mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-06-20 20:22:07 +08:00
leetcode update
This commit is contained in:
parent
58aaa6996d
commit
2c29fdd167
@ -5517,3 +5517,163 @@ func (hp) Push(any) {}
|
||||
func (hp) Pop() (_ any) { return }
|
||||
|
||||
```
|
||||
|
||||
## day75 2024-05-12
|
||||
|
||||
### 2373. Largest Local Values in a Matrix
|
||||
|
||||
You are given an n x n integer matrix grid.
|
||||
|
||||
Generate an integer matrix maxLocal of size (n - 2) x (n - 2) such that:
|
||||
|
||||
maxLocal[i][j] is equal to the largest value of the 3 x 3 matrix in grid centered around row i + 1 and column j + 1.
|
||||
In other words, we want to find the largest value in every contiguous 3 x 3 matrix in grid.
|
||||
|
||||
Return the generated matrix.
|
||||
|
||||
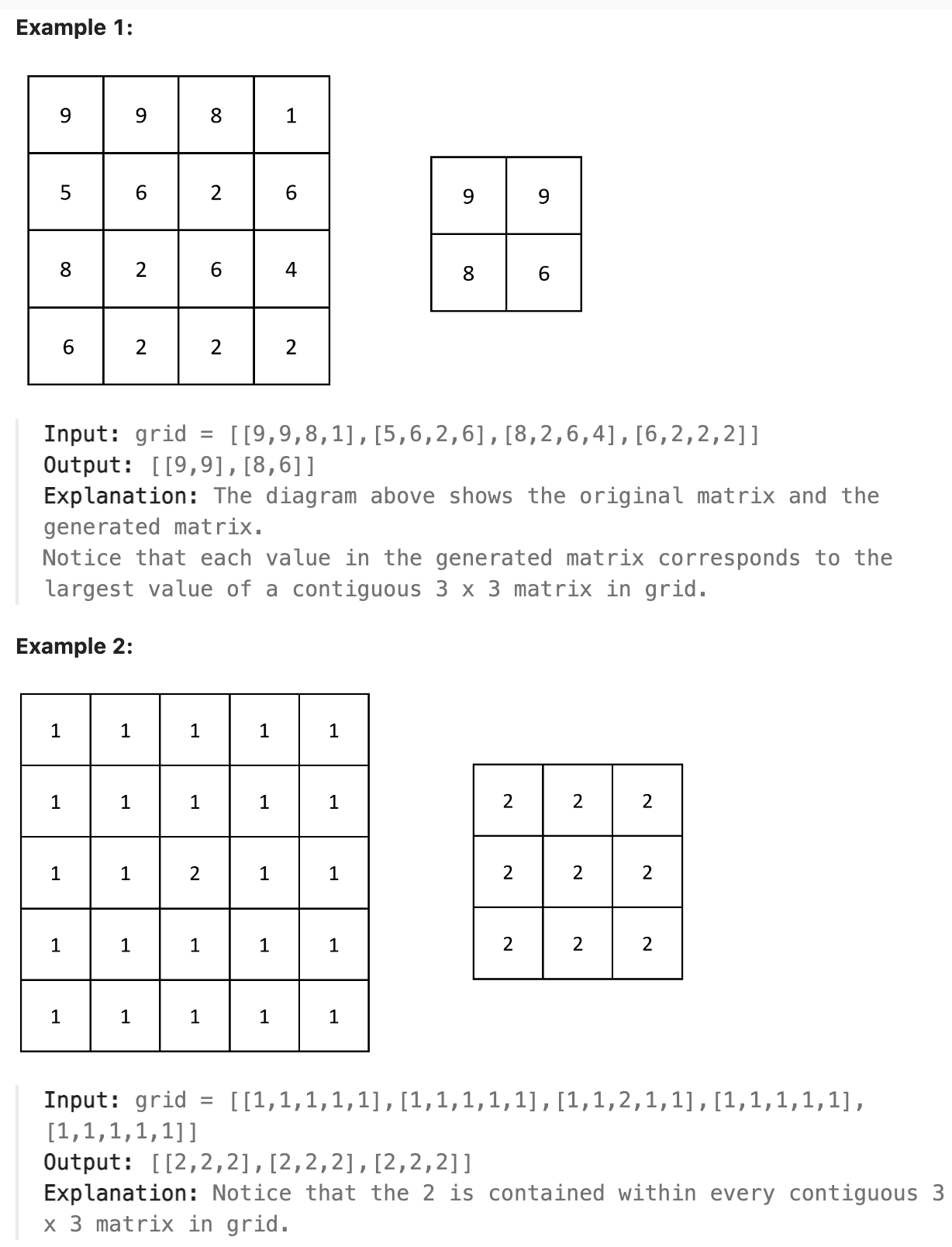
|
||||
|
||||
### 题解
|
||||
|
||||
本题是深度学习中经典的最大池化操作, 用四层循环来完成即可.
