mirror of
https://gitlab.com/game-loader/hugo.git
synced 2025-07-12 09:43:46 +08:00
leetcode update
This commit is contained in:
@ -7316,3 +7316,80 @@ func sortColors(nums []int) {
|
|||||||
### 总结
|
### 总结
|
||||||
|
|
||||||
思路是正确的, 但在实现时有一些细节要注意, 如遇到2将其交换到末尾时, 如果被交换过来的数也是2则要继续将其移到末尾, 直到交换过来的数不是2或者已经将当前下标后面的所有数都交换为2为止.
|
思路是正确的, 但在实现时有一些细节要注意, 如遇到2将其交换到末尾时, 如果被交换过来的数也是2则要继续将其移到末尾, 直到交换过来的数不是2或者已经将当前下标后面的所有数都交换为2为止.
|
||||||
|
|
||||||
|
## day108 2024-06-13
|
||||||
|
|
||||||
|
### 2037. Minimum Number of Moves to Seat Everyone
|
||||||
|
|
||||||
|
There are n seats and n students in a room. You are given an array seats of length n, where seats[i] is the position of the ith seat. You are also given the array students of length n, where students[j] is the position of the jth student.
|
||||||
|
|
||||||
|
You may perform the following move any number of times:
|
||||||
|
|
||||||
|
Increase or decrease the position of the ith student by 1 (i.e., moving the ith student from position x to x + 1 or x - 1)
|
||||||
|
Return the minimum number of moves required to move each student to a seat such that no two students are in the same seat.
|
||||||
|
|
||||||
|
Note that there may be multiple seats or students in the same position at the beginning.
|
||||||
|
|
||||||
|
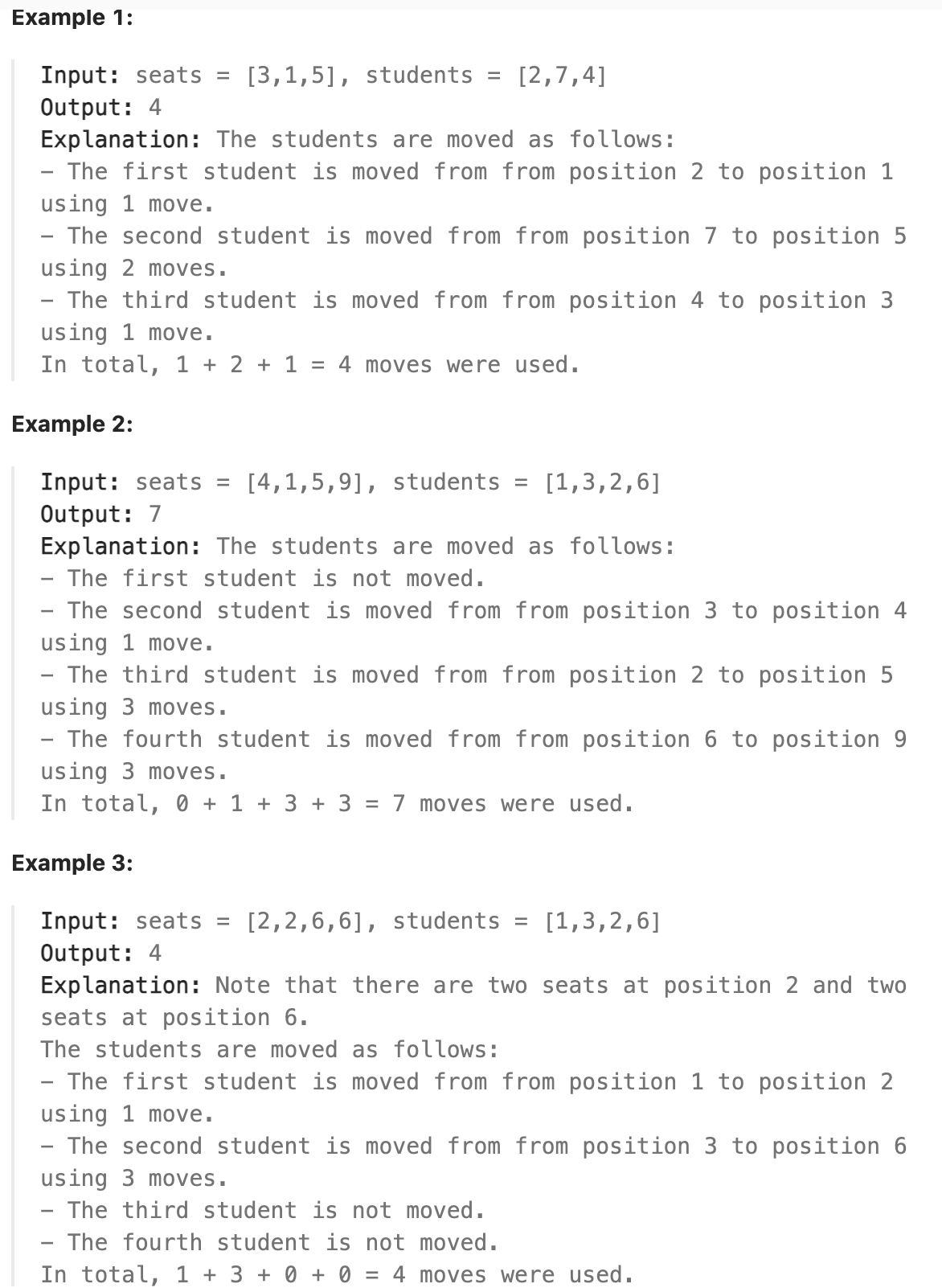
