mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-04-20 14:02:07 +08:00
leetcode update
This commit is contained in:
parent
cebaf32ea7
commit
637593df23
@ -6441,3 +6441,113 @@ func checkRecord(n int) int {
|
|||||||
return (before[0] + before[1] + before[2] + before[3] + before[4] + before[5]) % 1000000007
|
return (before[0] + before[1] + before[2] + before[3] + before[4] + before[5]) % 1000000007
|
||||||
}
|
}
|
||||||
```
|
```
|
||||||
|
|
||||||
|
## day91 2024-05-27
|
||||||
|
|
||||||
|
### 1608. Special Array With X Elements Greater Than or Equal X
|
||||||
|
|
||||||
|
You are given an array nums of non-negative integers. nums is considered special if there exists a number x such that there are exactly x numbers in nums that are greater than or equal to x.
|
||||||
|
|
||||||
|
Notice that x does not have to be an element in nums.
|
||||||
|
|
||||||
|
Return x if the array is special, otherwise, return -1. It can be proven that if nums is special, the value for x is unique.
|
||||||
|
|
||||||
|
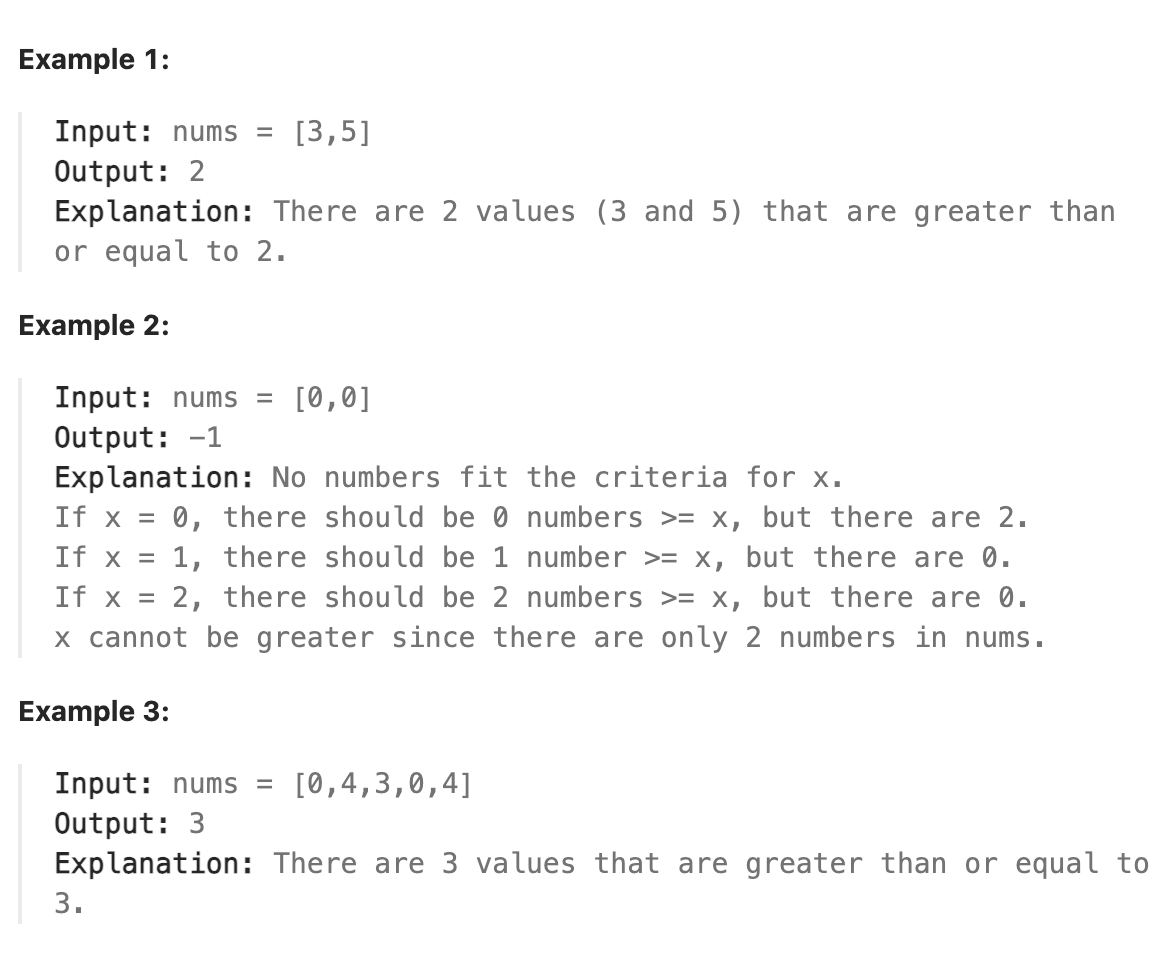
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
最简单的思路就是找到数组中的最大值, 再从最大值开始向下遍历各个整数, 并遍历数组查看是否满足条件. 但显然这样做时间复杂度很高. 如果先将数组排序, 则任一下标处的数后面的数必然大于等于这个数本身, 此时就可以用到数组末尾的距离来表示大于等于这个数的个数. 此时可以从后到前依次遍历并将当前下标到末尾的距离设为x, 并判断x是否大于前面的数, 如果大于则x满足条件. 对于这样在有序数组中依次遍历查找满足条件的x的值的问题都可以用二分法来加速解决. 设初始start为0, end为数组末尾下标, mid为二者和的一半. 因为题目中要求大于等于x的数的个数为x, 可以假设mid到数组末尾的距离为x, 判断这个数前面的数是否小于x并且当前mid下标对应的数大于等于x即可. 如果都满足则x为所求, 否则根据条件更新start或end的值.
|
||||||
|
|
||||||
|
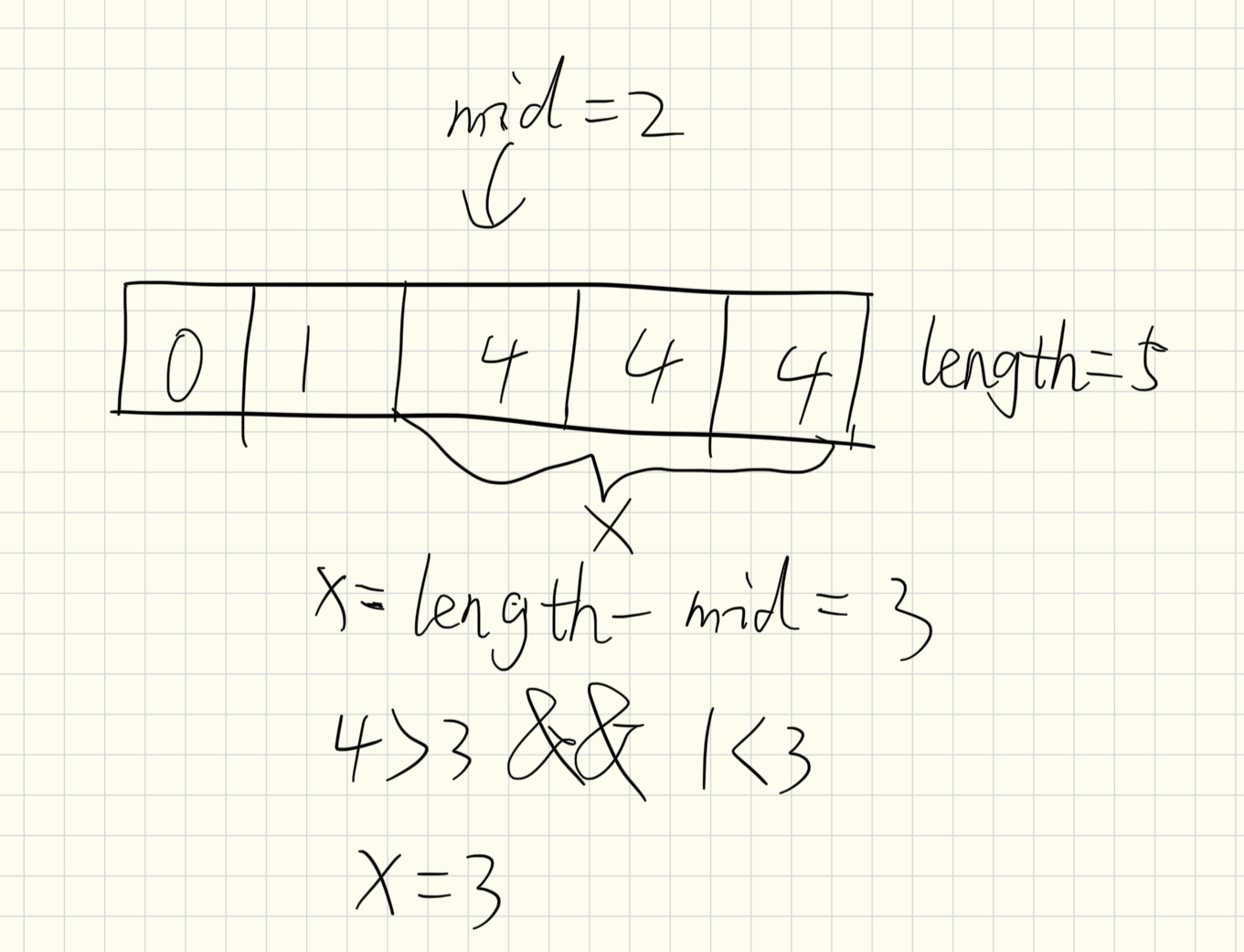
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
func specialArray(nums []int) int {
|
||||||
|
sort.Ints(nums)
|
||||||
|
length := len(nums)
|
||||||
|
start := 0
|
||||||
|
end := length - 1
|
||||||
|
mid := 0
|
||||||
|
x := 0
|
||||||
|
last := 0
|
||||||
|
for start <= end{
|
||||||
|
mid = (start + end) / 2
|
||||||
|
x = length - mid
|
||||||
|
if mid == 0{
|
||||||
|
last = 0
|
||||||
|
}else{
|
||||||
|
last = nums[mid-1]
|
||||||
|
}
|
||||||
|
if x <= nums[mid] && x > last{
|
||||||
|
return x
|
||||||
|
}else if x > nums[mid]{
|
||||||
|
start = mid + 1
|
||||||
|
}else if x <= last{
|
||||||
|
end = mid - 1
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return -1
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
## day92 2024-05-28
|
||||||
|
|
||||||
|
### 1208. Get Equal Substrings Within Budget
|
||||||
|
|
||||||
|
You are given two strings s and t of the same length and an integer maxCost.
|
||||||
|
|
||||||
|
You want to change s to t. Changing the ith character of s to ith character of t costs |s[i] - t[i]| (i.e., the absolute difference between the ASCII values of the characters).
|
||||||
|
|
||||||
|
Return the maximum length of a substring of s that can be changed to be the same as the corresponding substring of t with a cost less than or equal to maxCost. If there is no substring from s that can be changed to its corresponding substring from t, return 0.
