mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-04-20 05:52:07 +08:00
leetcode update
This commit is contained in:
parent
ecac9ba497
commit
1a58d25fc8
@ -5109,3 +5109,205 @@ func deleteNode(node *ListNode) {
|
|||||||
node.Next = nil
|
node.Next = nil
|
||||||
}
|
}
|
||||||
```
|
```
|
||||||
|
|
||||||
|
## day69 2024-05-06
|
||||||
|
|
||||||
|
### 2487. Remove Nodes From Linked List
|
||||||
|
|
||||||
|
You are given the head of a linked list.
|
||||||
|
|
||||||
|
Remove every node which has a node with a greater value anywhere to the right side of it.
|
||||||
|
|
||||||
|
Return the head of the modified linked list.
|
||||||
|
|
||||||
|
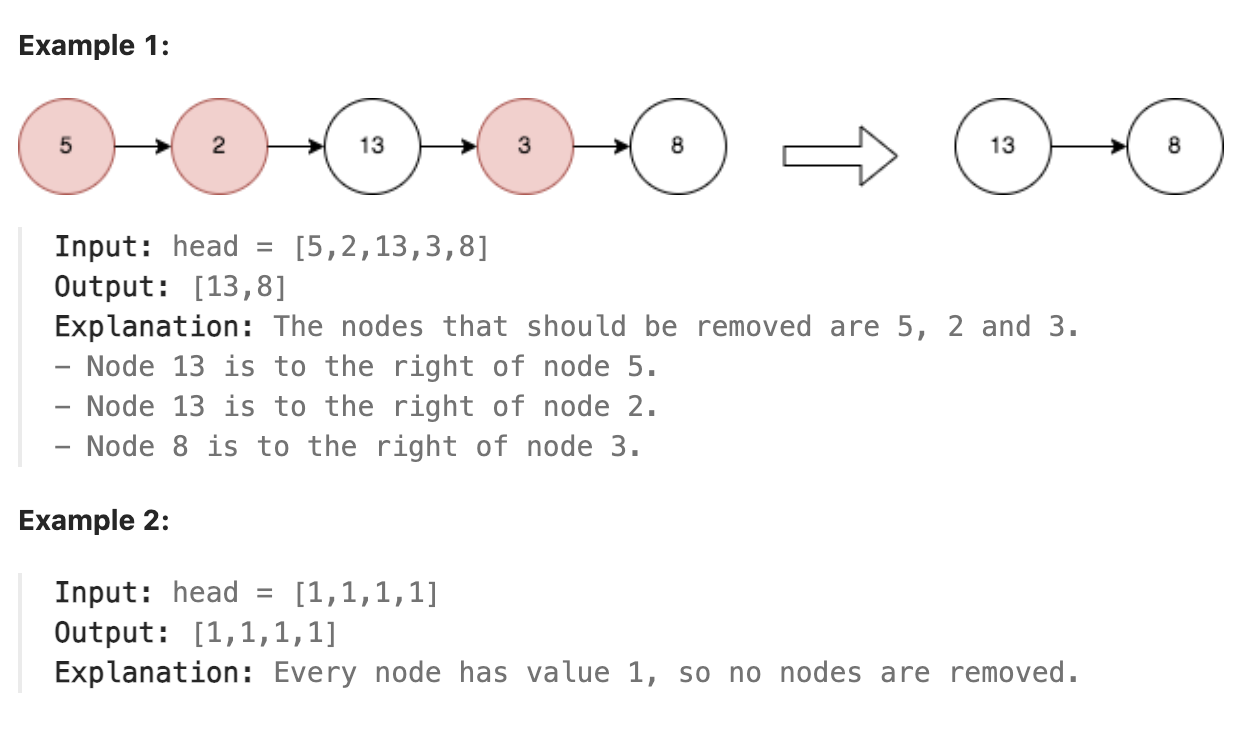
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题要删除的是链表右边存在比它值大的节点的节点. 最直接的思路就是将链表反向, 从头遍历, 保存当前遍历过的最大值, 小于该值的都删除, 大于则更新当前最大值, 删除完毕后再将链表反向, 就得到了删除后的结果.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
/**
|
||||||
|
* Definition for singly-linked list.
