mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-06-20 20:22:07 +08:00
leetcode update
This commit is contained in:
parent
9978891974
commit
5b9f2d1723
@ -1351,3 +1351,115 @@ func findMaxLength(nums []int) int {
|
||||
### 总结
|
||||
|
||||
本题和前几天的题目在思想上有异曲同工之妙, 也是前缀和的一种应用, 如果把0和1的数量差作为前缀和, 那么本题解题思路可简单概括为找到前缀和相等的位置的最远距离.
|
||||
|
||||
## day20 2024-03-17
|
||||
|
||||
### 57. Insert Interval
|
||||
|
||||
You are given an array of non-overlapping intervals intervals where intervals[i] = [starti, endi] represent the start and the end of the ith interval and intervals is sorted in ascending order by starti. You are also given an interval newInterval = [start, end] that represents the start and end of another interval.
|
||||
|
||||
Insert newInterval into intervals such that intervals is still sorted in ascending order by starti and intervals still does not have any overlapping intervals (merge overlapping intervals if necessary).
|
||||
|
||||
Return intervals after the insertion.
|
||||
|
||||
Note that you don't need to modify intervals in-place. You can make a new array and return it.
|
||||
|
||||
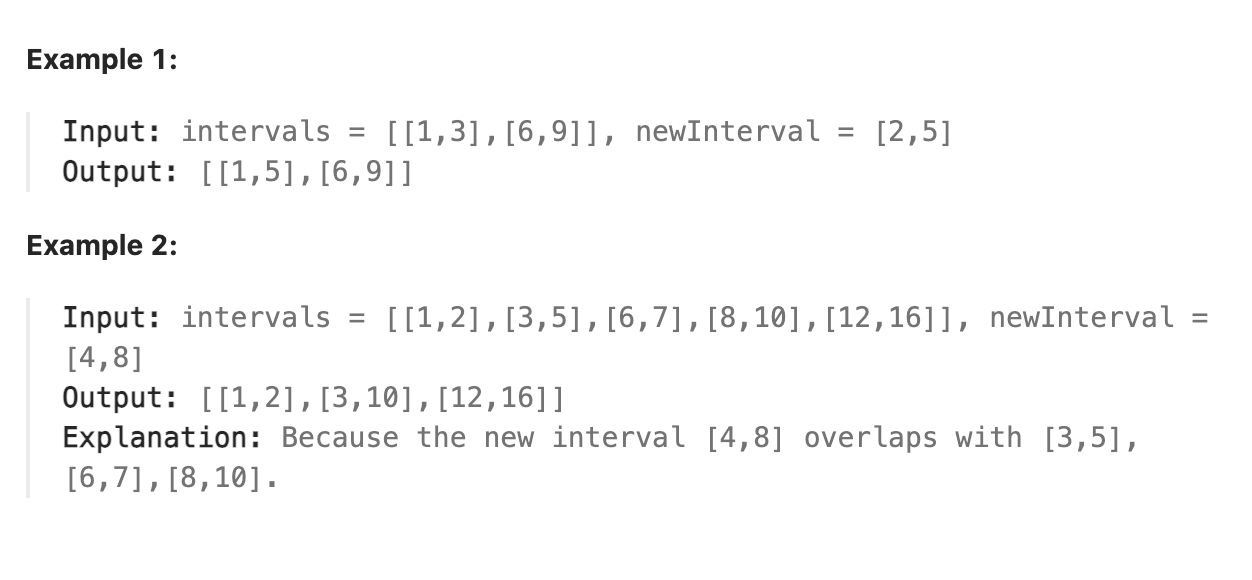
|
||||
|
||||
### 题解
|
||||
|
||||
遍历intervals, 将interval复制到结果数组中. 判断要插入的interval的start和end是否在当前interval中
|
||||
|
||||
1. 在范围内则将新interval的start设置为当前interval的start. 使用相同的方式判断end的范围.
|
||||
2. 若start或end不在当前interval范围内且小于下一个interval的start, 则将start或者end设置为新interval的start或end. 此时新interval构造完成, 将后面的interval原样复制到result中即可.
