mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-06-07 11:52:07 +08:00
leetcode update
This commit is contained in:
parent
75ce86639e
commit
41d8d6a860
@ -16340,3 +16340,49 @@ public:
|
|||||||
}
|
}
|
||||||
};
|
};
|
||||||
```
|
```
|
||||||
|
|
||||||
|
## day230 2024-10-24
|
||||||
|
|
||||||
|
### 951. Flip Equivalent Binary Trees
|
||||||
|
|
||||||
|
For a binary tree T, we can define a flip operation as follows: choose any node, and swap the left and right child subtrees.
|
||||||
|
|
||||||
|
A binary tree X is flip equivalent to a binary tree Y if and only if we can make X equal to Y after some number of flip operations.
|
||||||
|
|
||||||
|
Given the roots of two binary trees root1 and root2, return true if the two trees are flip equivalent or false otherwise.
|
||||||
|
|
||||||
|
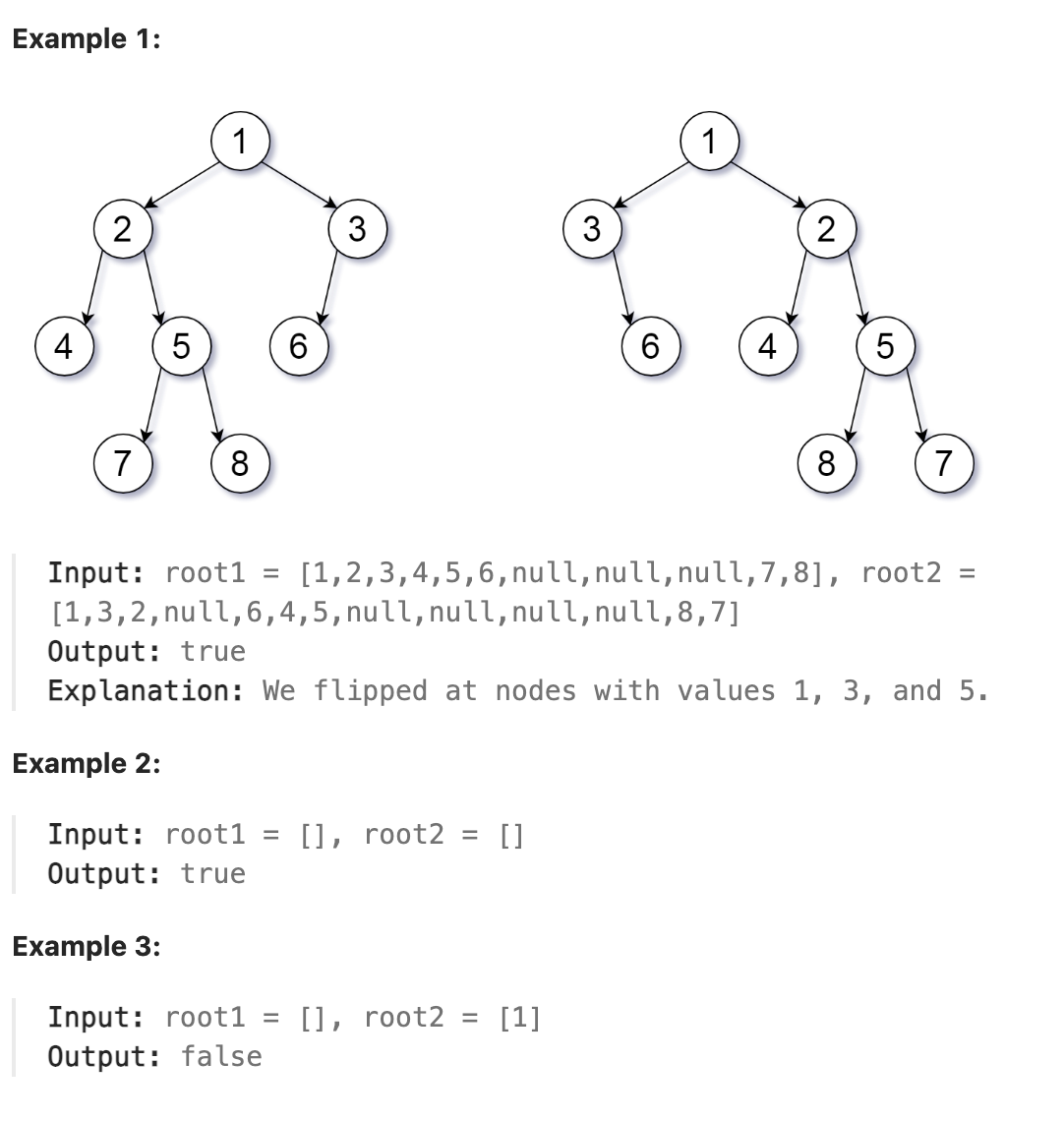
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题在使用dfs遍历树的基础上对dfs进行一些扩充,考虑如果两棵树是翻转等价的,则对于任何一个父节点,其两个子节点的值是一样的,只是值对应的左右子节点可能位置不同(一棵树是值1在左子节点,另一棵则是值1在右子节点),而在使用dfs遍历时无需考虑除当前节点左右子节点之外的节点,其余节点在递归时总会遍历到。
|
||||||
|
|
||||||
|
在dfs时,将两棵树当前节点的指针作为参数,只需判断当前两节点是否相等(包括都为空,或者一个为空一个不为空的情况),相等继续调用dfs判断左右子树是否相同或者为镜像翻转即可。即判断左树和左树相同,右树和右树相同(左右子树相同)或者左树和右树相同,右树和左树相同(左右子树翻转)即可。不相等直接返回false。
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```cpp
|
||||||
|
/**
|
||||||
|
* Definition for a binary tree node.
|
||||||
|
* struct TreeNode {
|
||||||
|
* int val;
|
||||||
|
* TreeNode *left;
|
||||||
|
* TreeNode *right;
|
||||||
|
* TreeNode() : val(0), left(nullptr), right(nullptr) {}
|
||||||
|
* TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
|
||||||
|
* TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left), right(right) {}
|
||||||
|
* };
|
||||||
|
*/
|
||||||
|
class Solution {
|
||||||
|
public:
|
||||||
|
bool flipEquiv(TreeNode* root1, TreeNode* root2) {
|
||||||
|
if (root1 == nullptr && root2 == nullptr){
|
||||||
|
return true;
|
||||||
|
}
|
||||||
|
if((root1 != nullptr && root2 == nullptr)||(root1==nullptr && root2 != nullptr)||(root1->val != root2->val)){
|
||||||
|
return false;
|
||||||
|
}
|
||||||
|
return (flipEquiv(root1->left, root2->left) && flipEquiv(root1->right,root2->right)) || (flipEquiv(root1->right, root2->left) && flipEquiv(root1->left,root2->right));
|
||||||
|
}
|
||||||
|
};
|
||||||
|
```
|
||||||
|
Loading…
Reference in New Issue
Block a user