mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-04-20 05:52:07 +08:00
leetcode update
This commit is contained in:
parent
cec8338f6e
commit
469a7e349b
@ -3528,3 +3528,86 @@ func addOneRow(root *TreeNode, val int, depth int) *TreeNode {
|
||||
### 总结
|
||||
|
||||
本题也可使用dfs结合变量记录当前深度来解决.
|
||||
|
||||
## day50 2024-04-17
|
||||
|
||||
### 988. Smallest String Starting From Leaf
|
||||
|
||||
You are given the root of a binary tree where each node has a value in the range [0, 25] representing the letters 'a' to 'z'.
|
||||
|
||||
Return the lexicographically smallest string that starts at a leaf of this tree and ends at the root.
|
||||
|
||||
As a reminder, any shorter prefix of a string is lexicographically smaller.
|
||||
|
||||
For example, "ab" is lexicographically smaller than "aba".
|
||||
|
||||
A leaf of a node is a node that has no children.
|
||||
|
||||
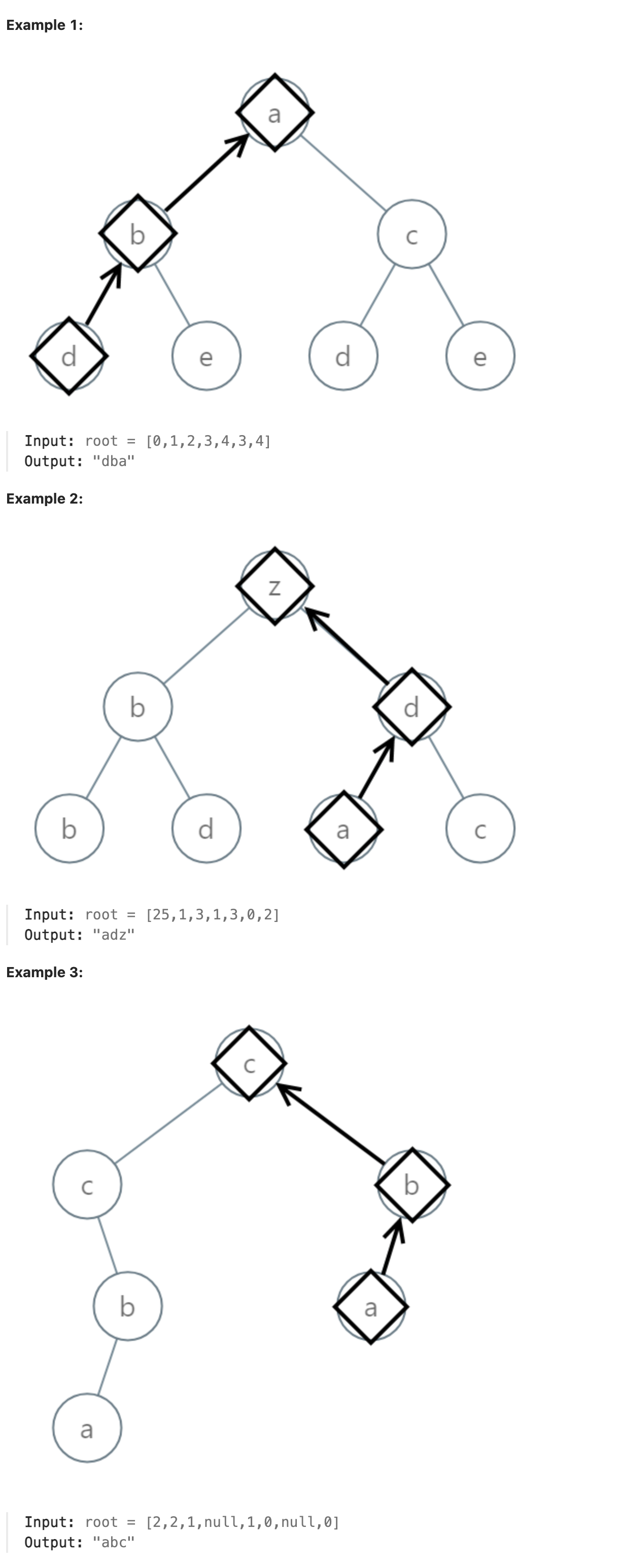
|
||||
|
||||
### 题解
|
||||
|
||||
使用递归实现dfs, 在dfs的过程中将路径上经过的节点数组作为参数传递给子节点, 对子节点执行dfs. dfs中将父路径的数组和左右两个子树返回的数组分别拼接, 对拼接后的数组进行比较, 将较小的作为返回值返回. 最终在根节点即可返回全局最小字典序字符串.
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
/**
|
||||
* Definition for a binary tree node.
|
||||
* type TreeNode struct {
|
||||
* Val int
|
||||
* Left *TreeNode
|
||||
* Right *TreeNode
|
||||
* }
|
||||
*/
|
||||
func smallestFromLeaf(root *TreeNode) string {
|
||||
result := dfs(root,[]int{})
|
||||
var output []byte
|
||||
for _, value := range result {
|
||||
newbyte := []byte{byte('a' + value)}
|
||||
output = append(newbyte, output...)
|
||||
}
|
||||
return string(output)
|
||||
}
|
||||
|
||||
func dfs(root *TreeNode, parent []int) ([]int) {
|
||||
localminstring := []int{root.Val}
|
||||
parentstring := append(parent, root.Val)
|
||||
if root.Left == nil && root.Right == nil {
|
||||
return localminstring
|
||||
} else if root.Left == nil && root.Right != nil {
|
||||
localminstring = append(localminstring, dfs(root.Right, parentstring)...)
|
||||
return localminstring
|
||||
} else if root.Left != nil && root.Right == nil {
|
||||
localminstring = append(localminstring, dfs(root.Left, parentstring)...)
|
||||
return localminstring
|
||||
} else {
|
||||
leftstring := dfs(root.Left, parentstring)
|
||||
rightstring := dfs(root.Right, parentstring)
|
||||
v1 := make([]int,1)
|
||||
v1 = append(v1, parentstring...)
|
||||
v2 := make([]int,0)
|
||||
v2 = append(v2, parentstring...)
|
||||
v1 = append(v1,leftstring...)
|
||||
v2 = append(v2,rightstring...)
|
||||
length1 := len(v1) - 1
|
||||
length2 := len(v2) - 1
|
||||
for length1 >= 0 && length2 >= 0 {
|
||||
if v1[length1] < v2[length2] {
|
||||
return append(localminstring, leftstring...)
|
||||
} else if v1[length1] > v2[length2] {
|
||||
return append(localminstring, rightstring...)
|
||||
}
|
||||
length1--
|
||||
length2--
|
||||
}
|
||||
if length1 > length2 {
|
||||
return append(localminstring, rightstring...)
|
||||
} else {
|
||||
return append(localminstring, leftstring...)
|
||||
}
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
```
|
||||
|
Loading…
Reference in New Issue
Block a user