mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-07-11 01:03:47 +08:00
leetcode update
This commit is contained in:
@ -993,3 +993,52 @@ func removeZeroSumSublists(head *ListNode) *ListNode {
|
||||
### 总结
|
||||
|
||||
删除中间部分节点的前缀和对应的key的项时, 考虑到中间部分的和一定为0, 因此用sum去累加中间部分节点的值并依次删除, 最后得到的sum就和删除节点开始前的sum相同. 本题一是要清楚通过前缀和来寻找连续的和为0的序列, 另一方面则是时刻记住这个序列的和为0的特性. 其实本题有一种代表元的抽象思想. 可以将一组和为0的序列看为一个, 其在加和过程中与不存在的节点具有等价性. 不影响和的变化.
|
||||
|
||||
## day16 2024-03-13
|
||||
|
||||
### 2485. Find the Pivot Integer
|
||||
|
||||
Given a positive integer n, find the pivot integer x such that:
|
||||
|
||||
The sum of all elements between 1 and x inclusively equals the sum of all elements between x and n inclusively.
|
||||
Return the pivot integer x. If no such integer exists, return -1. It is guaranteed that there will be at most one pivot index for the given input.
|
||||
|
||||
Example 1:
|
||||
|
||||
Input: n = 8
|
||||
Output: 6
|
||||
Explanation: 6 is the pivot integer since: 1 + 2 + 3 + 4 + 5 + 6 = 6 + 7 + 8 = 21.
|
||||
Example 2:
|
||||
|
||||
Input: n = 1
|
||||
Output: 1
|
||||
Explanation: 1 is the pivot integer since: 1 = 1.
|
||||
Example 3:
|
||||
|
||||
Input: n = 4
|
||||
Output: -1
|
||||
Explanation: It can be proved that no such integer exist.
|
||||
|
||||
### 题解
|
||||
|
||||
本题使用数学方法进行计算
|
||||
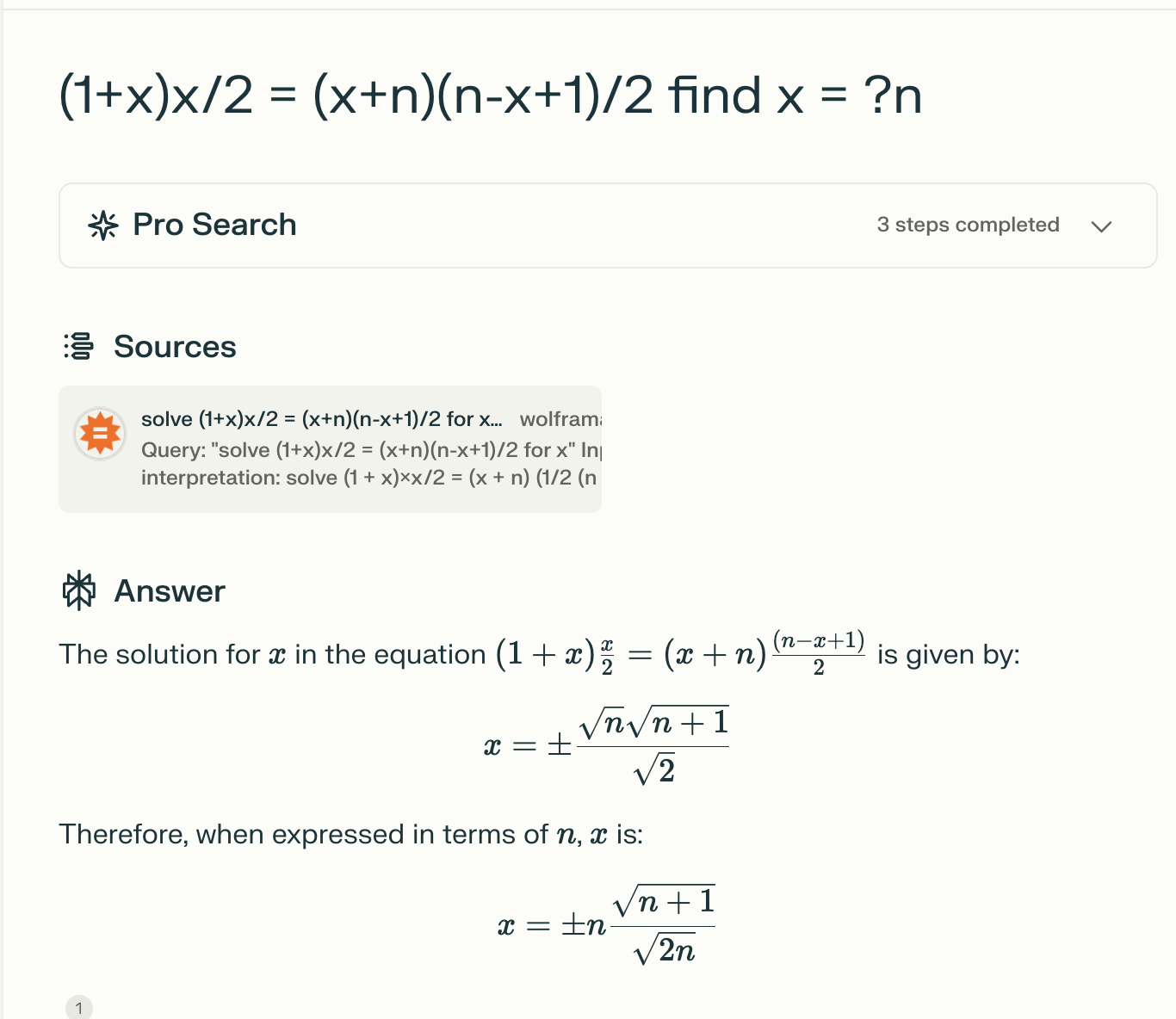
|
||||
根据公式直接求出x的值即可, 注意考虑到精度问题, 要对最终得到的运算结果与取整后的数字的差的绝对值进行判断, 小于一定限度即可认为是整数值.
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
func pivotInteger(n int) int {
|
||||
n_float := float64(n)
|
||||
x := n_float*math.Sqrt(n_float+1)/math.Sqrt(2*n_float)
|
||||
if math.Abs(x-float64(int(x))) < 0.0000001{
|
||||
return int(x)
|
||||
}else {
|
||||
return -1
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### 总结
|
||||
|
||||
查看他人解法发现本题其实也可以使用前缀和进行求解, 将每个下标位置处的前缀和求出并保存, 倒序遍历数组, 将后面的数的和与当前位置的前缀和比较即可, 示例代码
|
||||
|
Reference in New Issue
Block a user