|
||||||
|
* type ListNode struct {
|
||||||
|
* Val int
|
||||||
|
* Next *ListNode
|
||||||
|
* }
|
||||||
|
*/
|
||||||
|
func removeNodes(head *ListNode) *ListNode {
|
||||||
|
newhead := reverse(head)
|
||||||
|
curhead := newhead
|
||||||
|
maxval := newhead.Val
|
||||||
|
for newhead.Next != nil{
|
||||||
|
if newhead.Next.Val < maxval{
|
||||||
|
newhead.Next = newhead.Next.Next
|
||||||
|
}else{
|
||||||
|
maxval = newhead.Next.Val
|
||||||
|
newhead = newhead.Next
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return reverse(curhead)
|
||||||
|
|
||||||
|
|
||||||
|
}
|
||||||
|
|
||||||
|
func reverse(head *ListNode) *ListNode {
|
||||||
|
var pre *ListNode = nil
|
||||||
|
cur := head
|
||||||
|
for cur != nil {
|
||||||
|
nextTemp := cur.Next
|
||||||
|
cur.Next = pre
|
||||||
|
pre = cur
|
||||||
|
cur = nextTemp
|
||||||
|
}
|
||||||
|
return pre
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
## day70 2024-05-07
|
||||||
|
|
||||||
|
### 2816. Double a Number Represented as a Linked List
|
||||||
|
|
||||||
|
You are given the head of a non-empty linked list representing a non-negative integer without leading zeroes.
|
||||||
|
|
||||||
|
Return the head of the linked list after doubling it
|
||||||
|
|
||||||
|
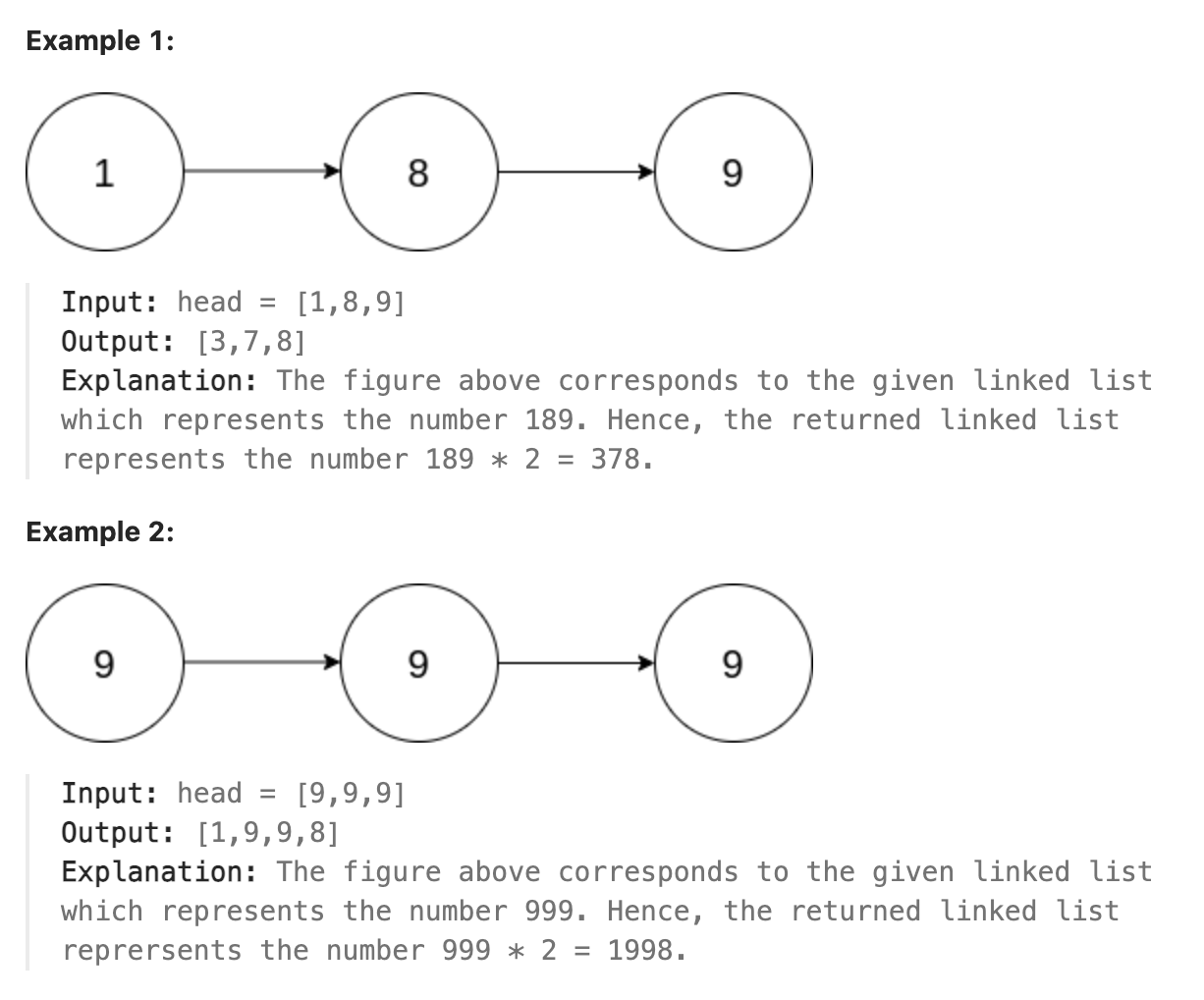
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
和昨天类似的解题思路, 将链表逆转, 依次计算各位并保存进位, 修改值最后一个节点有进位增加一个新的值为1的节点即可.再将链表逆转回来.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
/**
|
||||||
|
* Definition for singly-linked list.
|
||||||
|
* type ListNode struct {
|
||||||
|
* Val int
|
||||||
|
* Next *ListNode
|
||||||
|
* }
|
||||||
|
*/
|
||||||
|
func doubleIt(head *ListNode) *ListNode {
|
||||||
|
newhead := reverse(head)
|
||||||
|
curhead := newhead
|
||||||
|
addon := 0
|
||||||
|
for newhead.Next != nil{
|
||||||
|
newhead.Val = newhead.Val * 2 + addon
|
||||||
|
if newhead.Val >= 10{
|
||||||
