mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-07-15 03:03:47 +08:00
leetcode update
This commit is contained in:
@ -5311,3 +5311,40 @@ func findRelativeRanks(score []int) []string {
|
||||
|
||||
}
|
||||
```
|
||||
|
||||
## day72 2024-05-09
|
||||
|
||||
### 3075. Maximize Happiness of Selected Children
|
||||
|
||||
You are given an array happiness of length n, and a positive integer k.
|
||||
|
||||
There are n children standing in a queue, where the ith child has happiness value happiness[i]. You want to select k children from these n children in k turns.
|
||||
|
||||
In each turn, when you select a child, the happiness value of all the children that have not been selected till now decreases by 1. Note that the happiness value cannot become negative and gets decremented only if it is positive.
|
||||
|
||||
Return the maximum sum of the happiness values of the selected children you can achieve by selecting k children.
|
||||
|
||||
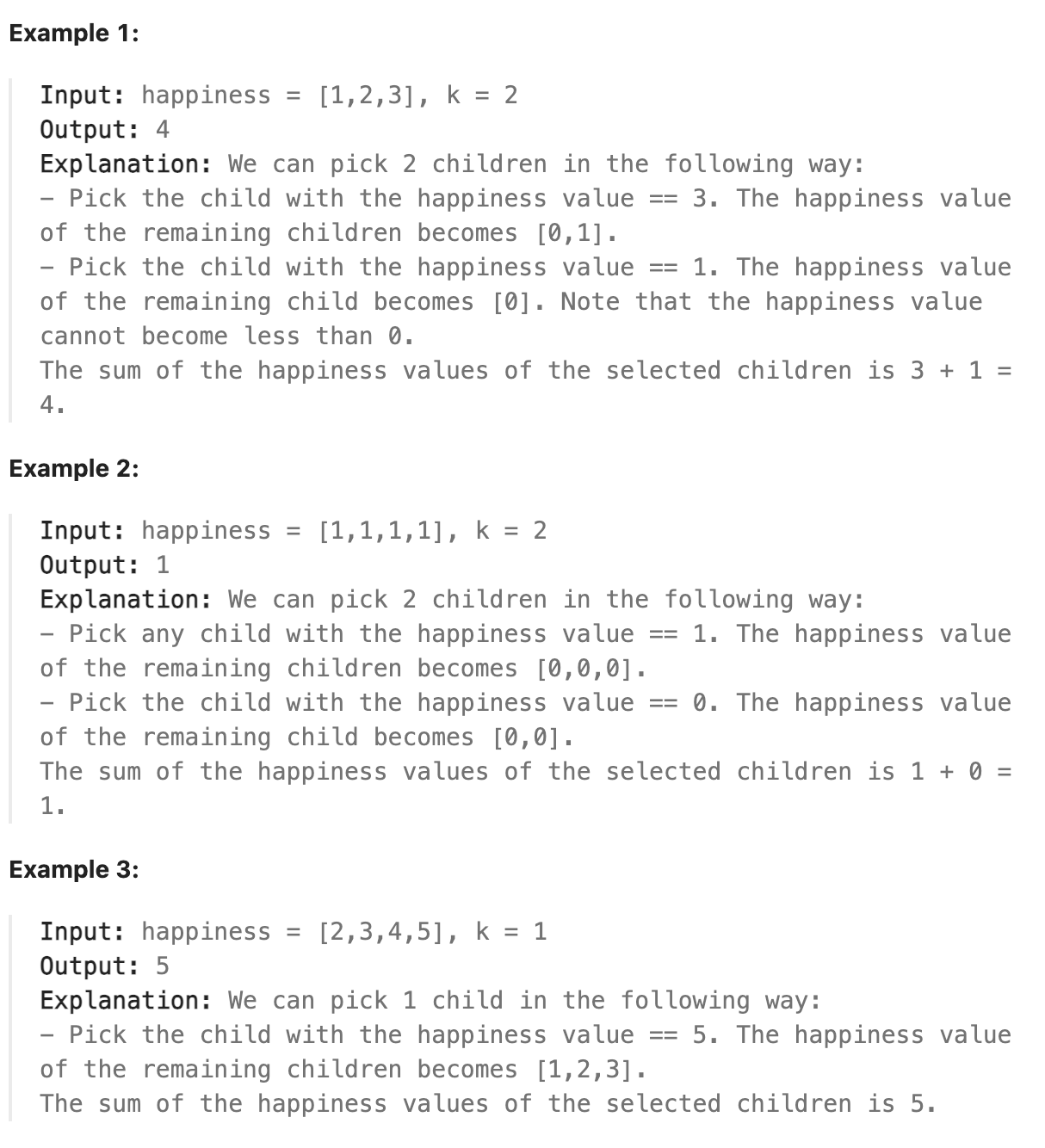
|
||||
|
||||
### 题解
|
||||
|
||||
本题思路上比较简单, 要求最大和, 将数组从大到小排序, 排序后依次取前k个元素加和即可, 注意处理每加一个元素, 下一个元素就要减掉目前已经加过的元素数量的值, 这可以通过数组下标来天然实现. 这类题目关键在于排序算法, 使用库中实现的排序算法一般都比较快. 但我们仍然要对常用的排序算法心中有数.
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
func maximumHappinessSum(happiness []int, k int) int64 {
|
||||
sort.Sort(sort.Reverse(sort.IntSlice(happiness)))
|
||||
result := 0
|
||||
temp := 0
|
||||
for index,value := range happiness{
|
||||
if index < k{
|
||||
temp = value - index
|
||||
if temp > 0{
|
||||
result += temp
|
||||
}
|
||||
}
|
||||
}
|
||||
return int64(result)
|
||||
}
|
||||
```
|
||||
|
Reference in New Issue
Block a user