mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-04-20 05:52:07 +08:00
leetcode update
This commit is contained in:
parent
5b9f2d1723
commit
8bacaf9282
@ -1463,3 +1463,71 @@ func insert(intervals [][]int, newInterval []int) [][]int {
|
|||||||
return res
|
return res
|
||||||
}
|
}
|
||||||
```
|
```
|
||||||
|
|
||||||
|
## day21 2024-03-18
|
||||||
|
|
||||||
|
### 452. Minimum Number of Arrows to Burst Balloons
|
||||||
|
|
||||||
|
There are some spherical balloons taped onto a flat wall that represents the XY-plane. The balloons are represented as a 2D integer array points where points[i] = [xstart, xend] denotes a balloon whose horizontal diameter stretches between xstart and xend. You do not know the exact y-coordinates of the balloons.
|
||||||
|
|
||||||
|
Arrows can be shot up directly vertically (in the positive y-direction) from different points along the x-axis. A balloon with xstart and xend is burst by an arrow shot at x if xstart <= x <= xend. There is no limit to the number of arrows that can be shot. A shot arrow keeps traveling up infinitely, bursting any balloons in its path.
|
||||||
|
|
||||||
|
Given the array points, return the minimum number of arrows that must be shot to burst all balloons.
|
||||||
|
|
||||||
|
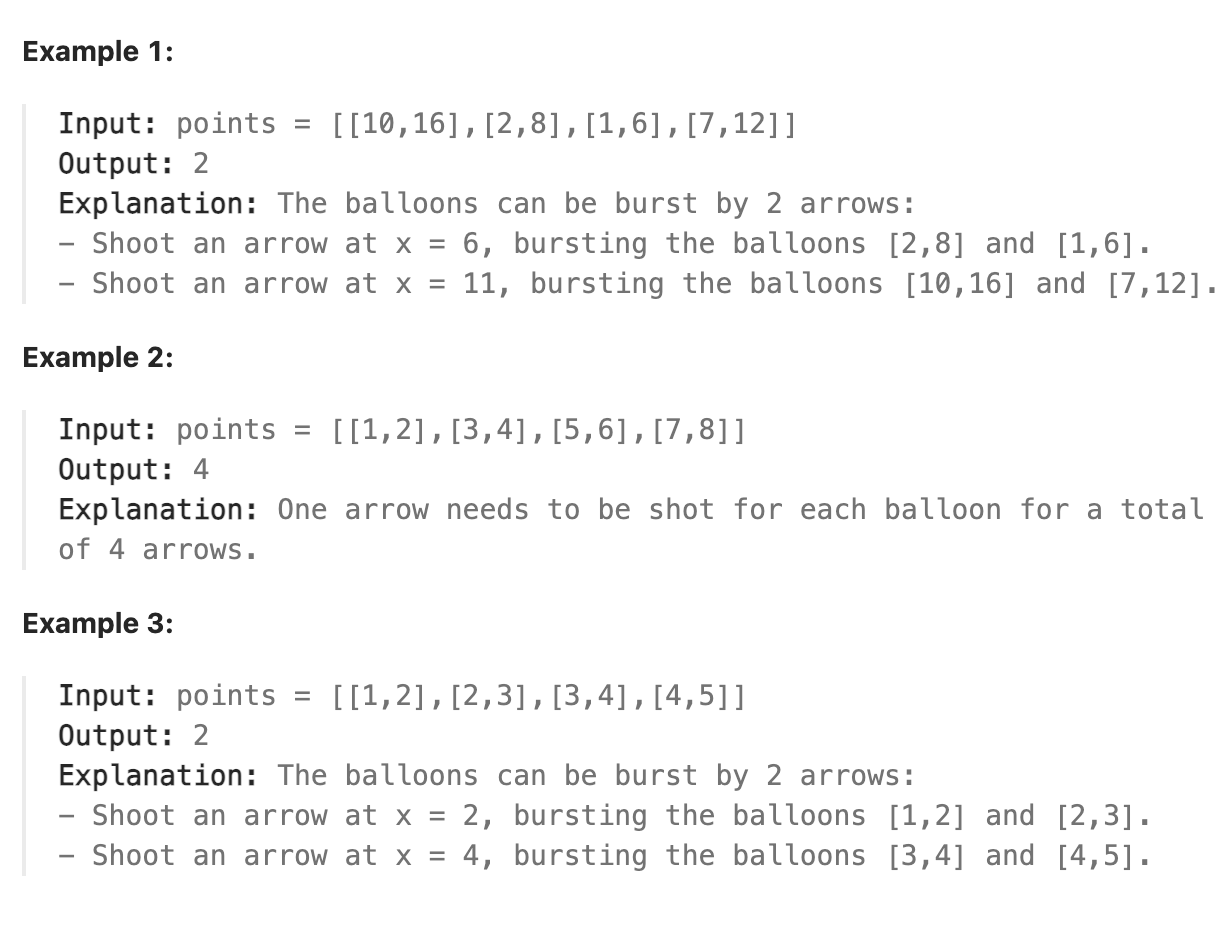
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题描述的很复杂, 其实是寻找相互交错的数组有几组, 如果将数组的范围看作集合, 范围重叠的数组可以看作一个大集合, 那么就是寻找这样的集合的数目. 本题可用贪心算法, 先将这些数组按照x_start的大小从小到大排序, 然后遍历并寻找x_start在当前重合范围内的数组,并且将重合范围设置为当前重合范围和当前遍历的数组的x_end中的较小值以缩小重合范围. 直到当前数组不满足条件, 即可认为之前的数组需要一个arrow. 继续遍历, 重复该操作, 即可找到所有需要的arrow.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
