mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-07-04 05:32:07 +08:00
leetcode update
This commit is contained in:
parent
559b0f9f62
commit
5015901f8e
@ -16995,3 +16995,100 @@ public:
|
|||||||
}
|
}
|
||||||
};
|
};
|
||||||
```
|
```
|
||||||
|
|
||||||
|
## day241 2024-11-04
|
||||||
|
|
||||||
|
### 3163. String Compression III
|
||||||
|
|
||||||
|
Given a string word, compress it using the following algorithm:
|
||||||
|
|
||||||
|
Begin with an empty string comp. While word is not empty, use the following operation:
|
||||||
|
Remove a maximum length prefix of word made of a single character c repeating at most 9 times.
|
||||||
|
Append the length of the prefix followed by c to comp.
|
||||||
|
Return the string comp.
|
||||||
|
|
||||||
|
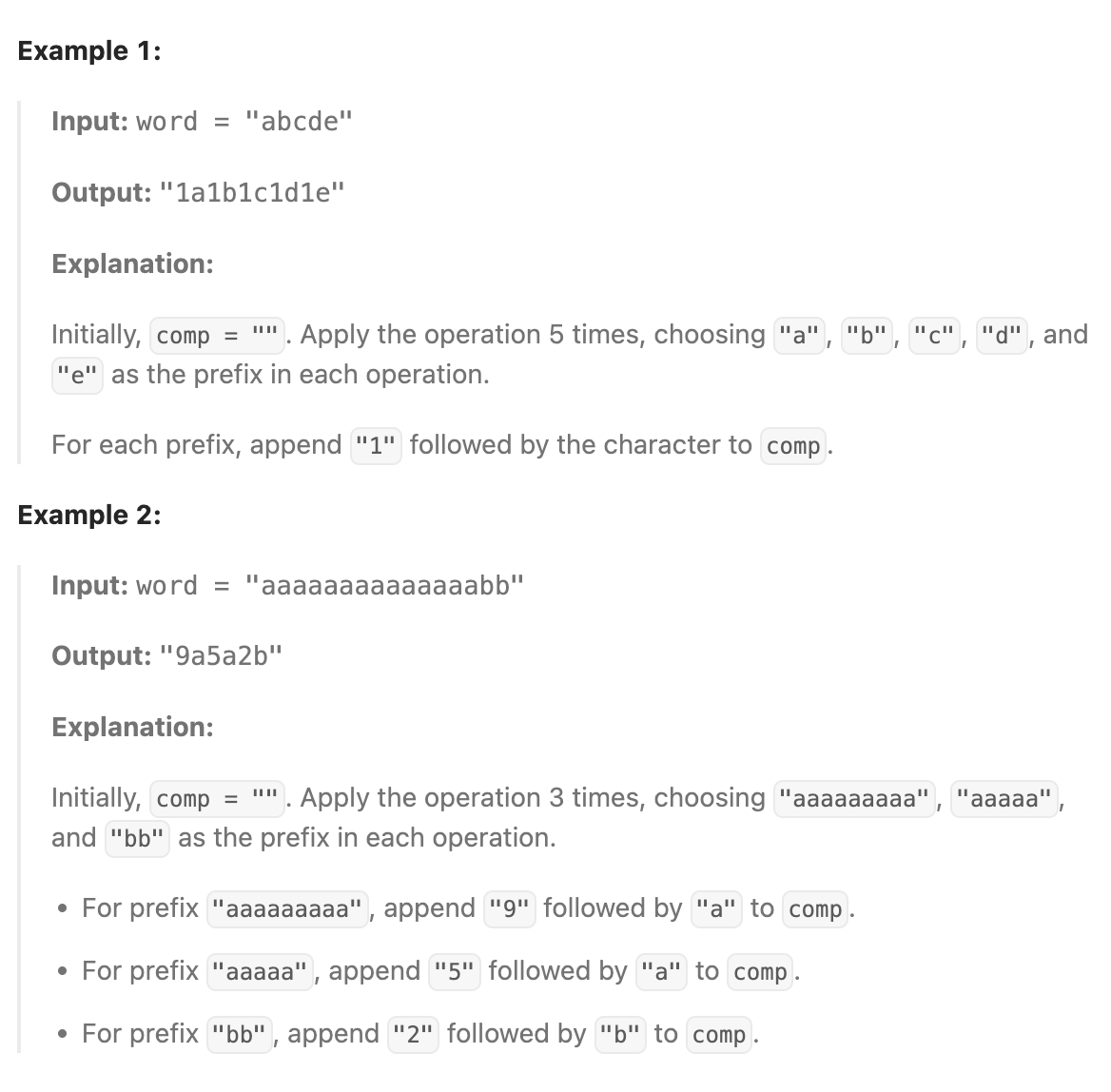
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题读懂题意后其实并不难,就是一个单纯的字符串统计,从头遍历字符串,统计当前重复出现的字符个数,当个数达到9个时或者遇到新字符时就向comp字符串末尾添加当前重复字符的重复次数和字符本身。
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```cpp
|
||||||
|
class Solution {
|
||||||
|
public:
|
||||||
|
string compressedString(string word) {