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
func largestLocal(grid [][]int) [][]int {
|
||||
n := len(grid)
|
||||
maxLocal := make([][]int, n-2)
|
||||
|
||||
for i := 0; i < n-2; i++ {
|
||||
maxLocal[i] = make([]int, n-2)
|
||||
for j := 0; j < n-2; j++ {
|
||||
max := grid[i][j]
|
||||
for k := 0; k < 3; k++ {
|
||||
for l := 0; l < 3; l++ {
|
||||
if grid[i+k][j+l] > max {
|
||||
max = grid[i+k][j+l]
|
||||
}
|
||||
}
|
||||
}
|
||||
maxLocal[i][j] = max
|
||||
}
|
||||
}
|
||||
|
||||
return maxLocal
|
||||
}
|
||||
```
|
||||
|
||||
## day76 2024-05-13
|
||||
|
||||
### 861. Score After Flipping Matrix
|
||||
|
||||
You are given an m x n binary matrix grid.
|
||||
|
||||
A move consists of choosing any row or column and toggling each value in that row or column (i.e., changing all 0's to 1's, and all 1's to 0's).
|
||||
|
||||
Every row of the matrix is interpreted as a binary number, and the score of the matrix is the sum of these numbers.
|
||||
|
||||
Return the highest possible score after making any number of moves (including zero moves).
|
||||
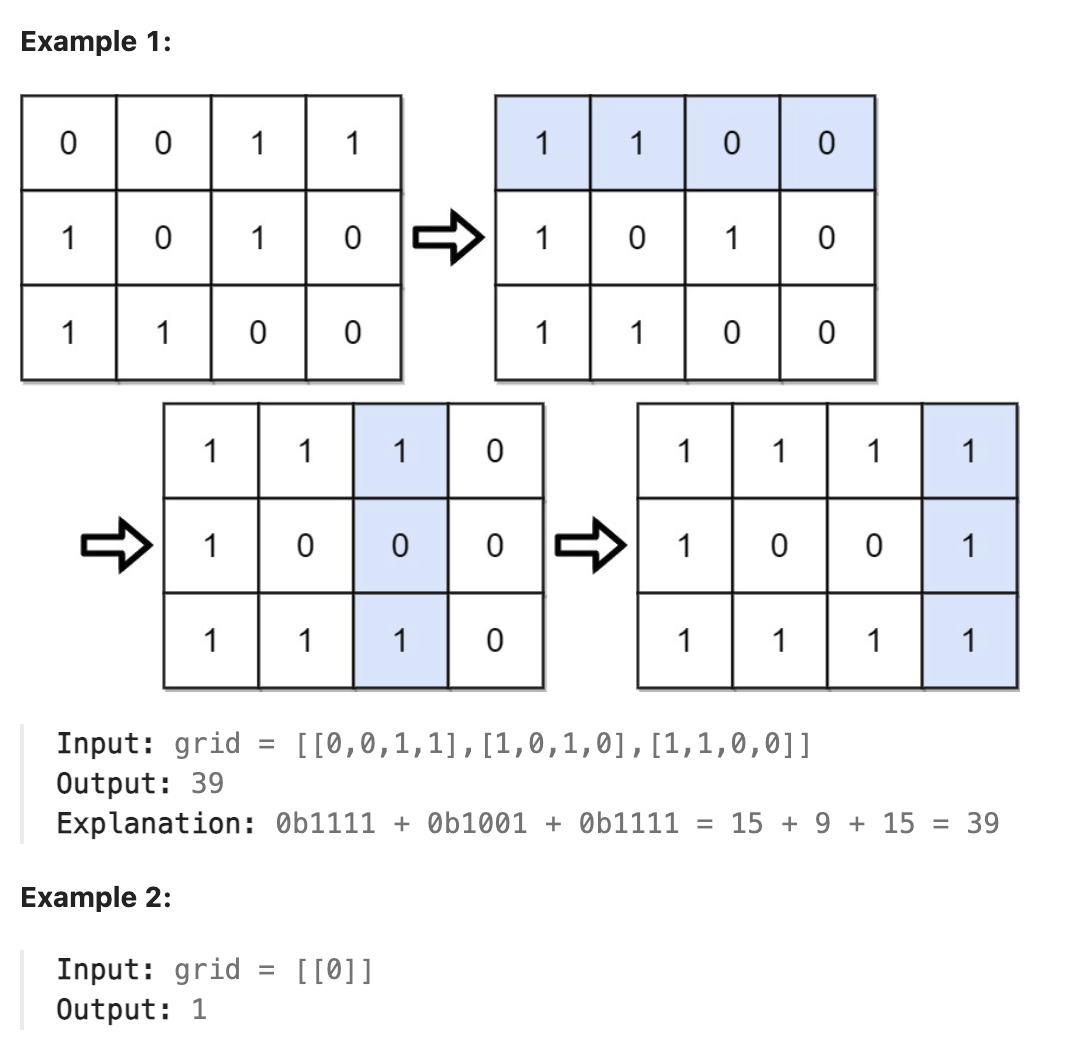
|
||||
|
||||
### 题解
|
||||
|
||||
考虑本题要求的是所有行的数的和. 因此某一行的值中某一位是0或者1对于和来说并不重要, 同一位上所有数中1的个数对和来说才比较重要. 只要某个位上的1的数量最多, 那么无论这些1分布在哪个数中, 最终的和都是最大的. 但每一行中的第一个1是比较特殊的, 考虑无论后面的位数怎么变动都不会超过最高位为1的数的大小, 因此最高位必须为1才能保证最大值.其余位按照列来翻转使得每一列的1的个数最多即可. 注意在遍历数组并执行首位为0的行进行行翻转的过程中可以记录每一列的1的个数, 行翻转后不需要再遍历数组, 只需根据每一列1的个数和列长判断列翻转后1的个数是否更多即可, 取列翻转和不翻转二者中的1的个数最多的数量与对应的位代表的数(可通过移位实现)相乘并累加即可.
