mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-06-07 11:52:07 +08:00
leetcode update
This commit is contained in:
parent
c6ad845913
commit
4aa9d1532c
@ -23422,4 +23422,144 @@ public:
|
|||||||
};
|
};
|
||||||
```
|
```
|
||||||
|
|
||||||
|
## day348 2025-02-21
|
||||||
|
### 1261. Find Elements in a Contaminated Binary Tree
|
||||||
|
Given a binary tree with the following rules:
|
||||||
|
|
||||||
|
root.val == 0
|
||||||
|
For any treeNode:
|
||||||
|
If treeNode.val has a value x and treeNode.left != null, then treeNode.left.val == 2 * x + 1
|
||||||
|
If treeNode.val has a value x and treeNode.right != null, then treeNode.right.val == 2 * x + 2
|
||||||
|
Now the binary tree is contaminated, which means all treeNode.val have been changed to -1.
|
||||||
|
|
||||||
|
Implement the FindElements class:
|
||||||
|
|
||||||
|
FindElements(TreeNode* root) Initializes the object with a contaminated binary tree and recovers it.
|
||||||
|
bool find(int target) Returns true if the target value exists in the recovered binary tree.
|
||||||
|
|
||||||
|
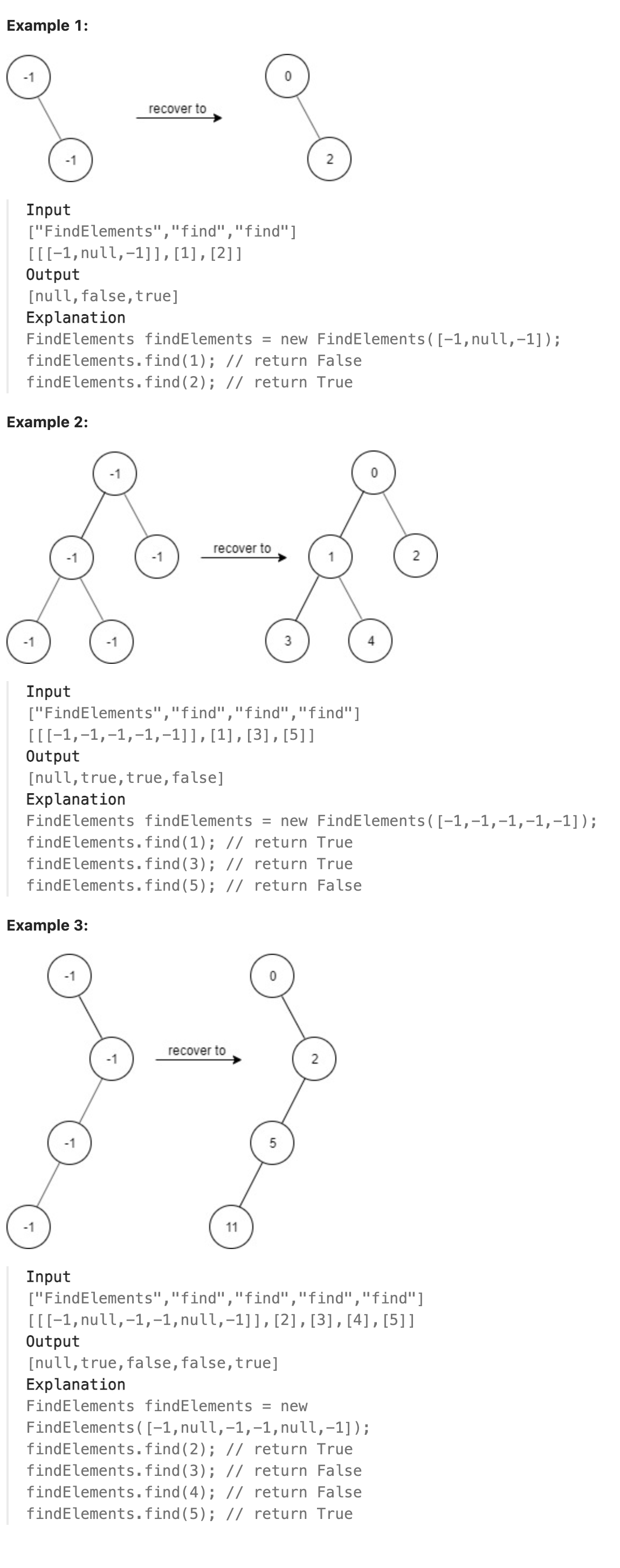
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
本题思路上并不困难,只需要执行bfs或者dfs(此处使用dfs)遍历树的同时按照对应的计算规则将每个节点的值计算出来并赋给节点,同时将值保存到一个哈希表中(无需排序因此使用unordered_map),对于find函数直接在哈希表中查找。
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
```cpp
|
||||||
|
/**
|
||||||
|
* Definition for a binary tree node.