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题总体思路比较直观, 因为找最小的移动次数让每个同学都坐在某个位置的椅子上, 因此只要找到同学位置和椅子位置最小的差值和就可以得到答案. 首先将椅子位置和同学位置均排序, 再从头遍历数组将二者差加和即得最终结果, 是一种贪心算法, 这里分析一下为什么排序后将椅子和同学的位置依次做差就能得到正确答案. 考虑数组中的任意两个位置, 假设椅子对应的位置为i和i+k, 同学对应的位置为j和j+m, 若则无论j,j+m和i的大小关系如何, 这两个同学挪到两个椅子的最小值均为|j-i|+|j+m-i-k|(可以自行考虑i,i+k,j,j+m这四个数的全部不同位置关系, 一一列举即可得出结论). 由两个位置的情况可推得一般情况, 即两个数组长度相同且均从小到大排序的情况下, 两个数组各个元素的差值的最小和就是各个位置元素差的和(还可以思考无论哪个同学坐到哪个位置上, 总要有另一个同学坐在另一个位置上从而将这两个同学的距离补上, 因此按照顺序一一就坐就是最优的).
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
func minMovesToSeat(seats []int, students []int) int {
|
||||||
|
sort.Ints(seats)
|
||||||
|
sort.Ints(students)
|
||||||
|
result := 0
|
||||||
|
for i,value := range seats{
|
||||||
|
if value > students[i]{
|
||||||
|
result += value - students[i]
|
||||||
|
}else{
|
||||||
|
result += students[i] - value
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return result
|
||||||
|
}
|
||||||
|
```
|
||||||
|
|
||||||
|
## day109 2024-06-14
|
||||||
|
|
||||||
|
### 945. Minimum Increment to Make Array Unique
|
||||||
|
|
||||||
|
You are given an integer array nums. In one move, you can pick an index i where 0 <= i < nums.length and increment nums[i] by 1.
|
||||||
|
|
||||||
|
Return the minimum number of moves to make every value in nums unique.
|
||||||
|
|
||||||
|
The test cases are generated so that the answer fits in a 32-bit integer.