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
func matrixScore(grid [][]int) int {
|
||||
collen := len(grid[0])
|
||||
colonenum := make([]int, collen)
|
||||
rowlen := len(grid)
|
||||
result := 0
|
||||
for _, row := range grid{
|
||||
if row[0] == 0{
|
||||
for coli, value := range row{
|
||||
value = value ^ 1
|
||||
colonenum[coli] += value
|
||||
}
|
||||
}else{
|
||||
for coli, value := range row{
|
||||
colonenum[coli] += value
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
for index, value := range colonenum[1:]{
|
||||
value = max(value, rowlen-value)
|
||||
result += value * (1<<(collen-index-2))
|
||||
}
|
||||
result += rowlen * (1<<(collen-1))
|
||||
return result
|
||||
|
||||
|
||||
}
|
||||
```
|
||||
|
||||
## day77 2024-05-14
|
||||
|
||||
### 1219. Path with Maximum Gold
|
||||
|
||||
In a gold mine grid of size m x n, each cell in this mine has an integer representing the amount of gold in that cell, 0 if it is empty.
|
||||
|
||||
Return the maximum amount of gold you can collect under the conditions:
|
||||
|
||||
Every time you are located in a cell you will collect all the gold in that cell.
|
||||
From your position, you can walk one step to the left, right, up, or down.
|
||||
You can't visit the same cell more than once.
|
||||
Never visit a cell with 0 gold.
|
||||
You can start and stop collecting gold from any position in the grid that has some gold.