|
newhead.Val = newhead.Val - 10
|
||||||
|
addon = 1
|
||||||
|
}else{
|
||||||
|
addon = 0
|
||||||
|
}
|
||||||
|
newhead = newhead.Next
|
||||||
|
}
|
||||||
|
newhead.Val = newhead.Val * 2 + addon
|
||||||
|
if newhead.Val >= 10{
|
||||||
|
newhead.Val = newhead.Val - 10
|
||||||
|
newhead.Next = &ListNode{1,nil}
|
||||||
|
}
|
||||||
|
|
||||||
|
|
||||||
|
return reverse(curhead)
|
||||||
|
|
||||||
|
}
|
||||||
|
|
||||||
|
func reverse(head *ListNode) *ListNode{
|
||||||
|
prev := head
|
||||||
|
prev = nil
|
||||||
|
next := head
|
||||||
|
for head != nil{
|
||||||
|
next = head.Next
|
||||||
|
head.Next = prev
|
||||||
|
prev = head
|
||||||
|
head = next
|
||||||
|
}
|
||||||
|
return prev
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
### 总结
|
||||||
|
|
||||||
|
逆转其实是多余的, 每一位数仅取决于当前位的值和后一位是否有进位, 因此用两个指针分别指向当前位和下一位, 计算下一位的值若进位将进位加到当前位即可, 这里的核心思路在于将当前位的倍乘和进位的加两个操作分离了, 在当前位的上一位时执行当前位的倍乘操作, 在当前位下一位执行当前位的进位加. 因为进位不受后面的位的影响, 因此使用双指针即可快速解决.
|
||||||
|
|
||||||
|
```go
|
||||||
|
/**
|
||||||
|
* Definition for singly-linked list.
|
||||||
|
* type ListNode struct {
|
||||||
|
* Val int
|
||||||
|
* Next *ListNode
|
||||||
|
* }
|
||||||
|
*/
|
||||||
|
|
||||||
|
func doubleIt(head *ListNode) *ListNode {
|
||||||
|
|
||||||
|
head = &ListNode{Next: head}
|
||||||
|
|
||||||
|
for curr, next := head, head.Next; next != nil; curr, next = next, next.Next {
|
||||||
|
next.Val *= 2
|
||||||
|
curr.Val += next.Val / 10
|
||||||
|
next.Val %= 10
|
||||||
|
}
|
||||||
|
|
||||||
|
if head.Val == 0 {
|
||||||
|
head = head.Next
|
||||||
|
}
|
||||||
|
|
||||||
|
return head
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
## day71 2024-05-08
|
||||||
|
|
||||||
|
### 506. Relative Ranks
|
||||||
|
|
||||||
|
You are given an integer array score of size n, where score[i] is the score of the ith athlete in a competition. All the scores are guaranteed to be unique.
