mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-04-20 05:52:07 +08:00
leetcode update
This commit is contained in:
parent
1b0e809b5f
commit
559b0f9f62
@ -16908,3 +16908,90 @@ public:
|
|||||||
}
|
}
|
||||||
};
|
};
|
||||||
```
|
```
|
||||||
|
|
||||||
|
## day239 2024-11-02
|
||||||
|
|
||||||
|
### 2490. Circular Sentence
|
||||||
|
|
||||||
|
A sentence is a list of words that are separated by a single space with no leading or trailing spaces.
|
||||||
|
|
||||||
|
For example, "Hello World", "HELLO", "hello world hello world" are all sentences.
|
||||||
|
Words consist of only uppercase and lowercase English letters. Uppercase and lowercase English letters are considered different.
|
||||||
|
|
||||||
|
A sentence is circular if:
|
||||||
|
|
||||||
|
The last character of a word is equal to the first character of the next word.
|
||||||
|
The last character of the last word is equal to the first character of the first word.
|
||||||
|
For example, "leetcode exercises sound delightful", "eetcode", "leetcode eats soul" are all circular sentences. However, "Leetcode is cool", "happy Leetcode", "Leetcode" and "I like Leetcode" are not circular sentences.
|
||||||
|
|
||||||
|
Given a string sentence, return true if it is circular. Otherwise, return false.
|
||||||
|
|
||||||
|
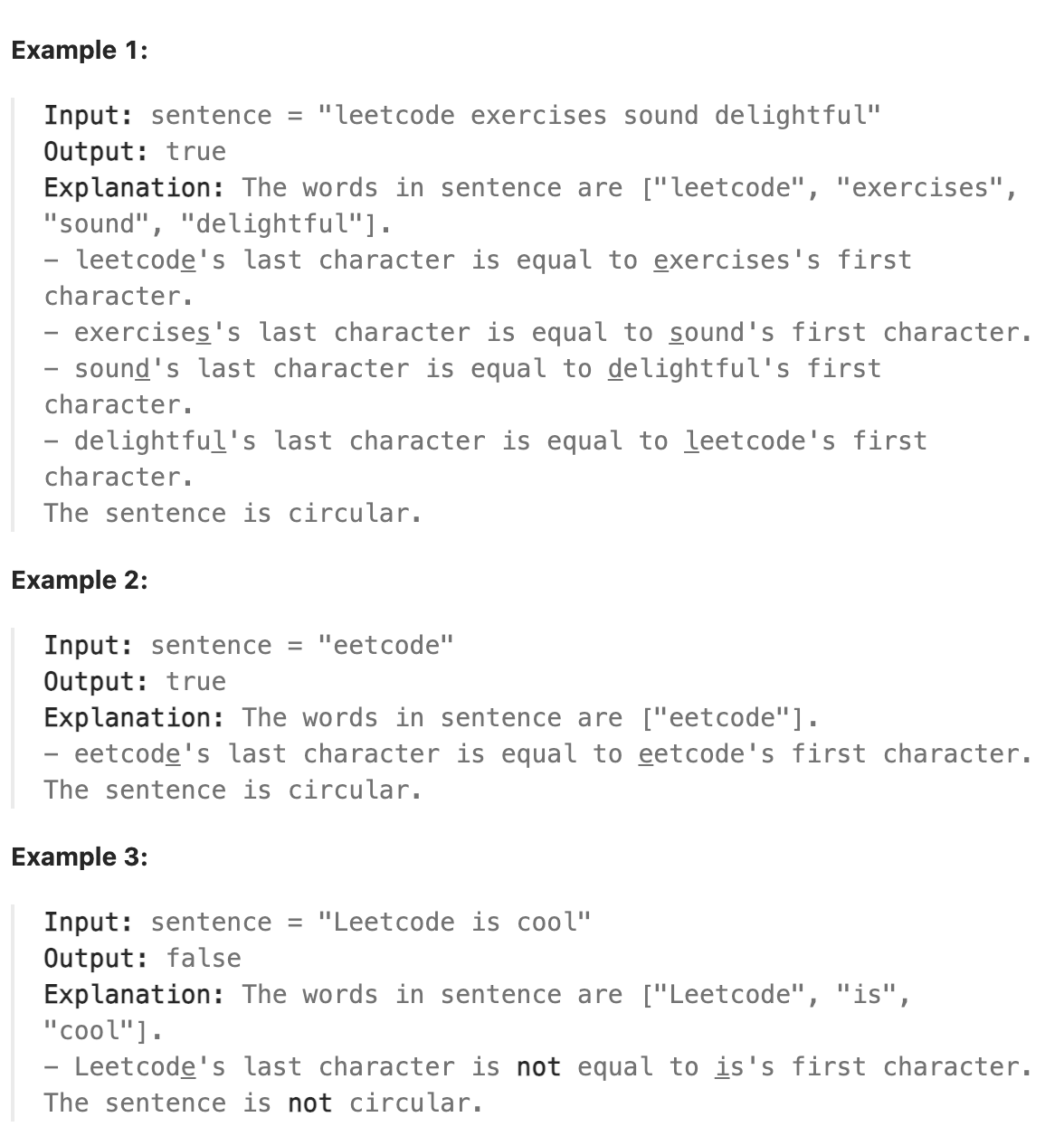
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题是一道简单题,先判断句子的首尾字符是否相同,不同直接返回false。再遍历句子,每当下一个字符为空格时,考虑到题目中明确说明单词和单词之间只有一个空格分隔,则判断当前字符和下下个字符是否相等,不相等直接返回false。遍历完成则返回true。
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```cpp
|
||||||
|
class Solution {
|
||||||
|
public:
|
||||||
|
bool isCircularSentence(string sentence) {
|
||||||
|
if(sentence[0] != sentence[sentence.size()-1]){