|
||||
|
||||
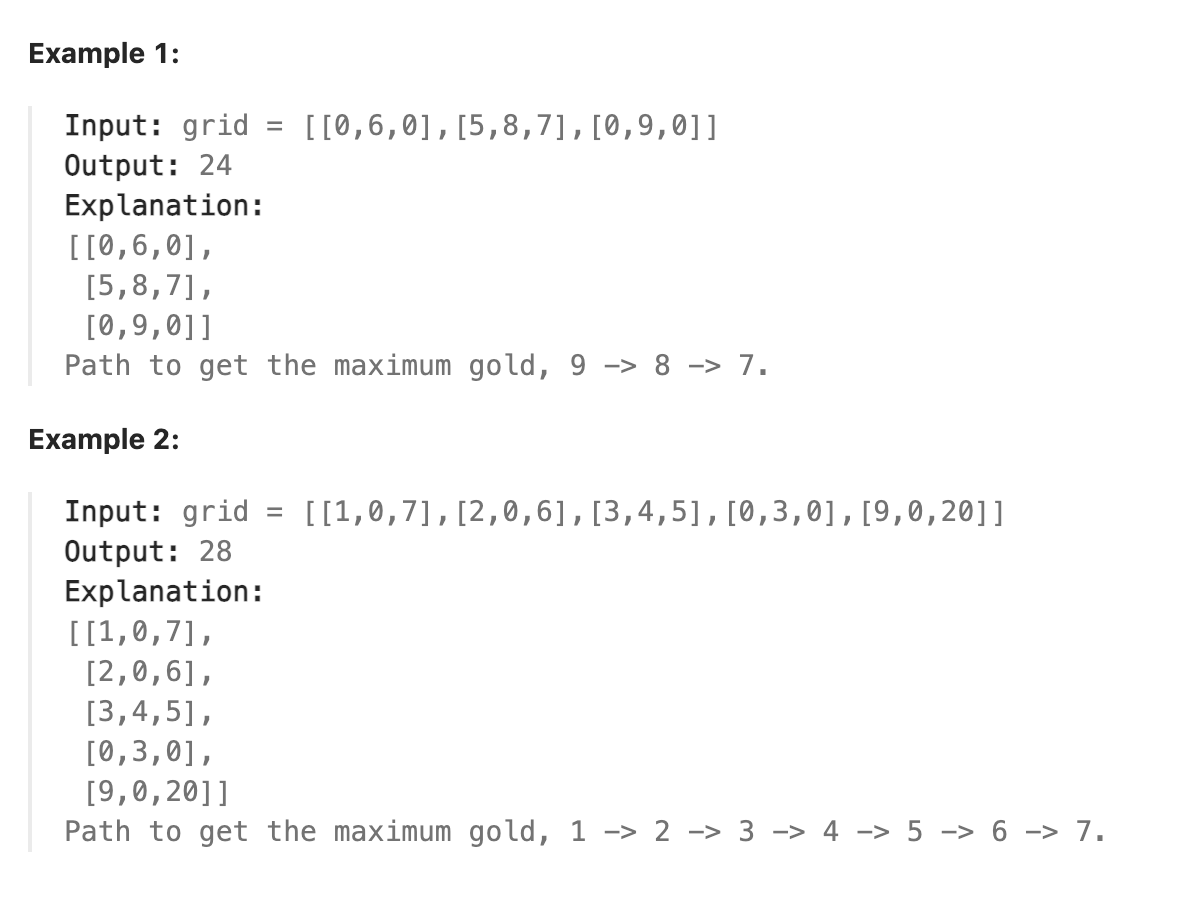
|
||||
|
||||
### 题解
|
||||
|
||||
本题需要找到所有的可行路径并比较每条路径上的金币和来求得最大值. 使用dfs结合回溯即可解决, 注意在数组上进行dfs时探索四个方向可以使用一个方向数组来表示方向, 每次将当前位置的坐标加上方向数组中的某一个方向并判断其是否超过边界及该方向是否有效(值大于0)即可. 注意对当前遍历的路径要先将当前坐标处的值置为0在dfs结束后要将值恢复便于后续回溯.
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
func getMaximumGold(grid [][]int) int {
|
||||
maxgold := 0
|
||||
rowlen := len(grid)
|
||||
collen := len(grid[0])
|
||||
var dfs func(int,int,[][]int)int
|
||||
direction := [][]int{{0,1},{0,-1},{1,0},{-1,0}}
|
||||
dfs = func(rowi int, coli int, grid [][]int)int{
|
||||
tempmax := 0
|
||||
temp := grid[rowi][coli]
|
||||
grid[rowi][coli] = 0
|
||||
for _, dir := range direction{
|
||||
temprow := rowi
|
||||
tempcol := coli
|
||||
temprow += dir[0]
|
||||
tempcol += dir[1]
|
||||
if temprow >= 0 && temprow < rowlen && tempcol >= 0 && tempcol < collen && grid[temprow][tempcol] > 0{
|
||||
tempmax = max(tempmax, dfs(temprow, tempcol, grid))
|
||||
}
|
||||
}
|
||||
grid[rowi][coli] = temp
|
||||
return tempmax+temp
|
||||
}
|
||||
|
||||
|
||||
for rowi, row := range grid{
|
||||
for coli, value := range row{
|
||||
if value != 0{
|
||||
maxgold = max(dfs(rowi, coli, grid),maxgold)
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
return maxgold
|
||||
}
|
||||
|
||||
|
||||
```
|
||||
|
||||
### 总结
|
||||
|
||||
其实上面代码还有优化的空间, 在遍历数组并走所有路径的过程中, 显然有些路径是重复的, 可以再使用一个三维数组保存二维数组中某一位向四个方向走的路径得到的四个方向的最大值. 这样在遇到重复路径时直接返回值即可. 不用再次递归遍历.
|
||||
|
Loading…
Reference in New Issue
Block a user