func findMinArrowShots(points [][]int) int {
|
||||||
|
sort.Slice(points, func (i, j int)bool{
|
||||||
|
if points[i][0]<points[j][0]{
|
||||||
|
return true
|
||||||
|
}else{
|
||||||
|
return false
|
||||||
|
}
|
||||||
|
})
|
||||||
|
arrow := 0
|
||||||
|
current := []int{points[0][0],points[0][1]}
|
||||||
|
for _, value := range points{
|
||||||
|
if value[0] <= current[1]{
|
||||||
|
if value[1] < current[1]{
|
||||||
|
current[1] = value[1]
|
||||||
|
}
|
||||||
|
current[0] = value[0]
|
||||||
|
}else{
|
||||||
|
arrow++
|
||||||
|
current[0] = value[0]
|
||||||
|
current[1] = value[1]
|
||||||
|
}
|
||||||
|
|
||||||
|
}
|
||||||
|
arrow++
|
||||||
|
return arrow
|
||||||
|
}
|
||||||
|
|
||||||
|
```
|
||||||
|
|
||||||
|
### 总结
|
||||||
|
|
||||||
|
本题的题目说明上有一些含糊, 根据题目说明, arrow只在竖直方向上移动, 也就是说, 必须要有重合的区间的数组才能用一个箭头, 假如有一个数组区间很大, 其和两个小区间重合但这两个小区间不重合, 按理来说应该使用两个arrow才能扎爆这三个气球, 但是看他人提交的代码中有如下一种代码, 似乎说明用一个arrow就可以. 究竟这种对不对有待进一步的思考. 代码如下
|
||||||
|
|
||||||
|
```go
|
||||||
|
func findMinArrowShots(points [][]int) int {
|
||||||
|
sort.Slice(points, func(i,j int) bool {
|
||||||
|
return points[i][1] < points[j][1]
|
||||||
|
})
|
||||||
|
res := 1
|
||||||
|
arrow := points[0][1]
|
||||||
|
for i := 0; i < len(points); i++ {
|
||||||
|
if arrow >= points[i][0] {continue}
|
||||||
|
res++
|
||||||
|
arrow = points[i][1]
|
||||||
|
}
|
||||||
|
return res
|
||||||
|
}
|
||||||
|
```
|
||||||
|
Loading…
Reference in New Issue
Block a user