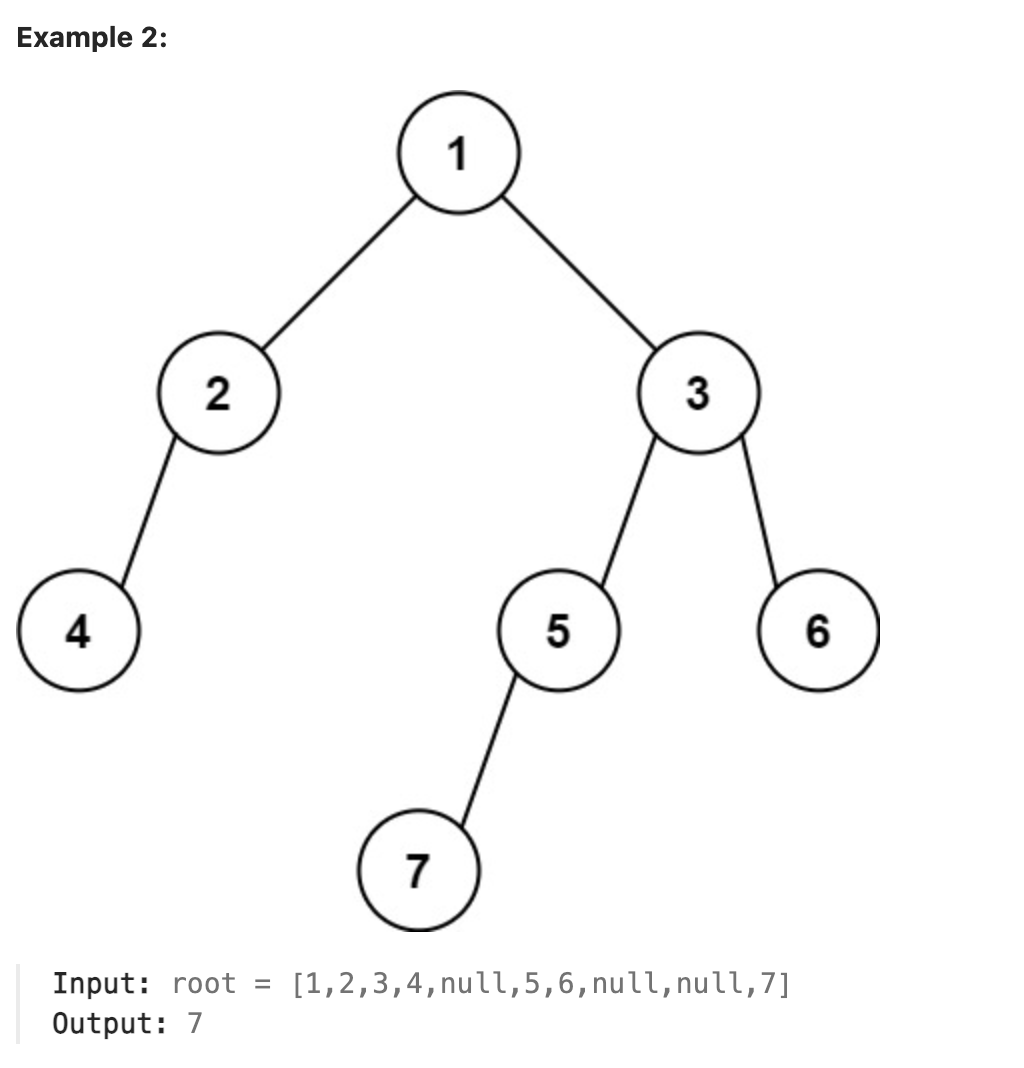
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
找到二叉树最底层最左边的节点值, 用层序遍历遍历到最后一层找到最后一层最左边节点的值即可. 实现层序遍历可以使用一个队列, 将当前层节点的所有子节点入队后将当前层节点出队. 在go中可以使用切片实现一个队列. 使用一个标记变量记录当前层所有节点是否有子节点, 若无子节点则当前层为最低层, 返回当前层最左侧节点的值(此时队列中第一个节点的值).
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
/**
|
||||||
|
* Definition for a binary tree node.
|
||||||
|
* type TreeNode struct {
|
||||||
|
* Val int
|
||||||
|
* Left *TreeNode
|
||||||
|
* Right *TreeNode
|
||||||
|
* }
|
||||||
|
*/
|
||||||
|
func findBottomLeftValue(root *TreeNode) int {
|
||||||
|
queue := []*TreeNode{root}
|
||||||
|
lowest := false
|
||||||
|
key := -1
|
||||||
|
var father *TreeNode
|
||||||
|
for !lowest{
|
||||||
|
queue = queue[key+1:]
|
||||||
|
lowest = true
|
||||||
|
for key, father = range queue{
|
||||||
|
if(father.Left != nil || father.Right != nil){
|
||||||
|
lowest = false
|
||||||
|
if(father.Left != nil){
|
||||||
|
queue = append(queue, father.Left)
|
||||||
|
}
|
||||||
|
if(father.Right != nil){
|
||||||
|
queue = append(queue, father.Right)
|
||||||
|
}
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
}
|
||||||
|
return queue[0].Val
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
### 总结
|
||||||
|
|
||||||
|
在题解中看到了一个使用深度优先搜索的方法, 记录当前搜索到的层级, 始终保存最大层级的第一个被搜索到的值, 因为使用的是后序遍历, 则每次遇到的当前层大于保存的最大层级时, 该节点就为新的最大层级的第一个节点, 即题目中要求的最左值(leftmost). 算法时间复杂度为O(n)------只遍历一次所有节点.
|
||||||
|
Loading…
Reference in New Issue
Block a user