mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-06-07 11:52:07 +08:00
add leetcode content
This commit is contained in:
parent
8f269ef2b1
commit
28eb47a664
@ -569,3 +569,84 @@ func minimumLength(s string) int {
|
|||||||
### 总结
|
### 总结
|
||||||
|
|
||||||
本题值得注意的地方在于for循环中条件的设置, 可能会忽略与运算符两侧条件表达式的前后顺序, 但由于短路机制的存在, 与运算符两侧表达式的前后顺序有时非常重要, 例如在本题中, 如果将forward<backward条件设置在前面, 则当forward到达数组的边界时会直接退出循环, 但若将相等判断放在前面, 会因为当forward到达数组边界时forward+1下标越界而出错.
|
本题值得注意的地方在于for循环中条件的设置, 可能会忽略与运算符两侧条件表达式的前后顺序, 但由于短路机制的存在, 与运算符两侧表达式的前后顺序有时非常重要, 例如在本题中, 如果将forward<backward条件设置在前面, 则当forward到达数组的边界时会直接退出循环, 但若将相等判断放在前面, 会因为当forward到达数组边界时forward+1下标越界而出错.
|
||||||
|
|
||||||
|
## day9 2024-03-06
|
||||||
|
|
||||||
|
### 141. Linked List Cycle
|
||||||
|
|
||||||
|
Given head, the head of a linked list, determine if the linked list has a cycle in it.
|
||||||
|
|
||||||
|
There is a cycle in a linked list if there is some node in the list that can be reached again by continuously following the next pointer. Internally, pos is used to denote the index of the node that tail's next pointer is connected to. Note that pos is not passed as a parameter.
|
||||||
|
|
||||||
|
Return true if there is a cycle in the linked list. Otherwise, return false.
|
||||||
|
|
||||||
|
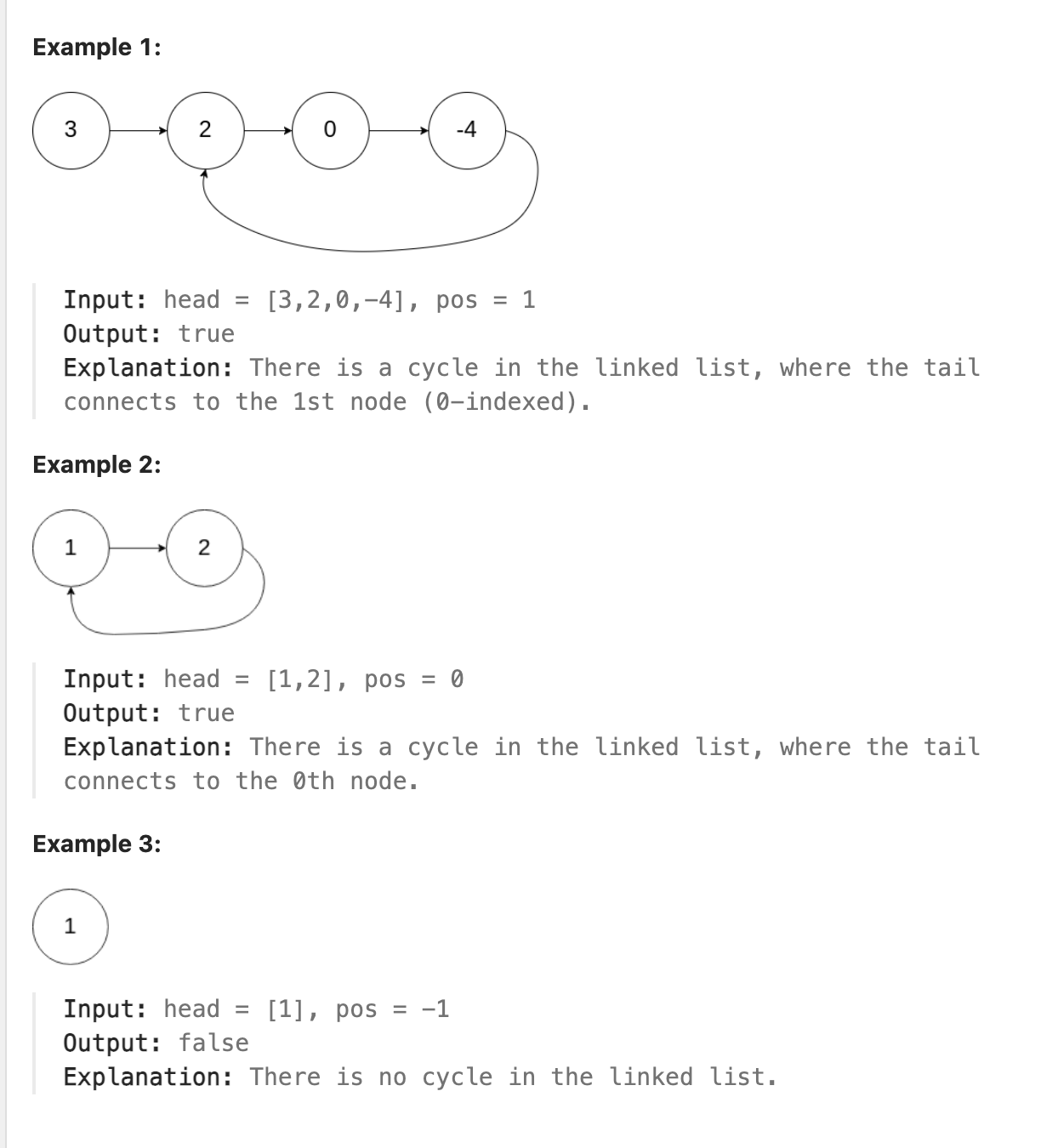
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题最简单的思路就是使用并查集, 因为go语言中的map类型在获取数据时会返回该键值在map中是否存在, 因此可以将map类型直接当作一个简单的并查集使用.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
/**
|
||||||
|
* Definition for singly-linked list.
|
||||||
|
* type ListNode struct {
|
||||||
|
* Val int
|
