mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-06-07 20:02:08 +08:00
leetcode update
This commit is contained in:
parent
187501c063
commit
4b5e5c64ab
@ -7982,3 +7982,56 @@ func minKBitFlips(nums []int, k int) int {
|
|||||||
return -1
|
return -1
|
||||||
}
|
}
|
||||||
```
|
```
|
||||||
|
|
||||||
|
## day120 2024-06-25
|
||||||
|
### 1038. Binary Search Tree to Greater Sum Tree
|
||||||
|
|
||||||
|
Given the root of a Binary Search Tree (BST), convert it to a Greater Tree such that every key of the original BST is changed to the original key plus the sum of all keys greater than the original key in BST.
|
||||||
|
|
||||||
|
As a reminder, a binary search tree is a tree that satisfies these constraints:
|
||||||
|
|
||||||
|
The left subtree of a node contains only nodes with keys less than the node's key.
|
||||||
|
The right subtree of a node contains only nodes with keys greater than the node's key.
|
||||||
|
Both the left and right subtrees must also be binary search trees.
|
||||||
|
|
||||||
|
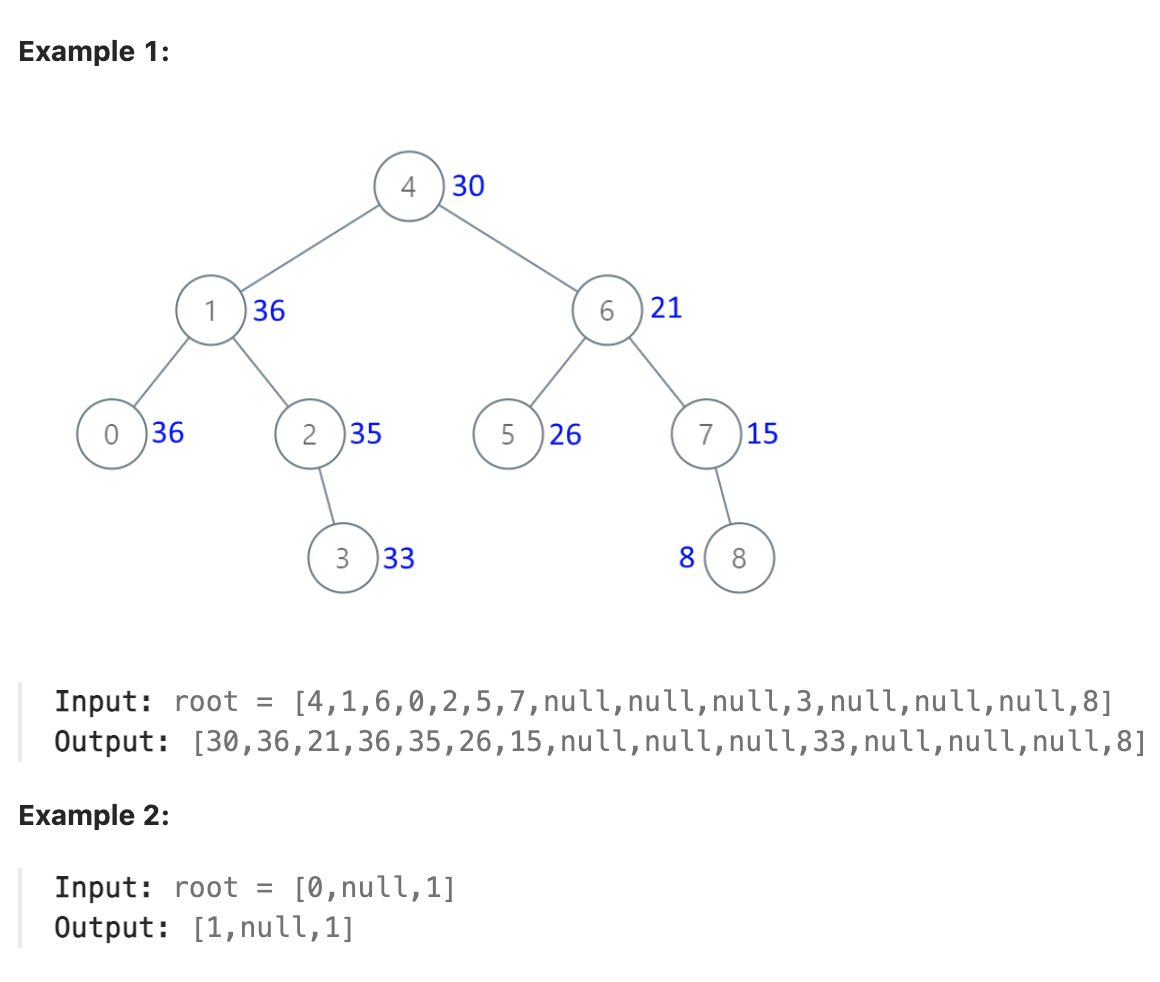
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
考虑到树为一棵二叉搜索树, 因此对于任何一个节点, 比它大的节点有其右子树的节点, 若其父节点为祖父节点的左子节点, 则还有其祖父节点以及祖父节点的右子树的节点. 以此类推. 考虑最简单的仅有三个节点的情况: 一个父节点和左右两个子节点. 对于左子节点而言, 需要加父节点和右子节点的值. 对于父节点要加右子节点的值. 右子节点不用处理. 因此通过对树遍历将问题转化为类似三个节点的情况. 左右子树均可视为一个节点. 从根节点开始, 对节点进行如下操作, 递归遍历右子树, 计算右子树所有节点的和返回. 将当前节点的值加上右子树的和, 将和传递给左子树并递归遍历左子树, 返回左子树所有节点的和. 最终返回根节点和左右两个子树的和. 注意更新节点值和计算子树和是分开的, 更新节点值要将传递的值和当前值以及右子树的和相加. 但返回时返回的是原来的节点值对应的子树和.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
```go
|
||||||
|
/**
|
||||||
|
* Definition for a binary tree node.
|
||||||
|
* type TreeNode struct {
|
||||||
|
* Val int
|
||||||
|
* Left *TreeNode
|
||||||
|
* Right *TreeNode
|
||||||
|
* }
|
||||||
|
*/
|
||||||
|
func bstToGst(root *TreeNode) *TreeNode {
|
||||||
|
rightFirst(root, 0)
|
||||||
|
return root
|
||||||
|
}
|
||||||
|
|
||||||
|
func rightFirst(root *TreeNode, pass int)int{
|
||||||
|
temp := root.Val
|
||||||
|
if root.Left == nil && root.Right == nil{
|
||||||
|
root.Val += pass
|
||||||
|
return temp
|
||||||
|
}
|
||||||
|
rightSum := 0
|
||||||
|
if root.Right != nil{
|
||||||
|
rightSum = rightFirst(root.Right, pass)
|
||||||
|
}
|
||||||
|
leftSum := 0
|
||||||
|
if root.Left != nil{
|
||||||
|
leftSum = rightFirst(root.Left, rightSum+temp+pass)
|
||||||
|
}
|
||||||
|
root.Val = root.Val + pass + rightSum
|
||||||
|
return temp + rightSum + leftSum
|
||||||
|
}
|
||||||
|
|
||||||
|
```
|
||||||
|
|
||||||
|
Loading…
Reference in New Issue
Block a user