|
||||||
|
|
||||||
|
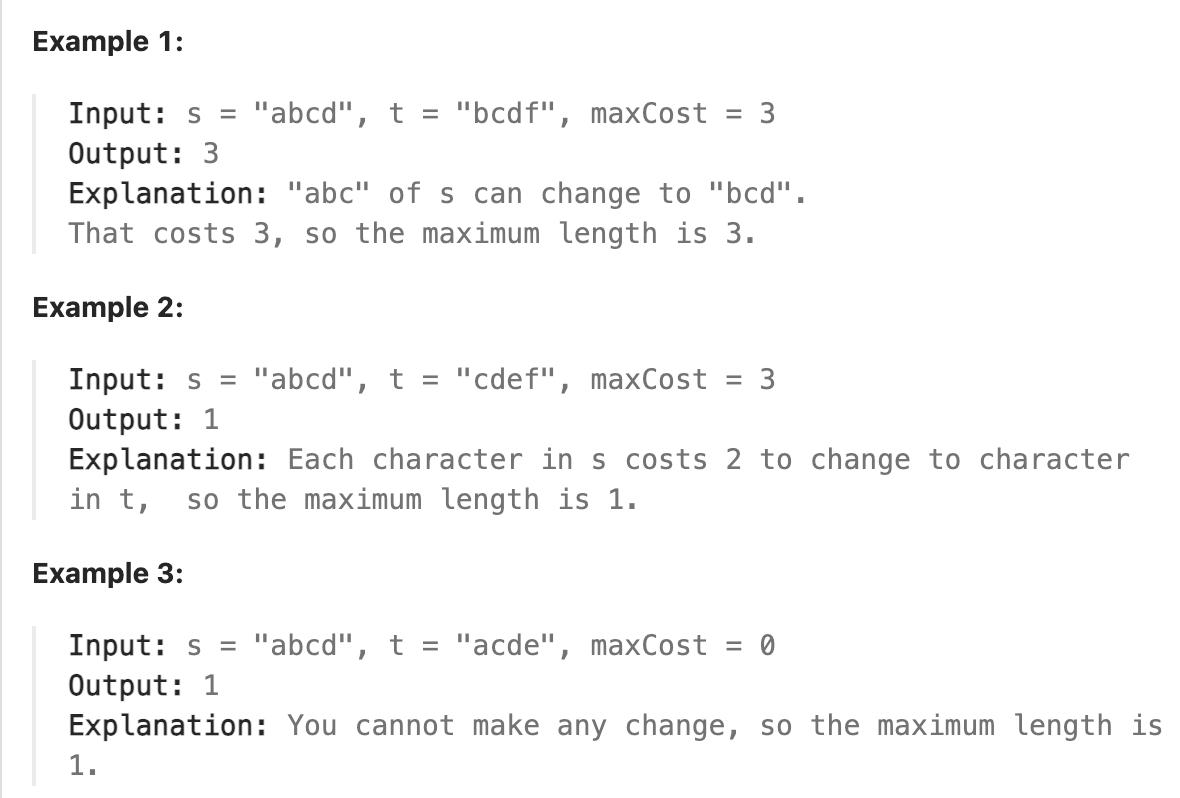
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题先计算出两个字符串各个位上的差值, 再使用滑动窗口找到窗口内和小于maxCost的最长窗口即可.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
func equalSubstring(s string, t string, maxCost int) int {
|
||||||
|
differs := []int{}
|
||||||
|
length := len(s)
|
||||||
|
differ := 0
|
||||||
|
for i,_ := range s{
|
||||||
|
differ = abs(s[i], t[i])
|
||||||
|
differs = append(differs, differ)
|
||||||
|
}
|
||||||
|
fmt.Println(differs)
|
||||||
|
|
||||||
|
begin := 0
|
||||||
|
end := 0
|
||||||
|
cost := 0
|
||||||
|
result := 0
|
||||||
|
for end < length{
|
||||||
|
// fmt.Println(end)
|
||||||
|
cost += differs[end]
|
||||||
|
if cost <= maxCost{
|
||||||
|
result = max(result, end-begin+1)
|
||||||
|
}else{
|
||||||
|
for begin<=end{
|
||||||
|
cost -= differs[begin]
|
||||||
|
begin++
|
||||||
|
if cost <= maxCost{
|
||||||
|
break
|
||||||
|
}
|
||||||
|
}
|
||||||
|
}
|
||||||
|
end++
|
||||||
|
}
|
||||||
|
return result
|
||||||
|
}
|
||||||
|
|
||||||
|
func abs(i byte, j byte)int{
|
||||||
|
if i > j{
|
||||||
|
return int(i - j)
|
||||||
|
}else{
|
||||||
|
return int(j - i)
|
||||||
|
}
|
||||||
|
}
|
||||||
|
```
|
||||||
|
Loading…
Reference in New Issue
Block a user