|
||||||
|
* struct TreeNode {
|
||||||
|
* int val;
|
||||||
|
* TreeNode *left;
|
||||||
|
* TreeNode *right;
|
||||||
|
* TreeNode() : val(0), left(nullptr), right(nullptr) {}
|
||||||
|
* TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
|
||||||
|
* TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left), right(right) {}
|
||||||
|
* };
|
||||||
|
*/
|
||||||
|
class FindElements {
|
||||||
|
public:
|
||||||
|
FindElements(TreeNode* root) {
|
||||||
|
root->val = 0;
|
||||||
|
dfs(root);
|
||||||
|
}
|
||||||
|
|
||||||
|
void dfs(TreeNode* root){
|
||||||
|
findtable.insert(root->val);
|
||||||
|
if(root->left != nullptr){
|
||||||
|
root->left->val = root->val*2+1;
|
||||||
|
dfs(root->left);
|
||||||
|
}
|
||||||
|
if(root->right != nullptr){
|
||||||
|
root->right->val = root->val * 2 + 2;
|
||||||
|
|
||||||
|
dfs(root->right);
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
unordered_set<int> findtable;
|
||||||
|
bool find(int target) {
|
||||||
|
return findtable.find(target) != findtable.end();
|
||||||
|
}
|
||||||
|
};
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Your FindElements object will be instantiated and called as such:
|
||||||
|
* FindElements* obj = new FindElements(root);
|
||||||
|
* bool param_1 = obj->find(target);
|
||||||
|
*/
|
||||||
|
```
|
||||||
|
|
||||||
|
## day349 2025-02-22
|
||||||
|
### 1028. Recover a Tree From Preorder traversal
|
||||||
|
We run a preorder depth-first search (DFS) on the root of a binary tree.
|
||||||
|
|
||||||
|
At each node in this traversal, we output D dashes (where D is the depth of this node), then we output the value of this node. If the depth of a node is D, the depth of its immediate child is D + 1. The depth of the root node is 0.
|
||||||
|
|
||||||
|
If a node has only one child, that child is guaranteed to be the left child.
|
||||||
|
|
||||||
|
Given the output traversal of this traversal, recover the tree and return its root.
|
||||||
|
|
||||||
|
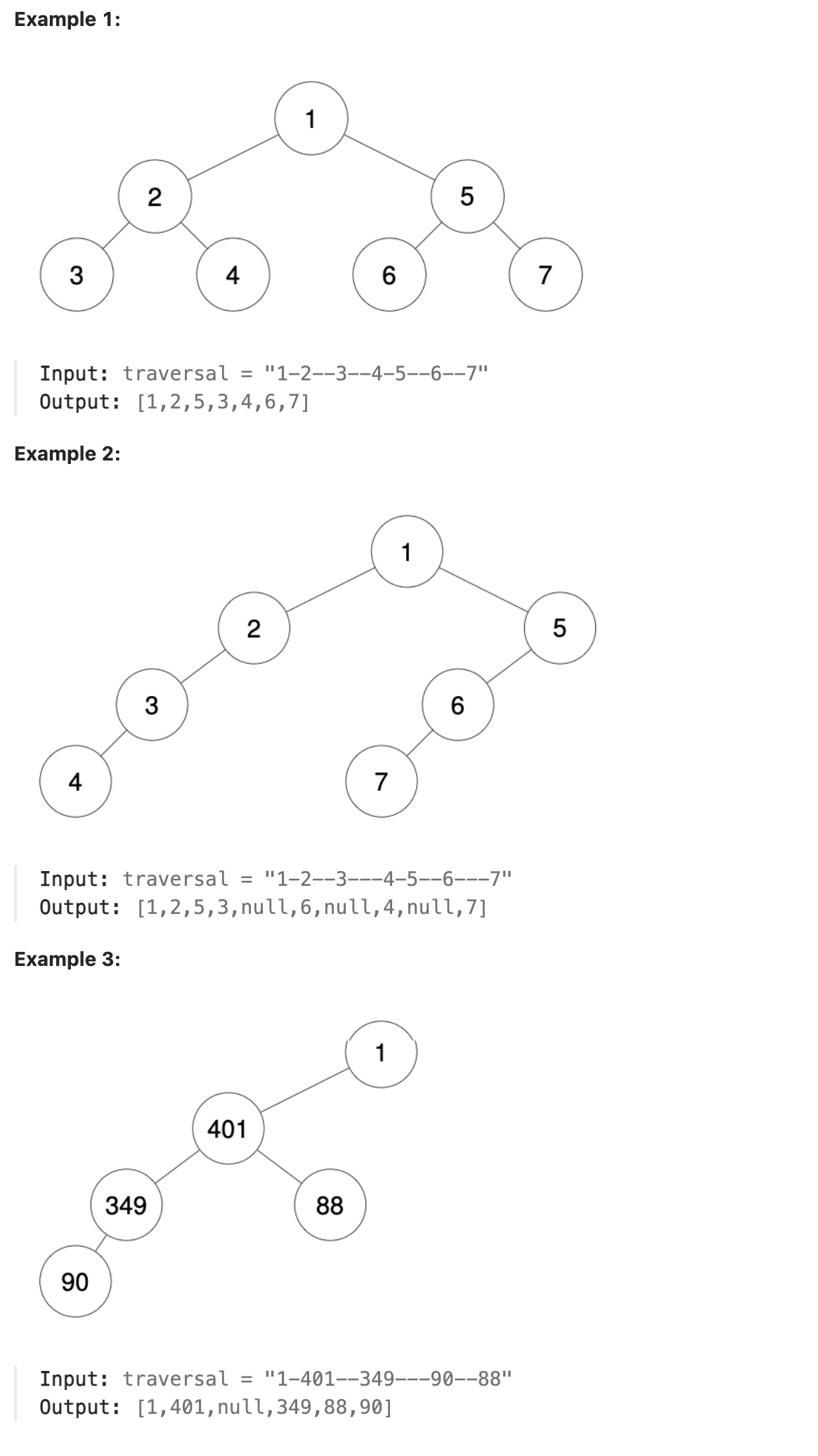
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
