mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-07-03 05:02:07 +08:00
add leetcode content
This commit is contained in:
parent
ed1e385216
commit
8f269ef2b1
@ -518,3 +518,54 @@ func bagOfTokensScore(tokens []int, power int) int {
|
|||||||
### 总结
|
### 总结
|
||||||
|
|
||||||
在实现过程中, 起初使用一个数组来保存每次的score值, 这样空间复杂度略大, 后来查看他人代码, 发现只需要一个max变量来保存当前最大的score值, 并在每次循环计算当前轮次的score值时与当前的最大值比较并根据二者大小更新max变量的值即可, 这样只需要O(1)的空间复杂度.
|
在实现过程中, 起初使用一个数组来保存每次的score值, 这样空间复杂度略大, 后来查看他人代码, 发现只需要一个max变量来保存当前最大的score值, 并在每次循环计算当前轮次的score值时与当前的最大值比较并根据二者大小更新max变量的值即可, 这样只需要O(1)的空间复杂度.
|
||||||
|
|
||||||
|
## day8 2024-03-05
|
||||||
|
|
||||||
|
### 1750. Minimum Length of String After Deleting Similar Ends
|
||||||
|
|
||||||
|
Given a string s consisting only of characters 'a', 'b', and 'c'. You are asked to apply the following algorithm on the string any number of times:
|
||||||
|
|
||||||
|
1. Pick a non-empty prefix from the string s where all the characters in the prefix are equal.
|
||||||
|
2. Pick a non-empty suffix from the string s where all the characters in this suffix are equal.
|
||||||
|
3. The prefix and the suffix should not intersect at any index.
|
||||||
|
4. The characters from the prefix and suffix must be the same.
|
||||||
|
5. Delete both the prefix and the suffix.
|
||||||
|
Return the minimum length of s after performing the above operation any number of times (possibly zero times).
|
||||||
|
|
||||||
|
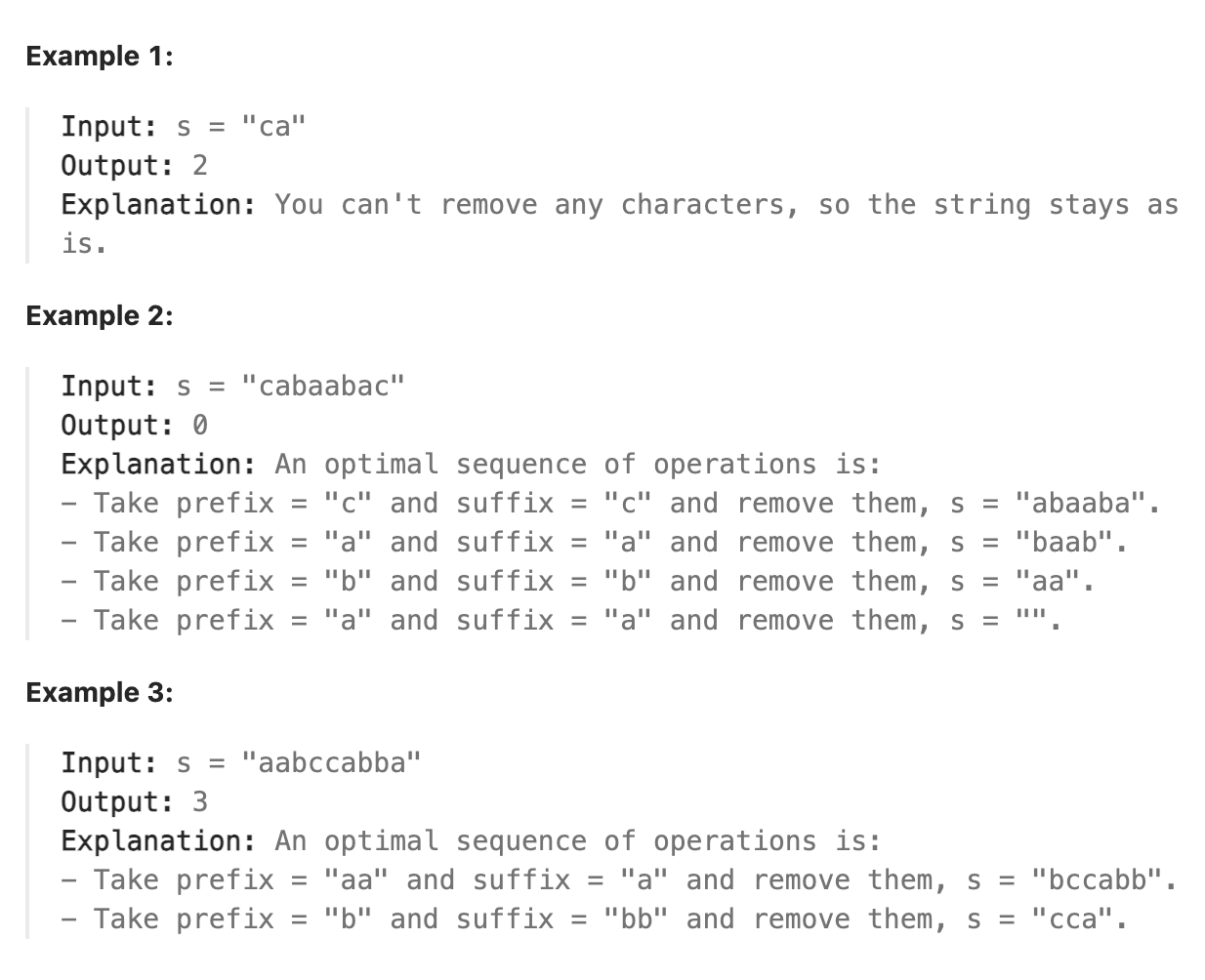
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题的题目略显复杂, 读起来乍一看让人不明所以, 实际上题目的目标即为从字符串的前后同时删除相同的字符, 删除时只要是相同的就全部删除, 如最前面有一个a最后面有3个a则同时将这四个a删除. 删除的字符串下标不能相交. 思路比较简单, 判断字符串的前后字符是否相同, 然后删除前后的连续相同字符即可, 注意下标不要重叠. 同时注意边界情况, 字符串只有一个字符的情况单独处理一下(直接返回1).
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
func minimumLength(s string) int {
|
||||||
|
forward := 0
|
||||||
|
backward := 0
|
||||||
|
for s[0] == s[len(s)-1]{
|
||||||
|
if len(s) == 1{
|
||||||
|
return 1
|
||||||
|
} else {
|
||||||
|
forward = 0
|
||||||
|
backward = len(s)-1
|
||||||
|
for forward<backward && s[forward+1] == s[forward] {
|
||||||
|
forward++
|
||||||
|
}
|
||||||
|
for backward>forward && s[backward-1] == s[backward] {
|
||||||
|
backward--
|
||||||
|
}
|
||||||
|
if forward == backward{
|
||||||
|
return 0
|
||||||
|
}
|
||||||
|
s = s[forward+1:backward]
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return len(s)
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
### 总结
|
||||||
|
|
||||||
|
本题值得注意的地方在于for循环中条件的设置, 可能会忽略与运算符两侧条件表达式的前后顺序, 但由于短路机制的存在, 与运算符两侧表达式的前后顺序有时非常重要, 例如在本题中, 如果将forward<backward条件设置在前面, 则当forward到达数组的边界时会直接退出循环, 但若将相等判断放在前面, 会因为当forward到达数组边界时forward+1下标越界而出错.
|
||||||
|
Loading…
Reference in New Issue
Block a user