|
||||||
|
return false;
|
||||||
|
}
|
||||||
|
for (int i=0;i<sentence.size()-1;i++){
|
||||||
|
if (sentence[i+1] == ' ' && sentence[i+2] != sentence[i]){
|
||||||
|
return false;
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return true;
|
||||||
|
}
|
||||||
|
};
|
||||||
|
```
|
||||||
|
|
||||||
|
## day240 2024-11-03
|
||||||
|
|
||||||
|
### 796. Rotate String
|
||||||
|
|
||||||
|
Given two strings s and goal, return true if and only if s can become goal after some number of shifts on s.
|
||||||
|
|
||||||
|
A shift on s consists of moving the leftmost character of s to the rightmost position.
|
||||||
|
|
||||||
|
For example, if s = "abcde", then it will be "bcdea" after one shift.
|
||||||
|
|
||||||
|
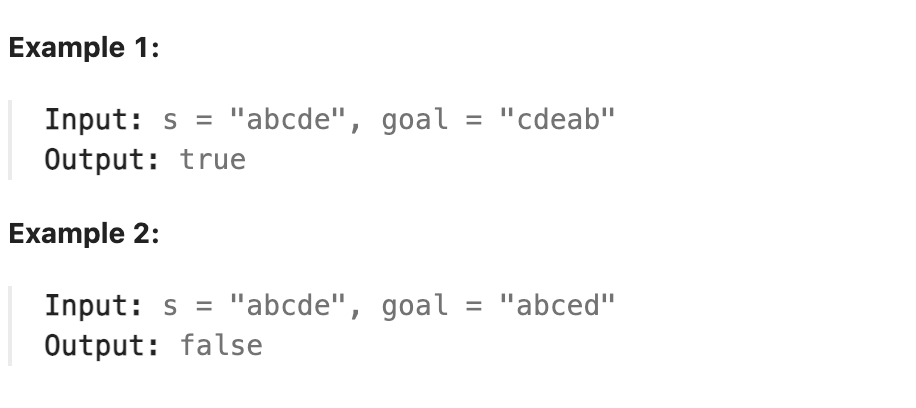
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题是一道简单题,一个比较容易想到的思路是对于s,选定开头字母如字母a作为整个循环字符串的起始,随后遍历goal字符串,遇到字符a就将其当作s的开头字符a,向后遍历goal字符串并与s比对,成功则为true,失败则继续遍历goal寻找下一个a,直到遍历完整个goal为止。
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```cpp
|
||||||
|
class Solution {
|
||||||
|
public:
|
||||||
|
bool rotateString(string s, string goal) {
|
||||||
|
if (s.size() != goal.size()){
|
||||||
|
return false;
|
||||||
|
}
|
||||||
|
char label = s[0];
|
||||||
|
for (int i=0;i<goal.size();i++){
|
||||||
|
if (goal[i] == label){
|
||||||
|
bool success = true;
|
||||||
|
for (int j=0;j<s.size();j++){
|
||||||
|
if(s[j] != goal[(i+j)%s.size()]){
|
||||||
|
success = false;
|
||||||
|
break;
|
||||||
|
}
|
||||||
|
}
|
||||||
|
if (success){
|
||||||
|
return true;
|
||||||
|
}
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return false;
|
||||||
|
}
|
||||||
|
};
|
||||||
|
```
|
||||||
|
Loading…
Reference in New Issue
Block a user