mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-06-07 11:52:07 +08:00
leetcode update
This commit is contained in:
parent
6c2c6e14d9
commit
19b03cbffe
@ -3703,7 +3703,7 @@ An island is surrounded by water and is formed by connecting adjacent lands hori
|
||||
|
||||
### 题解
|
||||
|
||||
本题遍历矩阵, 遇到1时对该元素执行bfs, 并将搜索过程中遇到的所有1置为0. 继续遍历, 重复此过程即可.
|
||||
本题遍历矩阵, 遇到1时对该元素执行bfs, 并将搜索过程中遇到的所有1置为0. 搜索结束后将最终结果加一. 继续遍历, 重复此过程即可.
|
||||
|
||||
### 代码
|
||||
|
||||
@ -3782,3 +3782,57 @@ func findIsl(i, j int, m [][]byte) int {
|
||||
}
|
||||
|
||||
```
|
||||
|
||||
## day53 2024-04-20
|
||||
|
||||
### 1992. Find All Groups of Farmland
|
||||
|
||||
You are given a 0-indexed m x n binary matrix land where a 0 represents a hectare of forested land and a 1 represents a hectare of farmland.
|
||||
|
||||
To keep the land organized, there are designated rectangular areas of hectares that consist entirely of farmland. These rectangular areas are called groups. No two groups are adjacent, meaning farmland in one group is not four-directionally adjacent to another farmland in a different group.
|
||||
|
||||
land can be represented by a coordinate system where the top left corner of land is (0, 0) and the bottom right corner of land is (m-1, n-1). Find the coordinates of the top left and bottom right corner of each group of farmland. A group of farmland with a top left corner at (r1, c1) and a bottom right corner at (r2, c2) is represented by the 4-length array [r1, c1, r2, c2].
|
||||
|
||||
Return a 2D array containing the 4-length arrays described above for each group of farmland in land. If there are no groups of farmland, return an empty array. You may return the answer in any order.
|
||||
|
||||
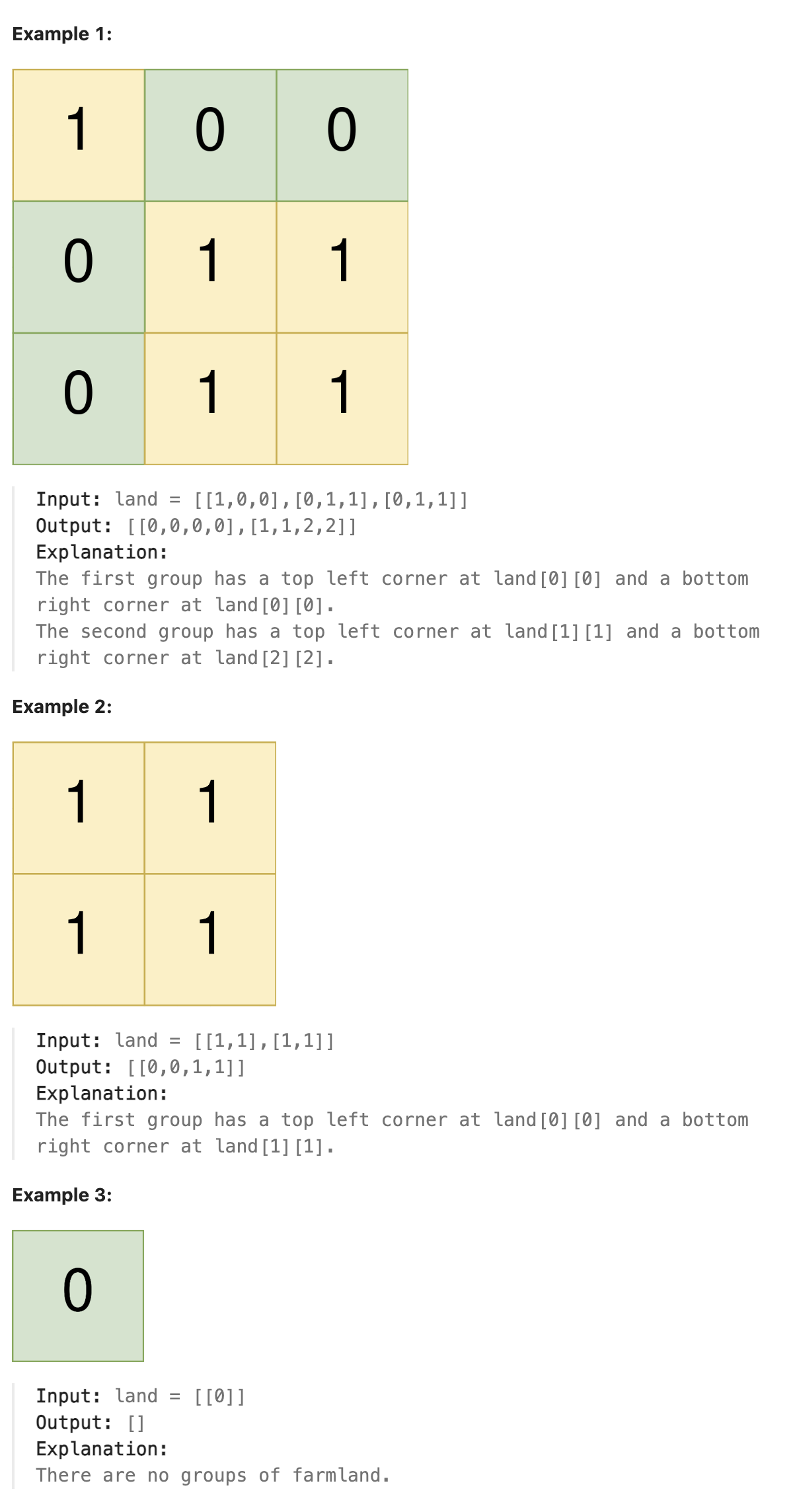
|
||||
|
||||
### 题解
|
||||
|
||||
本题限定了农田只会是一个矩形, 因此遍历数组,遇到1时向右向下遍历, 即可知道这个矩形的长和宽. 将左上和右下坐标插入矩形坐标数组中, 并将该片矩形全部置零. 如此反复即可. 在全部置零后将右下角坐标插入矩形坐标数组中, 并将坐标数组放入结果数组.
|
||||
|
||||
### 代码
|
||||
|
||||
```go
|
||||
func findFarmland(land [][]int) [][]int {
|
||||
rowlen := len(land)
|
||||
collen := len(land[0])
|
||||
result := [][]int{}
|
||||
nextrow := 0
|
||||
nextcol := 0
|
||||
for rowindex,row := range land{
|
||||
for colindex,_ := range row{
|
||||
if land[rowindex][colindex] == 1{
|
||||
farmland := []int{rowindex, colindex}
|
||||
nextrow = rowindex
|
||||
nextcol = colindex
|
||||
for nextrow < rowlen{
|
||||
if land[nextrow][colindex] == 0{
|
||||
break
|
||||
}
|
||||
nextcol = colindex
|
||||
for nextcol < collen && land[nextrow][nextcol] == 1{
|
||||
land[nextrow][nextcol] = 0
|
||||
nextcol++
|
||||
}
|
||||
nextrow++
|
||||
}
|
||||
farmland = append(farmland, nextrow-1)
|
||||
farmland = append(farmland, nextcol-1)
|
||||
result = append(result, farmland)
|
||||
}
|
||||
}
|
||||
}
|
||||
return result
|
||||
}
|
||||
```
|
||||
|
Loading…
Reference in New Issue
Block a user