|
||||||
|
|
||||||
|
The athletes are placed based on their scores, where the 1st place athlete has the highest score, the 2nd place athlete has the 2nd highest score, and so on. The placement of each athlete determines their rank:
|
||||||
|
|
||||||
|
The 1st place athlete's rank is "Gold Medal".
|
||||||
|
The 2nd place athlete's rank is "Silver Medal".
|
||||||
|
The 3rd place athlete's rank is "Bronze Medal".
|
||||||
|
For the 4th place to the nth place athlete, their rank is their placement number (i.e., the xth place athlete's rank is "x").
|
||||||
|
Return an array answer of size n where answer[i] is the rank of the ith athlete.
|
||||||
|
|
||||||
|
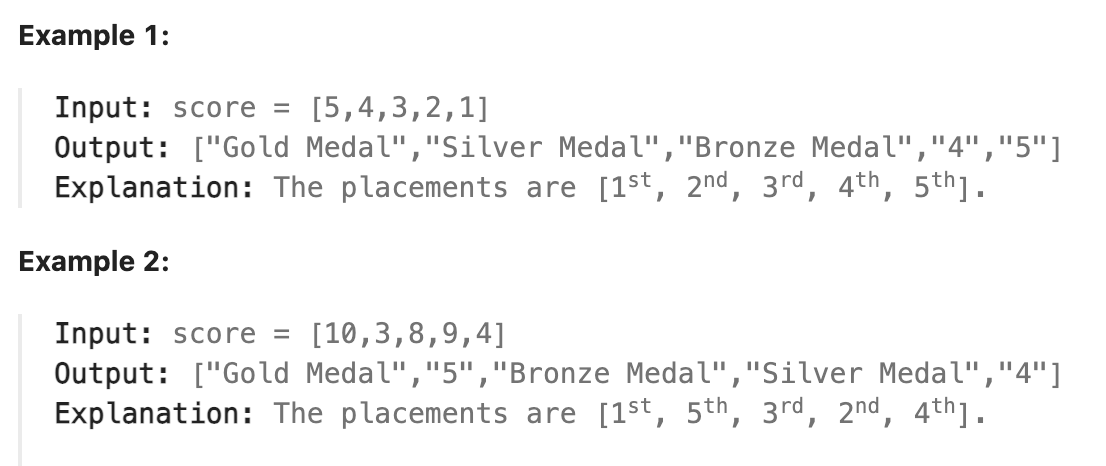
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题为简单题, 思路上比较清晰, 先排序, 然后保存对应score的排名, 再遍历一次数组, 根据score的排名赋予对应的rank即可, 特殊处理前三个即可.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
func findRelativeRanks(score []int) []string {
|
||||||
|
var copy []int
|
||||||
|
copy = append(copy, score...)
|
||||||
|
index, value := 0,0
|
||||||
|
|
||||||
|
|
||||||
|
sort.Sort(sort.Reverse(sort.IntSlice(copy)))
|
||||||
|
ranks := make([]int, 1000001)
|
||||||
|
for index,value = range copy{
|
||||||
|
ranks[value] = index+1
|
||||||
|
}
|
||||||
|
result := []string{}
|
||||||
|
for _, value = range score{
|
||||||
|
if ranks[value] == 1{
|
||||||
|
result = append(result, "Gold Medal")
|
||||||
|
}else if ranks[value] == 2{
|
||||||
|
result = append(result, "Silver Medal")
|
||||||
|
}else if ranks[value] == 3{
|
||||||
|
result = append(result, "Bronze Medal")
|
||||||
|
}else{
|
||||||
|
result = append(result, strconv.Itoa(ranks[value]))
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return result
|
||||||
|
|
||||||
|
|
||||||
|
}
|
||||||
|
```
|
||||||
|
Loading…
Reference in New Issue
Block a user