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
func insert(intervals [][]int, newInterval []int) [][]int {
|
||||
result := [][]int{}
|
||||
start := newInterval[0]
|
||||
end := newInterval[1]
|
||||
insertInterval := []int{}
|
||||
flag := 0
|
||||
for _, value := range intervals{
|
||||
if flag == 2{
|
||||
result = append(result, value)
|
||||
}else if flag == 0{
|
||||
if start < value[0]{
|
||||
insertInterval = append(insertInterval, start)
|
||||
flag++
|
||||
if end < value[0]{
|
||||
insertInterval = append(insertInterval, end)
|
||||
flag++
|
||||
result = append(result, insertInterval)
|
||||
result = append(result, value)
|
||||
}else if end >= value[0] && end <= value[1]{
|
||||
insertInterval = append(insertInterval, value[1])
|
||||
flag++
|
||||
result = append(result, insertInterval)
|
||||
}
|
||||
}else if start >= value[0] && start <= value[1]{
|
||||
insertInterval = append(insertInterval, value[0])
|
||||
flag++
|
||||
if end >= value[0] && end <= value[1]{
|
||||
insertInterval = append(insertInterval, value[1])
|
||||
flag++
|
||||
result = append(result, insertInterval)
|
||||
}
|
||||
}else{
|
||||
result = append(result, value)
|
||||
}
|
||||
}else{
|
||||
if end < value[0]{
|
||||
insertInterval = append(insertInterval, end)
|
||||
flag++
|
||||
result = append(result, insertInterval)
|
||||
result = append(result, value)
|
||||
}else if end >= value[0] && end <= value[1]{
|
||||
insertInterval = append(insertInterval, value[1])
|
||||
flag++
|
||||
result = append(result, insertInterval)
|
||||
}
|
||||
}
|
||||
}
|
||||
if flag == 0{
|
||||
result = append(result, []int{start, end})
|
||||
}else if flag == 1{
|
||||
insertInterval = append(insertInterval, end)
|
||||
result = append(result, insertInterval)
|
||||
}
|
||||
return result
|
||||
}
|
||||
```
|
||||
|
||||
### 总结
|
||||
|
||||
这种题思路上并没有特别之处, 但是判断逻辑比较繁琐, 需要耐心思考边界情况, 查看他人题解, 发现用原始数组与需要插入的interval做比较要比使用interval和原始数组的start和end做比较思路简单清晰得多, 这里还是对判断条件的变与不变理解的不够透彻, 需要插入的interval的start和end是不变的, 不断遍历原始数组与不变的start和end做比较要比使用不变的start和end去和不断变化的interval的start和end做比较判断起来容易得多. 找到不变的条件对于思路清楚的解决问题至关重要. 给出示例代码
|
||||
|
||||
```go
|
||||
func insert(intervals [][]int, newInterval []int) [][]int {
|
||||
|
||||
res := make([][]int, 0)
|
||||
|
||||
i := 0
|
||||
|
||||
for ; i < len(intervals) && intervals[i][1] < newInterval[0]; i++ {
|
||||
res = append(res, intervals[i])
|
||||
}
|
||||
|
||||
for ; i < len(intervals) && intervals[i][0] <= newInterval[1]; i++ {
|
||||
newInterval[0] = min(intervals[i][0], newInterval[0])
|
||||
newInterval[1] = max(intervals[i][1], newInterval[1])
|
||||
}
|
||||
|
||||
res = append(res, newInterval)
|
||||
|
||||
for i < len(intervals) {
|
||||
res = append(res, intervals[i])
|
||||
i++
|
||||
}
|
||||
|
||||
return res
|
||||
}
|
||||
```
|
||||
|
Loading…
Reference in New Issue
Block a user