mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-07-12 09:43:46 +08:00
leetcode update
This commit is contained in:
@ -2580,3 +2580,66 @@ func lengthOfLastWord(s string) int {
|
|||||||
### 总结
|
### 总结
|
||||||
|
|
||||||
前面的题解大多用了go strings库中的函数来按空格分割字符串, 再返回最后一个分割出来的字符串长度. 一般实际应用中, 使用官方库是第一选择, 因为官方库大多对这些操作做了大量优化, 效率会高得多.
|
前面的题解大多用了go strings库中的函数来按空格分割字符串, 再返回最后一个分割出来的字符串长度. 一般实际应用中, 使用官方库是第一选择, 因为官方库大多对这些操作做了大量优化, 效率会高得多.
|
||||||
|
|
||||||
|
## day35 2024-04-02
|
||||||
|
|
||||||
|
### 205. Isomorphic Strings
|
||||||
|
|
||||||
|
Given two strings s and t, determine if they are isomorphic.
|
||||||
|
|
||||||
|
Two strings s and t are isomorphic if the characters in s can be replaced to get t.
|
||||||
|
|
||||||
|
All occurrences of a character must be replaced with another character while preserving the order of characters. No two characters may map to the same character, but a character may map to itself.
|
||||||
|
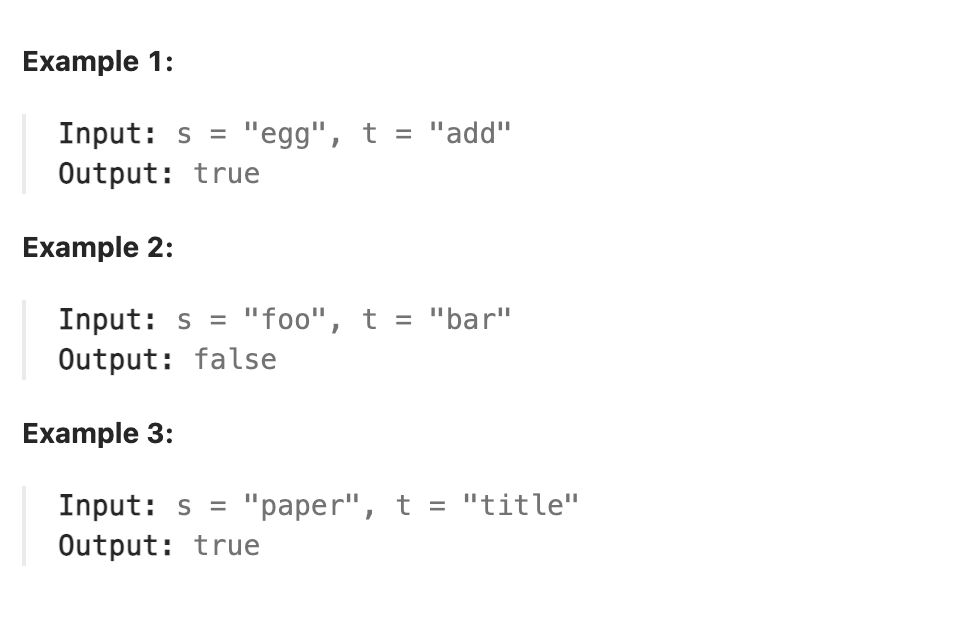
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题是一道简单题, 要求判定s和t是不是同构的, 即类似常说的ABB型这种结构. 本题使用一个哈希表记录字符与字符的映射即可, 如果出现了不同的映射则是不同构的.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
func isIsomorphic(s string, t string) bool {
|
||||||
|
table1 := map[rune]byte{}
|
||||||
|
table2 := map[byte]rune{}
|
||||||
|
for index, value := range s{
|
||||||
|
mapping1, exist := table1[value]
|
||||||
|
mapping2, exist2 := table2[t[index]]
|
||||||
|
if !exist{
|
||||||
|
table1[value] = t[index]
|
||||||
|
}else{
|
||||||
|
if !(mapping1 == t[index]){
|
||||||
|
return false
|
||||||
|
}
|
||||||
|
}
|
||||||
|
if !exist2{
|
||||||
|
table2[t[index]] = value
|
||||||
|
}else{
|
||||||
|
if mapping2 == value{
|
||||||
|
continue
|
||||||
|
}else{
|
||||||
|
return false
|
||||||
|
}
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return true
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
### 总结
|
||||||
|
|
||||||
|
查看前面的题解, 发现了果然0ms用时的思路还是不同凡响, 用字符本身作为下标维护两个数组, 在遍历两个字符串的时候将两个字符串当前的字符作为下标, 将当前遍历的字符串的下标作为值放入两个数组中, 这样就记录了同时出现的两个字符最后的出现的下标, 如果这两个字符一直同时出现则二者下标一直是相同的, 若没有同时出现说明字符映射改变了, 没有继续之前的映射. 则两个字符串就是不同构的. 字符的本质是数, 那么字符可以表示更多的信息, 而不是字符本身, 将其作为数组下标就既使用了字符本身这个信息, 还使用了数组对应位置的元素这个信息, 能使用更多的信息就意味着更高的效率.
|
||||||
|
|
||||||
|
```go
|
||||||
|
func isIsomorphic(s string, t string) bool {
|
||||||
|
s2t := [128]byte{}
|
||||||
|
t2s := [128]byte{}
|
||||||
|
for i, end := 0, len(s); i < end; i++ {
|
||||||
|
if s2t[s[i]] != t2s[t[i]] {
|
||||||
|
return false
|
||||||
|
}
|
||||||
|
s2t[s[i]], t2s[t[i]] = byte(i+1), byte(i+1)
|
||||||
|
}
|
||||||
|
return true
|
||||||
|
}
|
||||||
|
```
|
||||||
|
Reference in New Issue
Block a user