|
||||||
|
|
||||||
|
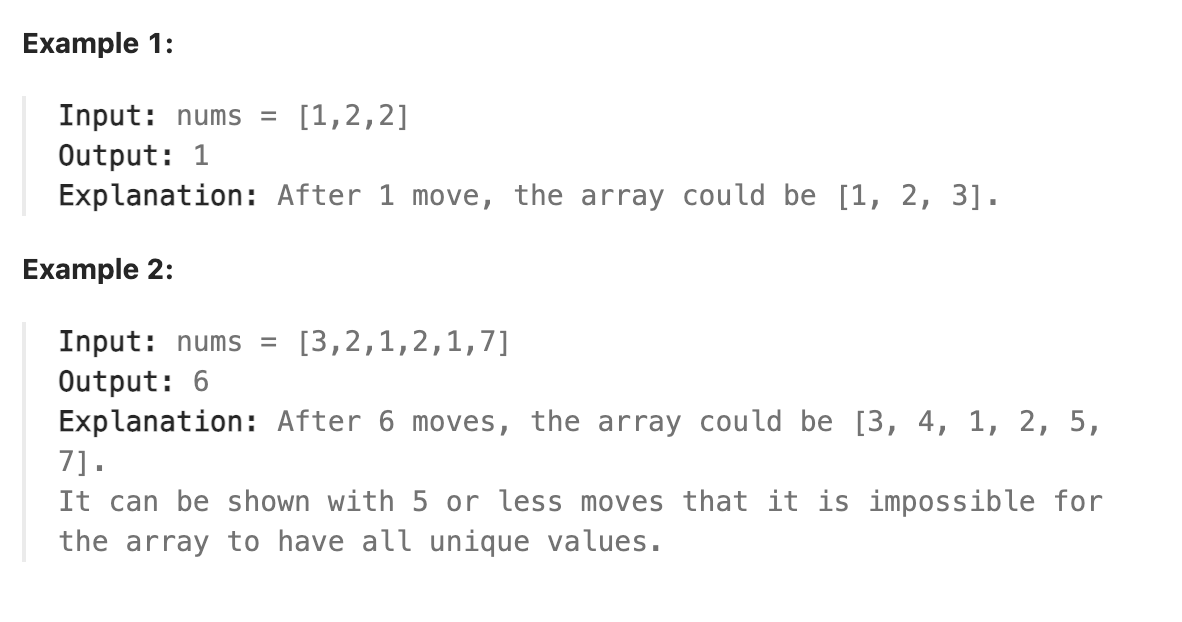
|
||||||
|
|
||||||
|
### 题解
|
||||||
|
|
||||||
|
本题思路上和昨天的题目基本一致, 先排序再从头遍历排好序的数组, 将重复的数字增加到最小不重复为止(如2,2,2,3, 将第二个2增加到3,将第三个2增加到4, 将3增加到5). 使用一个变量记录当前已经遍历过的数字修改后不重复数字中的最大值. 对遍历的每个数字, 判断其与当前最大值的关系, 若相等则将最大值加一并将结果加一, 小于最大值将最大值加一并将结果加上当前数字与最大值的差值, 大于最大值将最大值更新为当前数字.
|
||||||
|
考虑排序在这里的作用, 排序给每一个数字都提供了隐含的信息, 即前面的数字都比当前数字小, 后面的数字都比当前数字大, 这一信息使得我们可以做出更好的决策. 在未排序前, 对数组中的数我们无法知道是应该保持原样还是增加到某个数字可以满足题目条件, 但在排序后, 只需要增加到最小不重复的数即可, 考虑两个数字2,4, 假如当前不重复需要数字4,5(注意题目中只允许增加, 不允许减小). 无论是将2增加到4,4增加到5. 还是4不变,2增加到5, 增加的差值均为3. 这是因为任何比4大的值, 2都需要先增加到4, 再增加到这个值. 这一过程无论是在同一个数字上完成还是拆分为在两个数字上完成, 过程中需要改变的差值都是一样的.
|
||||||
|
|
||||||
|
### 代码
|
||||||
|
|
||||||
|
```go
|
||||||
|
func minIncrementForUnique(nums []int) int {
|
||||||
|
sort.Ints(nums)
|
||||||
|
current := -1
|
||||||
|
result := 0
|
||||||
|
for _,value := range nums{
|
||||||
|
if value == current{
|
||||||
|
value++
|
||||||
|
current++
|
||||||
|
result++
|
||||||
|
}else if value < current{
|
||||||
|
current++
|
||||||
|
result += current - value
|
||||||
|
}else {
|
||||||
|
current = value
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return result
|
||||||
|
}
|
||||||
|
```
|
||||||
|
Reference in New Issue
Block a user