||||||
|
* Next *ListNode
|
||||||
|
* }
|
||||||
|
*/
|
||||||
|
|
||||||
|
|
||||||
|
func hasCycle(head *ListNode) bool {
|
||||||
|
if head==nil{
|
||||||
|
return false
|
||||||
|
}
|
||||||
|
ptrs := make(map[*ListNode]int)
|
||||||
|
var exist bool
|
||||||
|
ptrs[head] = 1
|
||||||
|
for head.Next != nil{
|
||||||
|
_, exist = ptrs[head.Next]
|
||||||
|
if !exist{
|
||||||
|
ptrs[head.Next] = 1
|
||||||
|
head = head.Next
|
||||||
|
} else{
|
||||||
|
return true
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return false
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
### 总结
|
||||||
|
|
||||||
|
题目中给出提示本题可以使用O(1)的空间复杂度解决, 即使用常数空间复杂度, 研究他人题解发现本题同样可以使用快慢指针,核心思想类似于加速度, 快指针的加速度比慢指针要快, 即快指针每次移动的步长比慢指针移动的步长多1. 这样若链表中有环则快指针最终总能比慢指针快整整一个环的长度从而追上慢指针. 若没有环则快指针永远不可能追上慢指针. 类似于跑步时如果一直跑, 跑得快的同学最终总能套跑得慢的同学一圈. 快慢指针在很多情景下都有很巧妙的应用. 后续可以在遇到多个相关题目后加以总结. 给出快慢指针解决本题的代码示例
|
||||||
|
|
||||||
|
```go
|
||||||
|
/**
|
||||||
|
* Definition for singly-linked list.
|
||||||
|
* type ListNode struct {
|
||||||
|
* Val int
|
||||||
|
* Next *ListNode
|
||||||
|
* }
|
||||||
|
*/
|
||||||
|
func hasCycle(head *ListNode) bool {
|
||||||
|
if head == nil {
|
||||||
|
return false
|
||||||
|
}
|
||||||
|
|
||||||
|
slow := head
|
||||||
|
fast := head
|
||||||
|
|
||||||
|
for slow != nil && fast != nil && fast.Next != nil {
|
||||||
|
slow = slow.Next
|
||||||
|
fast = fast.Next.Next
|
||||||
|
|
||||||
|
if slow == fast {
|
||||||
|
return true
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
return false
|
||||||
|
}
|
||||||
|
```
|
||||||
|
Loading…
Reference in New Issue
Block a user