|
||||||
|
char num = '0';
|
||||||
|
char current = word[0];
|
||||||
|
string result;
|
||||||
|
for(auto ch:word){
|
||||||
|
if (ch == current){
|
||||||
|
if (num == '9'){
|
||||||
|
result.push_back(num);
|
||||||
|
result.push_back(current);
|
||||||
|
num = '1';
|
||||||
|
continue;
|
||||||
|
}
|
||||||
|
num++;
|
||||||
|
}else if(ch != current){
|
||||||
|
result.push_back(num);
|
||||||
|
result.push_back(current);
|
||||||
|
num = '1';
|
||||||
|
current = ch;
|
||||||
|
}
|
||||||
|
}
|
||||||
|
result.push_back(num);
|
||||||
|
result.push_back(current);
|
||||||
|
|
||||||
|
return result;
|
||||||
|
}
|
||||||
|
};
|
||||||
|
```
|
||||||
|
|
||||||
|
## day242 2024-11-05
|
||||||
|
|
||||||
|
### 2914. Minimum Number of Changes to Make Binary String Beautiful
|
||||||
|
|
||||||
|
You are given a 0-indexed binary string s having an even length.
|
||||||
|
|
||||||
|
A string is beautiful if it's possible to partition it into one or more substrings such that:
|
||||||
|
|
||||||
|
Each substring has an even length.
|
||||||
|
Each substring contains only 1's or only 0's.