本题是一道难题,只要思路清晰还是比较容易解决的,题目中给出的输入为对二叉树进行先序遍历的结果,每个数字前的横线代表该节点所在的深度,则可以直接模拟先序遍历的过程,设置构造函数的参数为字符串和子节点应有的深度,保存一个全局指针指向当前对字符串的扫描位置,扫描字符串获取字符串中下一个节点的深度和节点值,如果节点深度不符合要求,则直接返回,如果节点深度符合要求,则构造新节点并设置节点值,递归尝试给这个新节点构造左右子节点(此处构造时传入的深度为当前的深度加1)。
|
||||||
|
|
||||||
|
除此以外还可以使用栈来保存已经构造好的节点,扫描下一个节点,如果节点深度比栈顶深度小,则弹出栈顶直到栈顶深度为节点深度-1,即弹出栈顶直到栈顶为当前扫描节点的父节点,构造新节点并将其连接到栈顶父节点上,此处要判断是作为左子节点还是右子节点。连接好后将新节点入栈,此处可以发现得到的栈实际上从深度角度来说是一个单调栈。
|
||||||
|
|
||||||
|
这里利用的是先序遍历的特性,即节点的父节点的值一定先于节点的值出现(被遍历到)。
|
||||||
|
### 代码
|
||||||
|
```cpp
|
||||||
|
/**
|
||||||
|
* Definition for a binary tree node.
|
||||||
|
* struct TreeNode {
|
||||||
|
* int val;
|
||||||
|
* TreeNode *left;
|
||||||
|
* TreeNode *right;
|
||||||
|
* TreeNode() : val(0), left(nullptr), right(nullptr) {}
|
||||||
|
* TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
|
||||||
|
* TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left), right(right) {}
|
||||||
|
* };
|
||||||
|
*/
|
||||||
|
class Solution {
|
||||||
|
public:
|
||||||
|
int index = 0;
|
||||||
|
|
||||||
|
TreeNode* recoverFromPreorder(string traversal) {
|
||||||
|
if (traversal.empty()) {
|
||||||
|
return nullptr;
|
||||||
|
}
|
||||||
|
return construct(traversal, 0);
|
||||||
|
}
|
||||||
|
|
||||||
|
TreeNode* construct(const string& traversal, int depth) {
|
||||||
|
if (index >= traversal.length()) {
|
||||||
|
return nullptr;
|
||||||
|
}
|
||||||
|
|
||||||
|
int nextDepth = 0;
|
||||||
|
int start = index;
|
||||||
|
while (index < traversal.length() && traversal[index] == '-') {
|
||||||
|
nextDepth++;
|
||||||
|
index++;
|
||||||
|
}
|
||||||
|
|
||||||
|
if(nextDepth != depth) {
|
||||||
|
index = start;
|
||||||
|
return nullptr;
|
||||||
|
}
|
||||||
|
|
||||||
|
int nextVal = 0;
|
||||||
|
while (index < traversal.length() && isdigit(traversal[index])) {
|
||||||
|
nextVal = nextVal * 10 + (traversal[index] - '0');
|
||||||
|
index++;
|
||||||
|
}
|
||||||
|
|
||||||
|
TreeNode* root = new TreeNode(nextVal);
|
||||||
|
|
||||||
|
root->left = construct(traversal, depth + 1);
|
||||||
|
|
||||||
|
root->right = construct(traversal, depth + 1);
|
||||||
|
|
||||||
|
return root;
|
||||||
|
}
|
||||||
|
};
|
||||||
|
```
|
||||||
|
Loading…
Reference in New Issue
Block a user