|
||||||
|
You can change any character in s to 0 or 1.
|
||||||
|
|
||||||
|
Return the minimum number of changes required to make the string s beautiful.
|
||||||
|
|
||||||
|
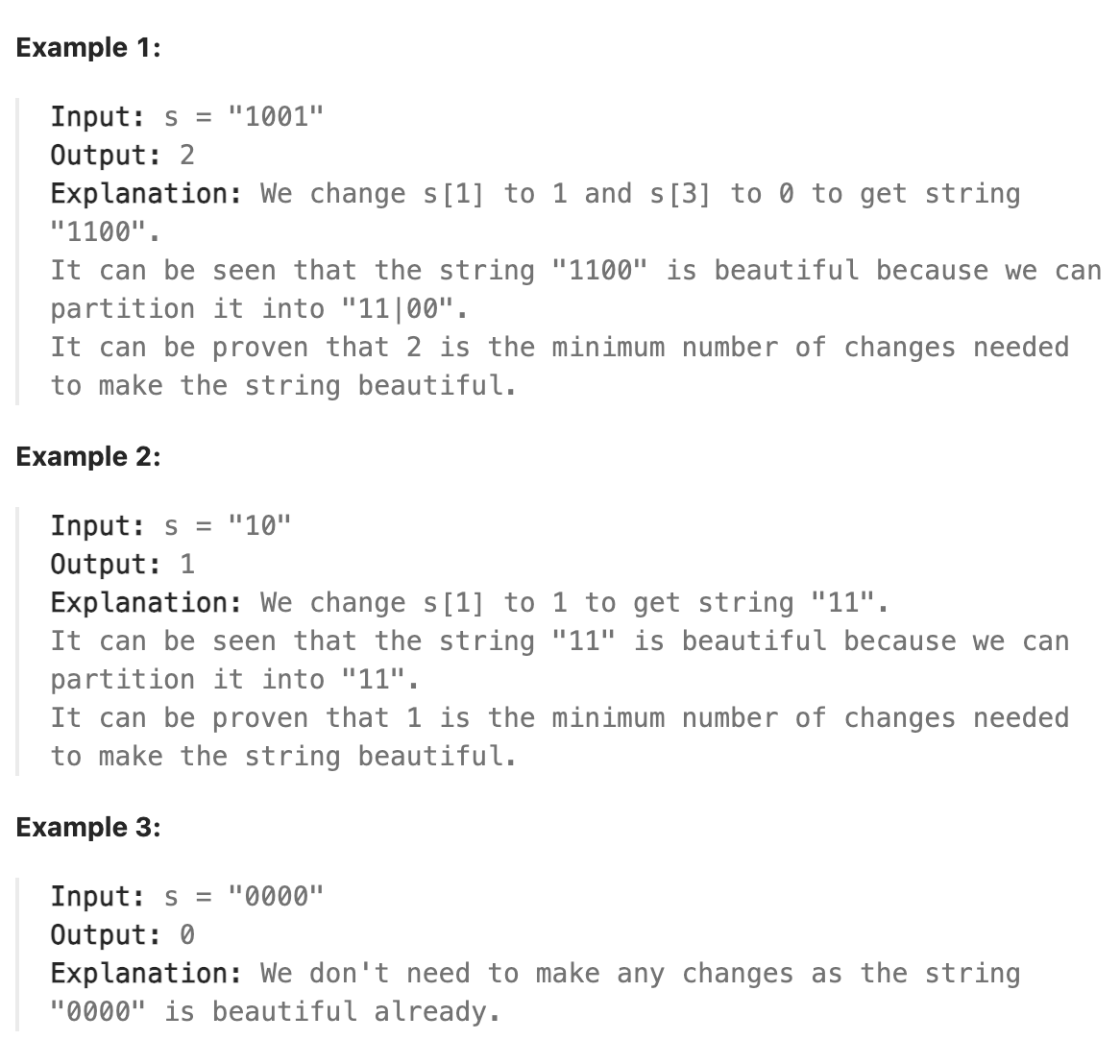
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题可使用贪心,考虑到只要求每个分割出来的偶数长度字符串中只包含0或者1,且字符串的总长为偶数。则只需记录当前连续出现的字符是0还是1并记录该字符连续出现的次数,遇到另外一个字符时,如果当前出现的次数为奇数则将另外一个字符变为当前字符,将次数+1变为偶数,继续向后计数。若遇到另外一个字符时当前出现次数为偶数则改变当前记录字符并从头开始计数。如此反复直到达到字符串结尾。
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```cpp
|
||||||
|
class Solution {
|
||||||
|
public:
|
||||||
|
int minChanges(string s) {
|
||||||
|
int count = 0;
|
||||||
|
char current = s[0];
|
||||||
|
int result = 0;
|
||||||
|
for (char ch : s){
|
||||||
|
if (current == ch){
|
||||||
|
count++;
|
||||||
|
}else{
|
||||||
|
if (count % 2 == 1){
|
||||||
|
result++;
|
||||||
|
count++;
|
||||||
|
}else{
|
||||||
|
count = 1;
|
||||||
|
current = ch;
|
||||||
|
}
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return result;
|
||||||
|
}
|
||||||
|
};
|
||||||
|
```
|
||||||
|
Loading…
Reference in New Issue
Block a user